PHP 포스트가 있는 정의되지 않은 인덱스
- 정의되지 않은 인덱스 오류 재현
-
isset()
을 사용하여$_POST
의 내용 확인 -
array_key_exists()
를 사용하여$_POST
에서 키 검색 -
in_array
를 사용하여$_POST
에 값이 있는지 확인 -
Null 병합 연산자 사용(
??
)
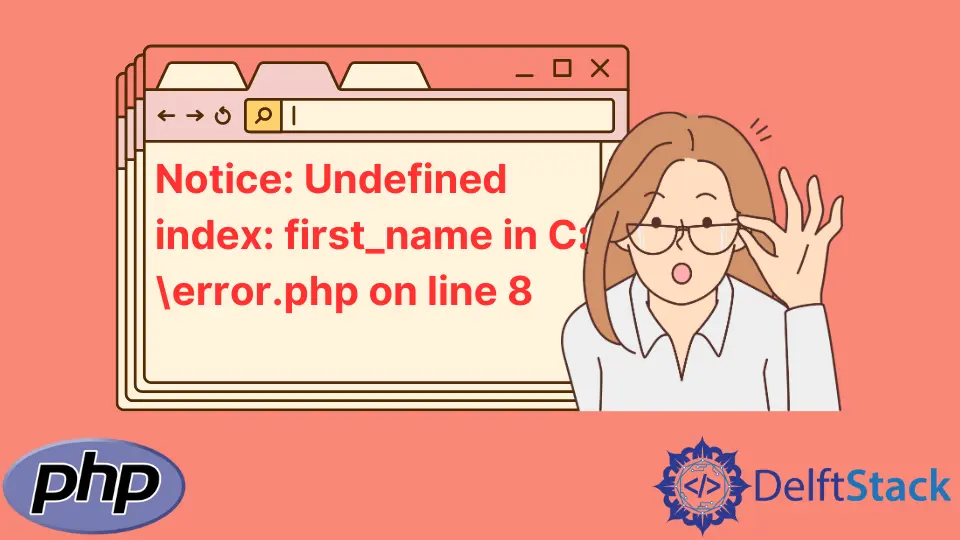
이 기사는 PHP $_POST
에서 undefined index
에 대한 네 가지 솔루션을 설명합니다. 솔루션은 isset()
, array_key_exists()
, in_array()
및 Null 병합 연산자를 사용합니다.
먼저 솔루션을 설명하기 전에 오류를 재현합니다. 한편, PHP 8
에서 undefined index
는 undefined array key
와 동일합니다.
정의되지 않은 인덱스 오류 재현
오류를 재현하려면 XAMPP
또는 WAMP
를 다운로드하고 htdocs
에 작업 디렉토리를 만드십시오. 작업 디렉토리에 다음 HTML 양식을 sample-html-form.html
로 저장합니다.
양식에는 사용자의 이름과 성을 허용하는 두 개의 입력 필드가 있습니다. 또한 양식의 action 속성을 0-reproduce-the-error.php
로 설정했음을 알 수 있습니다.
코드 - sample-html-form.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Sample HTML Form</title>
<style type="text/css">
body { height: 100vh; display: grid; place-items: center; }
main { display: grid; outline: 3px solid #1a1a1a; width: 50%; padding: 0.5em; }
form { align-self: center; justify-self: center; width: 50%;}
input:not([type="submit"]) { width: 50%; }
input[type="submit"] { padding: 0.5em;}
.form-row { display: flex; justify-content: space-between; margin-bottom: 1rem; }
.form-row:last-child { justify-content: center; }
</style>
</head>
<body>
<main>
<!--
This is a sample form that demonstrates how
to fix the undefined index in post when using
PHP. You can replace the value of the "action"
attribute with any of the PHP code in
this article.
- DelftStack.com
-->
<form method="post" action="0-reproduce-the-error.php">
<div class="form-row">
<label id="first_name">First name</label>
<input name="first_name" type="text" required>
</div>
<div class="form-row">
<label id="last_name">Last name</label>
<input type="text" name="last_name" required>
</div>
<div class="form-row">
<input type="submit" name="submit_bio_data" value="Submit">
</div>
</form>
</main>
</body>
</html>
코드 - 0-reproduc-the-error.php
:
<?php
// The purpose of this script is to reproduce
// the undefined index or undefined array
// key error messages. Also, it requires
// that the $_POST array does not contain
// the first_name and last_name indexes.
// DO NOT USE THIS CODE ON PRODUCTION SERVERS.
$first_name = $_POST['first_name'];
$last_name = $_POST['last_name'];
if ($first_name && $last_name) {
echo "Your first name is " . htmlspecialchars($first_name);
echo "Your last name is " . htmlspecialchars($last_name);
}
?>
이제 웹 브라우저에서 HTML 양식을 엽니다. 그런 다음 입력 필드에 빈 문자열을 입력하고 제출 버튼을 누르십시오.
PHP 5
에서는 다음 이미지와 같은 출력을 얻을 수 있습니다. 이미지는 undefined index
가 PHP 5
의 Notice
임을 보여줍니다.
출력:
PHP 8
을 사용하면 undefined index
가 undefined array key
가 됩니다. 또한 PHP 5
와 달리 undefined array key
는 PHP 8
의 경고
입니다.
출력:
다음은 그것을 해결할 다양한 방법을 보여주는 것입니다.
isset()
을 사용하여 $_POST
의 내용 확인
isset()
을 사용하면 컨텐츠를 사용하기 전에 $_POST
를 확인할 수 있습니다. 먼저 조건부 검사에서 isset()
을 사용하는 if...else
문을 설정합니다.
if
블록의 코드는 isset()
이 true
를 반환하는 경우에만 실행되어야 합니다. 이렇게 하면 undefined index
오류를 방지할 수 있습니다.
아래 코드에서는 HTML 형식의 이름 속성이 $_POST
에 존재하는지 확인하고 있습니다. 또한 사용자가 빈 문자열을 입력하지 않았는지 확인합니다.
검사 결과 true
가 반환되면 사용자가 제공한 데이터를 인쇄합니다. PHP 코드를 작업 디렉토리에 1-fix-with-isset.php
로 저장하고 HTML 형식과 연결합니다.
코드 - 1-fix-with-isset.php
:
<?php
// It's customary to check that the user clicked
// the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// In the following "if" statement, we check for
// the following:
// 1. The existence of the first_name last_name
// in $_POST.
// 2. Prevent the user from supplying an empty
// string.
if (isset($_POST['first_name'], $_POST['last_name'])
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name'])))
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']);
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
// If the user sees the following message, that
// means either first_name or last_name is not
// in the $_POST array.
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
출력(사용자가 빈 값을 제공할 때):
array_key_exists()
를 사용하여 $_POST
에서 키 검색
array_key_exists()
는 이름에서 알 수 있듯이 $_POST
에서 키를 검색합니다. 우리의 경우 이러한 키는 HTML 양식 입력의 이름 속성입니다.
array_key_exists()
가 $_POST
에서 이름 속성을 찾는 경우에만 양식을 처리합니다. 안전을 위해 empty()
함수를 사용하여 사용자가 일부 데이터를 입력했는지 확인합니다.
다음 코드에서 이 모든 것을 찾을 수 있습니다. 코드를 테스트하려면 작업 디렉토리에 2-fix-with-array-key-exists.php
로 저장하고 action
속성 값을 업데이트하여 HTML 양식과 연결하십시오.
이제 다음 단계를 수행하십시오.
- 코드 편집기에서 HTML 파일을 엽니다.
First name
입력의name
속성을first_name
으로 업데이트합니다(마지막 “e"는 삭제했습니다).- 웹 브라우저로 전환하고 성과 이름을 입력합니다.
양식을 올바른 데이터로 채우면 오류가 발생합니다. first_name
과 first_name
사이에 불일치가 있기 때문입니다.
PHP 스크립트는 후자를 예상했지만 전자를 얻었습니다. 오류 검사가 없으면 undefined index
또는 undefined array key
가 표시됩니다.
코드 - 2-fix-with-array-key-exists.php
:
<?php
// Ensure the user clicked the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// Use array_key_exists to check for the
// first_name and last_name fields. At the
// same time, prevent the user from supplying
// an empty string. You can also implement other
// validation checks depending on your use case.
if (array_key_exists('first_name', $_POST) && array_key_exists('last_name', $_POST)
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name']))
)
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
출력(DevTools
에서 강조 표시된 부분에 주의):
in_array
를 사용하여 $_POST
에 값이 있는지 확인
PHP in_array()
함수는 $_POST
에서 특정 값을 검색할 수 있습니다. 이것을 알면 사용자가 HTML 양식을 채웠는지 확인하는 데 사용할 수 있습니다.
empty()
함수와 함께 사용자가 빈 문자열을 제공하지 않았는지 확인할 수 있습니다. 검색 및 공백 검사 모두 정의되지 않은 인덱스
또는 정의되지 않은 배열 키
를 방지합니다.
아래에 자세히 설명된 예에서는 in_array
및 empty()
를 사용하여 undefined index
오류를 방지합니다. PHP 코드를 3-fix-with-in-array.php
로 저장한 다음 HTML의 action
속성을 PHP 파일 이름으로 설정합니다.
HTML 양식을 열고 Last name
필드를 채우고 First name
은 공백으로 둡니다. 양식을 제출하면 in_array
가 $_POST
에서 이름을 찾지 못했기 때문에 사용자 정의 오류 메시지를 받게 됩니다.
코드 - 3-fix-with-in-array.php
:
<?php
// Ensure the user clicked the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// Use in_array to check for the first and last
// name fields. Also, check for empty submissions
// by the user. We advise that you implement other
// checks to suit your use case.
if (in_array('first_name', $_POST) && in_array('last_name', $_POST)
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name']))
)
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
출력:
Null 병합 연산자 사용(??
)
null 병합 연산자(??
)는 피연산자가 있는 경우 왼쪽에 피연산자를 반환합니다. 그렇지 않으면 오른쪽에 있는 피연산자를 반환합니다.
이 지식으로 무장하면 Null 병합 연산자에 입력 값을 전달할 수 있습니다. 결과적으로 값이 있으면 연산자가 값을 반환합니다. 그렇지 않으면 다른 값을 반환하도록 합니다.
아래 코드에서 Null 병합 연산자가 이 다른 값을 반환했는지 확인합니다. 그렇다면 양식 입력 중 하나가 $_POST
에 없음을 의미합니다.
이를 통해 undefined index
또는 undefined array key
이외의 사용자 지정 오류 메시지를 표시할 수 있습니다. 코드를 4-fix-with-null-coalescing-operator.php
로 저장하고 HTML 양식과 연결합니다.
그런 다음 웹 브라우저를 열고 올바른 데이터로 양식을 작성하십시오. 양식을 제출할 때 오류 메시지가 표시되지 않습니다.
코드 - 4-fix-with-null-coalescing-operator.php
:
<?php
// It's customary to check that the user clicked
// the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// This works in PHP 7 and above. It's called
// the null coalescing operator. It'll return
// the value on the left if it exists or the
// value on the right if it does not. While
// By doing this, we ensure the user does not
// enter empty strings. What's more, you can
// implement other checks for your use case.
$first_name = !empty(trim($_POST['first_name'])) ?? 'Missing';
$last_name = !empty(trim($_POST['last_name'])) ?? 'Missing';
// if the null coalescing operator returns
// the string "Missing" for first_name
// or last_name, that means they are not valid
// indexes of the $_POST array.
if ($first_name && $last_name !== 'Missing') {
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
// If the user sees the following message, that
// means either first_name or last_name is not
// in the $_POST array.
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
출력:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn