Índice indefinido con PHP Post
- Reproducir el error de índice indefinido
-
Utilice
isset()
para comprobar el contenido de$_POST
-
Utilice
array_key_exists()
para buscar las claves en$_POST
-
Utilice
in_array
para comprobar si existe un valor en$_POST
-
Usar operador coalescente nulo (
??
)
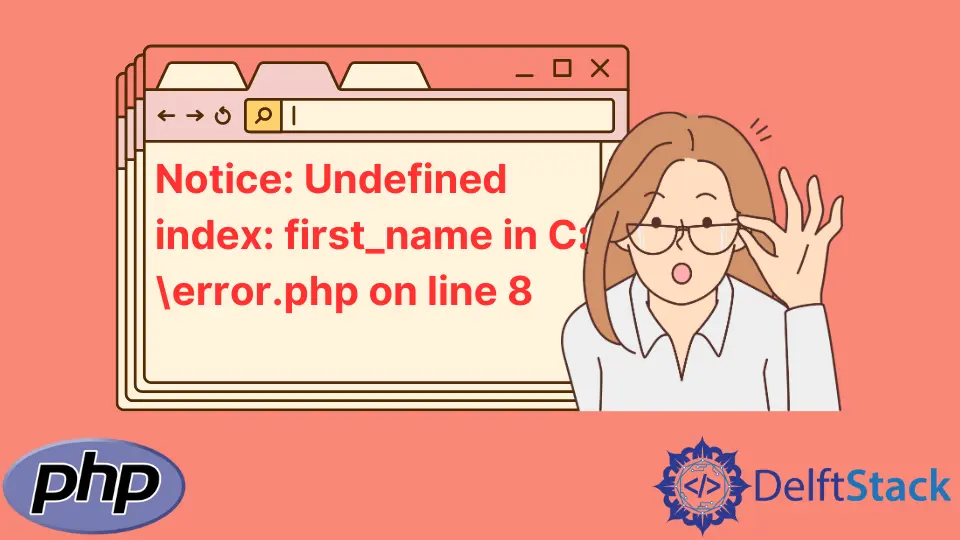
Este artículo enseña cuatro soluciones para undefined index
en PHP $_POST
. Las soluciones utilizarán isset()
, array_key_exists()
, in_array()
, y el operador de fusión nulo.
Primero, reproduciremos el error antes de explicar las soluciones. Mientras tanto, en PHP 8
, undefined index
es lo mismo que undefined array key
.
Reproducir el error de índice indefinido
Para reproducir el error, descarga XAMPP
o WAMP
y crea un directorio de trabajo en htdocs
. Guarde el siguiente formulario HTML en su directorio de trabajo como sample-html-form.html
.
El formulario tiene dos campos de entrada que aceptan el nombre y apellido de un usuario. También notará que hemos establecido el atributo de acción del formulario en 0-reproduce-the-error.php
.
Código - sample-html-form.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Sample HTML Form</title>
<style type="text/css">
body { height: 100vh; display: grid; place-items: center; }
main { display: grid; outline: 3px solid #1a1a1a; width: 50%; padding: 0.5em; }
form { align-self: center; justify-self: center; width: 50%;}
input:not([type="submit"]) { width: 50%; }
input[type="submit"] { padding: 0.5em;}
.form-row { display: flex; justify-content: space-between; margin-bottom: 1rem; }
.form-row:last-child { justify-content: center; }
</style>
</head>
<body>
<main>
<!--
This is a sample form that demonstrates how
to fix the undefined index in post when using
PHP. You can replace the value of the "action"
attribute with any of the PHP code in
this article.
- DelftStack.com
-->
<form method="post" action="0-reproduce-the-error.php">
<div class="form-row">
<label id="first_name">First name</label>
<input name="first_name" type="text" required>
</div>
<div class="form-row">
<label id="last_name">Last name</label>
<input type="text" name="last_name" required>
</div>
<div class="form-row">
<input type="submit" name="submit_bio_data" value="Submit">
</div>
</form>
</main>
</body>
</html>
Código - 0-reproduce-el-error.php
:
<?php
// The purpose of this script is to reproduce
// the undefined index or undefined array
// key error messages. Also, it requires
// that the $_POST array does not contain
// the first_name and last_name indexes.
// DO NOT USE THIS CODE ON PRODUCTION SERVERS.
$first_name = $_POST['first_name'];
$last_name = $_POST['last_name'];
if ($first_name && $last_name) {
echo "Your first name is " . htmlspecialchars($first_name);
echo "Your last name is " . htmlspecialchars($last_name);
}
?>
Ahora, abra el formulario HTML en su navegador web. Luego escriba cadenas vacías en los campos de entrada y presione el botón Enviar.
En PHP 5
, obtendrás una salida como la que se muestra en la siguiente imagen. La imagen muestra que undefined index
es un Notice
en PHP 5
.
Producción:
Si usa PHP 8
, undefined index
se convierte en undefined array key
. Además, a diferencia de PHP 5
, undefined array key
es un Warning
en PHP 8
.
Producción:
Lo siguiente es mostrarte diferentes formas que lo resolverán.
Utilice isset()
para comprobar el contenido de $_POST
Con isset()
, puedes comprobar $_POST
antes de usar cualquiera de sus contenidos. Primero, configure una declaración if...else
que use isset()
en su verificación condicional.
El código en el bloque if
solo debe ejecutarse si isset()
devuelve true
. Al hacer esto, evitará el error de undefined index
.
En el siguiente código, estamos comprobando si los atributos de nombre del formulario HTML existen en $_POST
. Además, nos aseguramos de que el usuario no haya ingresado cadenas vacías.
Si la comprobación devuelve true
, imprimimos los datos proporcionados por el usuario. Guarde el código PHP como 1-fix-with-isset.php
en su directorio de trabajo y vincúlelo con el formulario HTML.
Código - 1-fix-with-isset.php
:
<?php
// It's customary to check that the user clicked
// the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// In the following "if" statement, we check for
// the following:
// 1. The existence of the first_name last_name
// in $_POST.
// 2. Prevent the user from supplying an empty
// string.
if (isset($_POST['first_name'], $_POST['last_name'])
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name'])))
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']);
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
// If the user sees the following message, that
// means either first_name or last_name is not
// in the $_POST array.
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
Salida (cuando el usuario proporciona valores vacíos):
Utilice array_key_exists()
para buscar las claves en $_POST
El array_key_exists()
como su nombre lo indica buscará una clave en $_POST
. En nuestro caso, estas claves son los atributos de nombre de las entradas del formulario HTML.
Solo procesaremos el formulario si array_key_exists()
encuentra los atributos del nombre en $_POST
. Para estar seguros, usamos la función empty()
para asegurarnos de que el usuario ingrese algunos datos.
Encontrará todo esto en el siguiente código. Para probar el código, guárdelo como 2-fix-with-array-key-exists.php
en su directorio de trabajo y vincúlelo con su formulario HTML actualizando el valor del atributo action
.
Ahora, haz los siguientes pasos.
- Abra el archivo HTML en su editor de código.
- Actualice el atributo
name
de la entradaFirst name
afirst_nam
(eliminamos la últimae
). - Cambie a su navegador web y complete el nombre y apellido.
Se producirá un error cuando rellene el formulario con los datos correctos. Eso es porque hay una falta de coincidencia entre first_nam
y first_name
.
El script PHP esperaba lo último, pero obtuvo lo primero. Sin la comprobación de errores, obtendrá el undefined index
o undefined array key
.
Código - 2-fix-with-array-key-exists.php
:
<?php
// Ensure the user clicked the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// Use array_key_exists to check for the
// first_name and last_name fields. At the
// same time, prevent the user from supplying
// an empty string. You can also implement other
// validation checks depending on your use case.
if (array_key_exists('first_name', $_POST) && array_key_exists('last_name', $_POST)
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name']))
)
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
Salida (preste atención a la parte resaltada en DevTools
):
Utilice in_array
para comprobar si existe un valor en $_POST
La función PHP in_array()
puede buscar $_POST
para un valor específico. Sabiendo esto, podemos usarlo para confirmar si el usuario llenó el formulario HTML.
Junto con la función empty()
, podemos asegurarnos de que el usuario no proporcionó cadenas vacías. Tanto la búsqueda como la comprobación de vacíos evitan que se produzcan undefined index
o undefined array key
.
En el ejemplo que se detalla a continuación, usamos in_array
y empty()
para evitar el error de undefined index
. Guarde el código PHP como 3-fix-with-in-array.php
, luego configure el atributo action
del HTML con el nombre del archivo PHP.
Abra el formulario HTML, complete el campo Last name
y deje el First name
en blanco. Envíe el formulario y obtendrá el mensaje de error personalizado porque in_array
no encontró el primer nombre en $_POST
.
Código - 3-fix-with-in-array.php
:
<?php
// Ensure the user clicked the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// Use in_array to check for the first and last
// name fields. Also, check for empty submissions
// by the user. We advise that you implement other
// checks to suit your use case.
if (in_array('first_name', $_POST) && in_array('last_name', $_POST)
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name']))
)
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
Producción:
Usar operador coalescente nulo (??
)
El operador coalescente nulo (??
) devolverá el operando en su lado izquierdo si existe. De lo contrario, devolverá el operando de la derecha.
Armados con este conocimiento, podemos pasar un valor de entrada al operador coalescente nulo. Como resultado, si el valor existe, el operador lo devolverá; de lo contrario, haremos que devuelva otro valor.
En el código a continuación, verificaremos si el operador fusionado nulo devolvió este otro valor. Si es así, eso significa que una de las entradas del formulario no está en $_POST
.
Con esto, podemos mostrar un mensaje de error personalizado que no sea undefined index
o undefined array key
. Guarde el código como 4-fix-with-null-coalescing-operator.php
y vincúlelo con su formulario HTML.
Luego, abra su navegador web y complete el formulario con los datos correctos. No recibirá mensajes de error cuando envíe el formulario.
Código - 4-fix-with-null-coalescing-operator.php
:
<?php
// It's customary to check that the user clicked
// the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// This works in PHP 7 and above. It's called
// the null coalescing operator. It'll return
// the value on the left if it exists or the
// value on the right if it does not. While
// By doing this, we ensure the user does not
// enter empty strings. What's more, you can
// implement other checks for your use case.
$first_name = !empty(trim($_POST['first_name'])) ?? 'Missing';
$last_name = !empty(trim($_POST['last_name'])) ?? 'Missing';
// if the null coalescing operator returns
// the string "Missing" for first_name
// or last_name, that means they are not valid
// indexes of the $_POST array.
if ($first_name && $last_name !== 'Missing') {
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
// If the user sees the following message, that
// means either first_name or last_name is not
// in the $_POST array.
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
Producción:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn