How to Work With DatePicker in PHP
-
Use
strtotime()
andDateTime()
to Create Date Within PHP -
Use Essential JS for PHP for
DatePicker
Capabilities
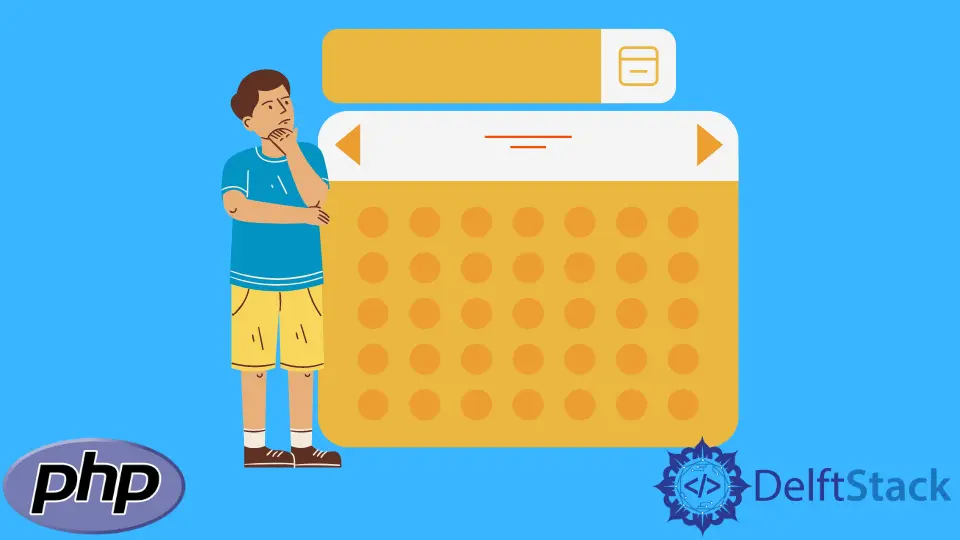
When we fill out forms within any site, we might need to use a DatePicker to select a date. For example, date of birth or date of course completion.
To do so, you can use HTML and JavaScript. However, to use the date, we need to compare the date the user picks on the server-side.
We can’t use PHP on the client-side but can compare the DatePicker value on the server-side. In this article, we will use two functions to compare the values we get from the DatePicker on the client-side and a PHP library for DatePickers.
Use strtotime()
and DateTime()
to Create Date Within PHP
Using JS DatePicker
, the value of the date the user picks will most likely be stored in a string. We will use the strtotime()
function to allow for good usage.
In this example, we can compare the user’s time with the baseline date to know if the user is above 18.
Code:
<html>
<head>
<title>PHP Test</title>
</head>
<body>
<?php
function above_18($arg) {
$baseline_date = strtotime("2004-03-26");
$users_date = strtotime($arg);
if ($user_date < $baseline_date) {
return "You are below the age of 18.";
} else {
return "You are above the age of 18. Continue with your registration process";
}
}
// Obtain the date of birth of user
// and place as the argument for the above_18() function
$notification = above_18("2007-10-29");
print_r($notification);
?>
</body>
</html>
Output:
You are below the age of 18.
Now, let’s use the DateTime()
method to achieve the same results, where the DatePicker
value is different from what’s on the server-side.
Code:
<html>
<head>
<title>PHP Test</title>
</head>
<body>
<?php
function above_18($arg) {
$baseline_date = new DateTime("2004-03-26");
$users_date = new DateTime($arg);
if ($user_date < $baseline_date) {
return "You are below the age of 18.";
} else {
return "You are above the age of 18. Continue with your registration process";
}
}
// Obtain the date of birth of user
// and place as the argument for the above_18() function
$notification = above_18("07-10-29");
print_r($notification);
?>
</body>
</html>
Output:
You are below the age of 18.
Use Essential JS for PHP for DatePicker
Capabilities
Aside from just comparing the JS DatePicker
values, we can use a third-party library called Essential JS for PHP to create a PHP DatePicker
.
In the following example, we’ll create a PHP DatePicker
control by invoking the PHP wrapper class from the EJ
namespace and set minimum and maximum dates via the minDate
and maxDate
methods.
Code:
<?php
$date = new EJ\DatePicker("datePicker");
echo $date->value(new DateTime())->
minDate(new DateTime("11/1/2016"))->
maxDate(new DateTime("11/24/2016"))->
render();
?>
Output:
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn