How to Find Root Directory Path in PHP
-
Use the
__DIR__
Predefined Constant to Find the Path of the Directory of a File in PHP -
Use the
dirname()
Function to Find the Path of the Root Directory of a Project in PHP -
Use
$_SERVER['DOCUMENT_ROOT']
to Find the Document Root Directory of a File in PHP
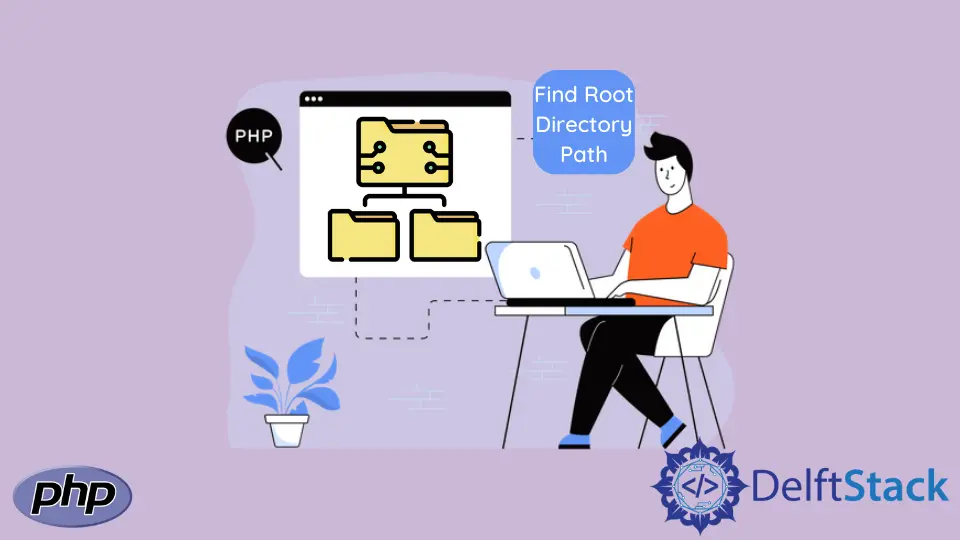
We will introduce different methods to find the path of the root directory of a PHP project.
Use the __DIR__
Predefined Constant to Find the Path of the Directory of a File in PHP
In PHP, there are predefined constants that can be used to achieve various functionalities. __DIR__
is one magical constant that returns the complete file path of the current file from the root directory. It means it will return the file’s directory. dirname(__FILE__)
can also be used for the same purpose.
Suppose we have a project
folder which is the root directory of the project. The project
folder has the following file path /var/www/HTML/project
. Inside the project
folder, we have the index.php
file and another folder master
. Inside the master
folder, we have two PHP files: login.php
and register.php
.
project
├── index.php
└── master
├── login.php
└── register.php
Suppose we are currently working on login.php
. In such a file structure, we can get the directory’s path using the __DIR__
constant in the login.php
file. We can use the echo
function to print the constant.
Example Code:
<?php
echo __DIR__;
?>
Output:
/var/www/html/project/master
Use the dirname()
Function to Find the Path of the Root Directory of a Project in PHP
The function dirname(__FILE__)
is similar to __DIR__
. We can find the path of the directory of a file using this function. We can also move to the upper levels in the file path using the dirname()
function. The first parameter of the function is the path of the file, which is denoted by the __FILE__
constant. The second parameter is an integer which is called levels. We can set the levels to direct the function to level up in the file path. The default value of the level is 1
. As we increase the level, the function will get the file path of one level up. So, we can use this function to find the exact file path of the project’s root directory in PHP.
For example, we can consider the file structure as the first method. Working from the file, login.php
, we can use the dirname()
function with level 2
and the __FILE__
constant as parameters. Then we can get the exact file path of the working directory. Thus, we can change the levels according to our choice to move upward and downward in the file path. In this way, we can find the path of the root directory of the project in PHP.
Example Code:
<?php
echo dirname(__FILE__,2);
?>
Output:
/var/www/html/project
Use $_SERVER['DOCUMENT_ROOT']
to Find the Document Root Directory of a File in PHP
We can use the $_SERVER[]
array with the DOCUMENT_ROOT
indices to find the document root directory of the currently executing script. It will return the complete path of the document root directory. It is defined in the configuration file in the server. For the file structure above, we can print the $_SERVER['DOCUMENT_ROOT']
with the echo
function to find the document root directory of the file login.php
.
As shown in the output below, we found out the path html
is the document root directory of the login.php
file. We can see the file path of the root directory as well.
Example Code:
<?php
echo $_SERVER['DOCUMENT_ROOT'];
?>
Output:
/var/www/html
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn