PHP のポストで未定義のインデックス
- 未定義のインデックスエラーを再現する
-
isset()
を使用して、$_POST
の内容を確認する -
array_key_exists()
を使用して、$_POST
のキーを検索する -
in_array
を使用して、$_POST
に値が存在するかどうかを確認する -
ヌル合体演算子を使用する(
??
)
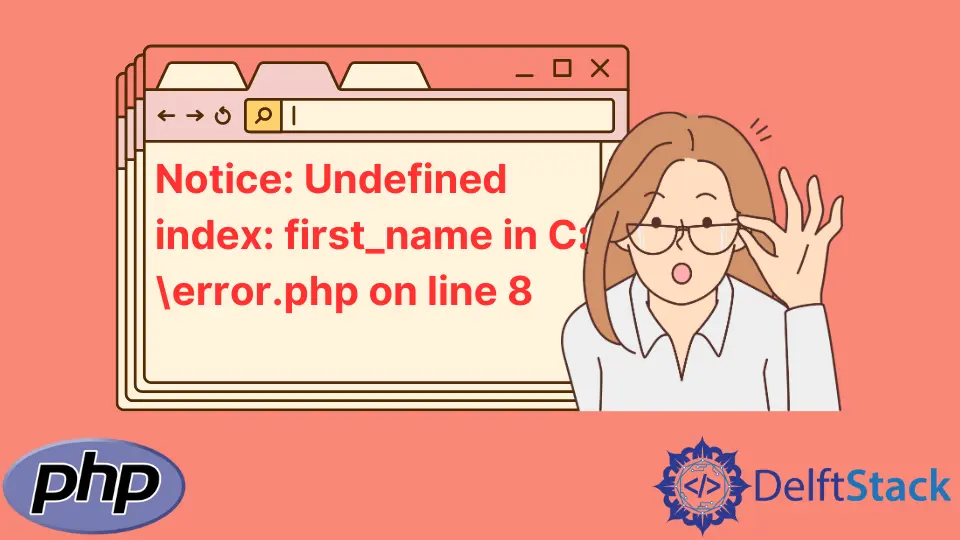
この記事では、PHP $_POST
の undefined index
に対する 4つのソリューションについて説明します。ソリューションは、isset()
、array_key_exists()
、in_array()
、および Null 合体演算子を使用します。
まず、解決策を説明する前にエラーを再現します。一方、PHP 8
では、undefined index
は undefined array key
と同じです。
未定義のインデックスエラーを再現する
エラーを再現するには、XAMPP
または WAMP
をダウンロードし、htdocs
に作業ディレクトリを作成します。次の HTML フォームを作業ディレクトリに sample-html-form.html
として保存します。
フォームには、ユーザーの名前と名前を受け入れる 2つの入力フィールドがあります。また、フォームの action 属性が 0-reproduce-the-error.php
に設定されていることにも注意してください。
コード-sample-html-form.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Sample HTML Form</title>
<style type="text/css">
body { height: 100vh; display: grid; place-items: center; }
main { display: grid; outline: 3px solid #1a1a1a; width: 50%; padding: 0.5em; }
form { align-self: center; justify-self: center; width: 50%;}
input:not([type="submit"]) { width: 50%; }
input[type="submit"] { padding: 0.5em;}
.form-row { display: flex; justify-content: space-between; margin-bottom: 1rem; }
.form-row:last-child { justify-content: center; }
</style>
</head>
<body>
<main>
<!--
This is a sample form that demonstrates how
to fix the undefined index in post when using
PHP. You can replace the value of the "action"
attribute with any of the PHP code in
this article.
- DelftStack.com
-->
<form method="post" action="0-reproduce-the-error.php">
<div class="form-row">
<label id="first_name">First name</label>
<input name="first_name" type="text" required>
</div>
<div class="form-row">
<label id="last_name">Last name</label>
<input type="text" name="last_name" required>
</div>
<div class="form-row">
<input type="submit" name="submit_bio_data" value="Submit">
</div>
</form>
</main>
</body>
</html>
コード-0-reproduce-the-error.php
:
<?php
// The purpose of this script is to reproduce
// the undefined index or undefined array
// key error messages. Also, it requires
// that the $_POST array does not contain
// the first_name and last_name indexes.
// DO NOT USE THIS CODE ON PRODUCTION SERVERS.
$first_name = $_POST['first_name'];
$last_name = $_POST['last_name'];
if ($first_name && $last_name) {
echo "Your first name is " . htmlspecialchars($first_name);
echo "Your last name is " . htmlspecialchars($last_name);
}
?>
次に、Web ブラウザで HTML フォームを開きます。次に、入力フィールドに空の文字列を入力し、送信ボタンを押します。
PHP 5
では、次の画像に示すような出力が得られます。この画像は、undefined index
が PHP 5
の Notice
であることを示しています。
出力:
PHP 8
を使用する場合、undefined index
は undefined array key
になります。また、PHP 5
とは異なり、undefined array key
は PHP 8
の Warning
です。
出力:
次のことは、それを解決するさまざまな方法を示すことです。
isset()
を使用して、$_POST
の内容を確認する
isset()
を使用すると、そのコンテンツを使用する前に $_POST
を確認できます。まず、条件チェックで isset()
を使用する if...else
ステートメントを設定します。
if
ブロックのコードは、isset()
が true
を返した場合にのみ実行する必要があります。これにより、undefined index
エラーを防ぐことができます。
以下のコードでは、HTML フォームの名前属性が $_POST
に存在するかどうかを確認しています。また、ユーザーが空の文字列を入力していないことを確認します。
チェックで true
が返された場合、ユーザーが入力したデータを出力します。PHP コードを 1-fix-with-isset.php
として作業ディレクトリに保存し、HTML フォームにリンクします。
コード-1-fix-with-isset.php
:
<?php
// It's customary to check that the user clicked
// the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// In the following "if" statement, we check for
// the following:
// 1. The existence of the first_name last_name
// in $_POST.
// 2. Prevent the user from supplying an empty
// string.
if (isset($_POST['first_name'], $_POST['last_name'])
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name'])))
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']);
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
// If the user sees the following message, that
// means either first_name or last_name is not
// in the $_POST array.
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
出力(ユーザーが空の値を指定した場合):
array_key_exists()
を使用して、$_POST
のキーを検索する
array_key_exists()
は、その名前が示すように、$_POST
でキーを検索します。この場合、これらのキーは HTML フォーム入力の名前属性です。
array_key_exists()
が $_POST
で名前属性を見つけた場合にのみ、フォームを処理します。安全のために、empty()
関数を使用して、ユーザーがデータを入力したことを確認します。
これらはすべて、次のコードに含まれています。コードをテストするには、コードを作業ディレクトリに 2-fix-with-array-key-exists.php
として保存し、action
属性の値を更新して HTML フォームにリンクします。
ここで、次の手順を実行します。
- コードエディタで HTML ファイルを開きます。
First name
入力のname
属性をfirst_nam
に更新します(最後のe
を削除しました)。- Web ブラウザーに切り替えて、名前と名前を入力します。
フォームに正しいデータを入力すると、エラーが発生します。これは、first_nam
と first_name
の間に不一致があるためです。
PHP スクリプトは後者を期待していましたが、前者を取得しました。エラーチェックを行わないと、undefined index
または undefined array key
が取得されます。
コード-2-fix-with-array-key-exists.php
:
<?php
// Ensure the user clicked the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// Use array_key_exists to check for the
// first_name and last_name fields. At the
// same time, prevent the user from supplying
// an empty string. You can also implement other
// validation checks depending on your use case.
if (array_key_exists('first_name', $_POST) && array_key_exists('last_name', $_POST)
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name']))
)
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
出力(DevTools
で強調表示されている部分に注意してください):
in_array
を使用して、$_POST
に値が存在するかどうかを確認する
PHP の in_array()
関数は、$_POST
で特定の値を検索できます。これを知っていると、ユーザーが HTML フォームに入力したかどうかを確認するために使用できます。
empty()
関数と一緒に、ユーザーが空の文字列を指定しなかったことを確認できます。検索と空のチェックの両方で、undefined index
または undefined array key
が防止されます。
以下に詳述する例では、in_array
と empty()
を使用して、undefined index
エラーを防ぎます。PHP コードを 3-fix-with-in-array.php
として保存し、HTML の action
属性に PHP ファイルの名前を設定します。
HTML フォームを開き、Last name
フィールドに入力し、First name
を空白のままにします。フォームを送信すると、in_array
が $_POST
で名を見つけられなかったため、カスタムエラーメッセージが表示されます。
コード-3-fix-with-in-array.php
:
<?php
// Ensure the user clicked the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// Use in_array to check for the first and last
// name fields. Also, check for empty submissions
// by the user. We advise that you implement other
// checks to suit your use case.
if (in_array('first_name', $_POST) && in_array('last_name', $_POST)
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name']))
)
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
出力:
ヌル合体演算子を使用する(??
)
null 合体演算子(??
)は、オペランドが存在する場合、左側にオペランドを返します。それ以外の場合は、右側のオペランドを返します。
この知識があれば、入力値を Null 合体演算子に渡すことができます。その結果、値が存在する場合、演算子はその値を返します。それ以外の場合は、別の値を返すようにします。
以下のコードでは、Null 合体演算子がこの他の値を返したかどうかを確認します。含まれている場合は、フォーム入力の 1つが $_POST
にないことを意味します。
これにより、undefined index
または undefined array key
以外のカスタムエラーメッセージを表示できます。コードを 4-fix-with-null-coalescing-operator.php
として保存し、HTML フォームにリンクします。
次に、Web ブラウザーを開き、フォームに正しいデータを入力します。フォームを送信してもエラーメッセージは表示されません。
コード-4-fix-with-null-coalescing-operator.php
:
<?php
// It's customary to check that the user clicked
// the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// This works in PHP 7 and above. It's called
// the null coalescing operator. It'll return
// the value on the left if it exists or the
// value on the right if it does not. While
// By doing this, we ensure the user does not
// enter empty strings. What's more, you can
// implement other checks for your use case.
$first_name = !empty(trim($_POST['first_name'])) ?? 'Missing';
$last_name = !empty(trim($_POST['last_name'])) ?? 'Missing';
// if the null coalescing operator returns
// the string "Missing" for first_name
// or last_name, that means they are not valid
// indexes of the $_POST array.
if ($first_name && $last_name !== 'Missing') {
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
// If the user sees the following message, that
// means either first_name or last_name is not
// in the $_POST array.
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
出力:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn