PHP Post 的未定義索引
- 重現未定義的索引錯誤
-
使用
isset()
檢查$_POST
的內容 -
使用
array_key_exists()
搜尋$_POST
中的鍵 -
使用
in_array
檢查值是否存在於$_POST
-
使用空合併運算子 (
??
)
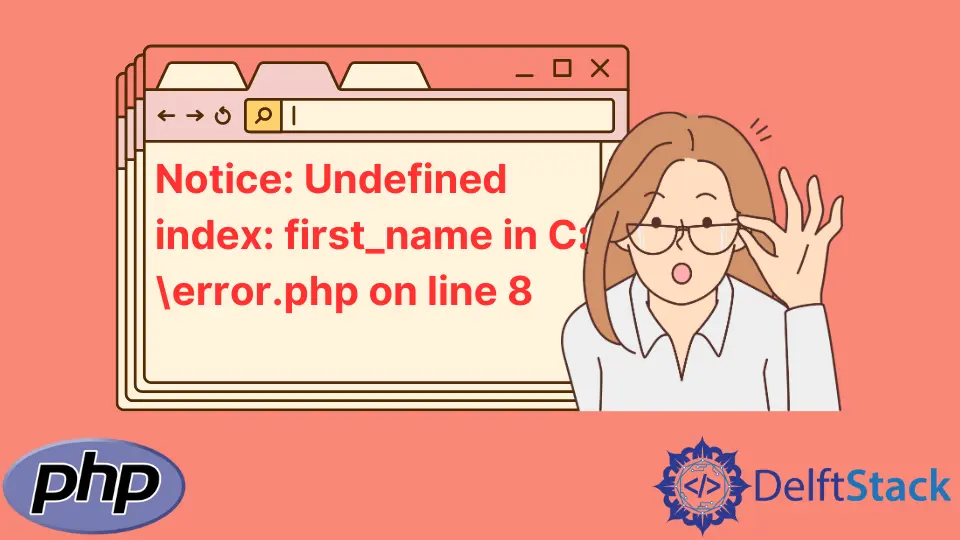
本文介紹了 PHP $_POST
中未定義索引
的四種解決方案。解決方案將使用 isset()
、array_key_exists()
、in_array()
和 Null 合併運算子。
首先,我們將在解釋解決方案之前重現錯誤。同時,在 PHP 8
中,未定義索引
與未定義陣列鍵
相同。
重現未定義的索引錯誤
要重現錯誤,請下載 XAMPP
或 WAMP
並在 htdocs
中建立一個工作目錄。在你的工作目錄中將以下 HTML 表單儲存為 sample-html-form.html
。
該表單有兩個輸入欄位,它們接受使用者的名字和姓氏。你還會注意到我們已將表單的 action 屬性設定為 0-reproduce-the-error.php
。
程式碼 - sample-html-form.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Sample HTML Form</title>
<style type="text/css">
body { height: 100vh; display: grid; place-items: center; }
main { display: grid; outline: 3px solid #1a1a1a; width: 50%; padding: 0.5em; }
form { align-self: center; justify-self: center; width: 50%;}
input:not([type="submit"]) { width: 50%; }
input[type="submit"] { padding: 0.5em;}
.form-row { display: flex; justify-content: space-between; margin-bottom: 1rem; }
.form-row:last-child { justify-content: center; }
</style>
</head>
<body>
<main>
<!--
This is a sample form that demonstrates how
to fix the undefined index in post when using
PHP. You can replace the value of the "action"
attribute with any of the PHP code in
this article.
- DelftStack.com
-->
<form method="post" action="0-reproduce-the-error.php">
<div class="form-row">
<label id="first_name">First name</label>
<input name="first_name" type="text" required>
</div>
<div class="form-row">
<label id="last_name">Last name</label>
<input type="text" name="last_name" required>
</div>
<div class="form-row">
<input type="submit" name="submit_bio_data" value="Submit">
</div>
</form>
</main>
</body>
</html>
程式碼 - 0-reproduce-the-error.php
:
<?php
// The purpose of this script is to reproduce
// the undefined index or undefined array
// key error messages. Also, it requires
// that the $_POST array does not contain
// the first_name and last_name indexes.
// DO NOT USE THIS CODE ON PRODUCTION SERVERS.
$first_name = $_POST['first_name'];
$last_name = $_POST['last_name'];
if ($first_name && $last_name) {
echo "Your first name is " . htmlspecialchars($first_name);
echo "Your last name is " . htmlspecialchars($last_name);
}
?>
現在,在 Web 瀏覽器中開啟 HTML 表單。然後在輸入欄位中輸入空字串並點選提交按鈕。
在 PHP 5
中,你將獲得如下圖所示的輸出。圖片顯示 undefined index
是 PHP 5
中的 Notice
。
輸出:
如果你使用 PHP 8
,未定義索引
變成未定義陣列鍵
。此外,與 PHP 5
不同,undefined array key
是 PHP 8
中的 Warning
。
輸出:
接下來是向你展示解決問題的不同方法。
使用 isset()
檢查 $_POST
的內容
使用 isset()
,你可以在使用其任何內容之前檢查 $_POST
。首先,設定一個 if...else
語句,在其條件檢查中使用 isset()
。
if
塊中的程式碼應該只在 isset()
返回 true
時執行。通過這樣做,你將防止出現未定義索引
錯誤。
在下面的程式碼中,我們正在檢查 HTML 表單的名稱屬性是否存在於 $_POST
中。此外,我們確保使用者沒有輸入空字串。
如果檢查返回 true
,我們列印使用者提供的資料。在你的工作目錄中將 PHP 程式碼儲存為 1-fix-with-isset.php
並將其與 HTML 表單連結。
程式碼 - 1-fix-with-isset.php
:
<?php
// It's customary to check that the user clicked
// the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// In the following "if" statement, we check for
// the following:
// 1. The existence of the first_name last_name
// in $_POST.
// 2. Prevent the user from supplying an empty
// string.
if (isset($_POST['first_name'], $_POST['last_name'])
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name'])))
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']);
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
// If the user sees the following message, that
// means either first_name or last_name is not
// in the $_POST array.
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
輸出(當使用者提供空值時):
使用 array_key_exists()
搜尋 $_POST
中的鍵
顧名思義,array_key_exists()
將在 $_POST
中搜尋一個鍵。在我們的例子中,這些鍵是 HTML 表單輸入的名稱屬性。
如果 array_key_exists()
在 $_POST
中找到名稱屬性,我們將只處理該表單。為了安全起見,我們使用 empty()
函式來確保使用者輸入了一些資料。
你將在以下程式碼中找到所有這些。要測試程式碼,請將其儲存為工作目錄中的 2-fix-with-array-key-exists.php
,並通過更新 action
屬性的值將其與 HTML 表單連結。
現在,執行以下步驟。
- 在程式碼編輯器中開啟 HTML 檔案。
- 將
First name
輸入的name
屬性更新為first_nam
(我們刪除了最後的e
)。 - 切換到你的 Web 瀏覽器並填寫名字和姓氏。
當你用正確的資料填寫表格時會發生錯誤。那是因為 first_nam
和 first_name
之間存在不匹配。
PHP 指令碼期望後者,但它得到了前者。如果沒有錯誤檢查,你將獲得未定義索引
或未定義陣列鍵
。
程式碼 - 2-fix-with-array-key-exists.php
:
<?php
// Ensure the user clicked the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// Use array_key_exists to check for the
// first_name and last_name fields. At the
// same time, prevent the user from supplying
// an empty string. You can also implement other
// validation checks depending on your use case.
if (array_key_exists('first_name', $_POST) && array_key_exists('last_name', $_POST)
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name']))
)
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
輸出(注意 DevTools
中突出顯示的部分):
使用 in_array
檢查值是否存在於 $_POST
PHP in_array()
函式可以搜尋 $_POST
以獲得特定值。知道了這一點,我們可以用它來確認使用者是否填寫了 HTML 表單。
與 empty()
函式一起,我們可以確保使用者沒有提供空字串。搜尋和空位檢查都可以防止未定義索引
或未定義陣列鍵
。
在下面詳述的示例中,我們使用 in_array
和 empty()
來防止 undefined index
錯誤。將 PHP 程式碼儲存為 3-fix-with-in-array.php
,然後將 HTML 的 action
屬性設定為 PHP 檔案的名稱。
開啟 HTML 表單,填寫 Last name
欄位,並將 First name
留空。提交表單,你將收到自定義錯誤訊息,因為 in_array
未在 $_POST
中找到名字。
程式碼 - 3-fix-with-in-array.php
:
<?php
// Ensure the user clicked the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// Use in_array to check for the first and last
// name fields. Also, check for empty submissions
// by the user. We advise that you implement other
// checks to suit your use case.
if (in_array('first_name', $_POST) && in_array('last_name', $_POST)
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name']))
)
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
輸出:
使用空合併運算子 (??
)
空合併運算子 (??
) 將返回其左側的運算元(如果存在)。否則,它將返回右側的運算元。
有了這些知識,我們可以將輸入值傳遞給 Null 合併運算子。結果,如果該值存在,則運算子將其返回; 否則,我們會讓它返回另一個值。
在下面的程式碼中,我們將檢查 Null 合併運算子是否返回了這個其他值。如果是,則表示其中一個表單輸入不在 $_POST
中。
有了這個,我們可以顯示除未定義索引
或未定義陣列鍵
之外的自定義錯誤訊息。將程式碼儲存為 4-fix-with-null-coalescing-operator.php
並將其與你的 HTML 表單連結。
然後,開啟你的網路瀏覽器並使用正確的資料填寫表格。提交表單時不會收到任何錯誤訊息。
程式碼 - 4-fix-with-null-coalescing-operator.php
:
<?php
// It's customary to check that the user clicked
// the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// This works in PHP 7 and above. It's called
// the null coalescing operator. It'll return
// the value on the left if it exists or the
// value on the right if it does not. While
// By doing this, we ensure the user does not
// enter empty strings. What's more, you can
// implement other checks for your use case.
$first_name = !empty(trim($_POST['first_name'])) ?? 'Missing';
$last_name = !empty(trim($_POST['last_name'])) ?? 'Missing';
// if the null coalescing operator returns
// the string "Missing" for first_name
// or last_name, that means they are not valid
// indexes of the $_POST array.
if ($first_name && $last_name !== 'Missing') {
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
// If the user sees the following message, that
// means either first_name or last_name is not
// in the $_POST array.
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
輸出:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn