How to Calculate the Difference Between Two Dates Using PHP
-
Use
strtotime()
to Calculate the Difference Between Two Dates for PHP Version Below 5.3 -
Use
DateTime()
andDateInterval()
Objects for PHP 5.3 and Above
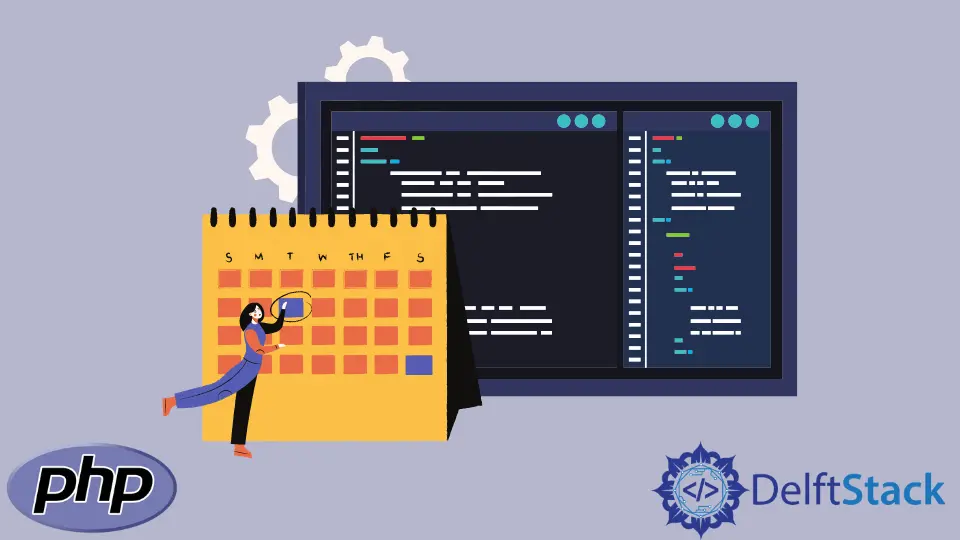
There are several ways to calculate the difference between two dates using PHP.
This article will show you how to use PHP version < 5.3 and the newest and more reliable method for the newer version (>5.3) to calculate the dates difference.
Use strtotime()
to Calculate the Difference Between Two Dates for PHP Version Below 5.3
The strtotime()
converts two dates to UNIX time and calculate the number of seconds from them. From this, it’s easier to calculate different methods using seconds. The function accepts a string parameter in English which shows the description of date-time.
Example:
$firstDate = "2019-01-01";
$secondDate = "2020-03-04";
$dateDifference = abs(strtotime($secondDate) - strtotime($firstDate));
$years = floor($dateDifference / (365 * 60 * 60 * 24));
$months = floor(($dateDifference - $years * 365 * 60 * 60 * 24) / (30 * 60 * 60 * 24));
$days = floor(($dateDifference - $years * 365 * 60 * 60 * 24 - $months * 30 * 60 * 60 *24) / (60 * 60 * 24));
echo $years." year, ".$months." months and ".$days." days";
//output: 1 year, 2 months and 3 days
The example above get the difference of two dates using several functions like abs()
, floor()
and mainly the strtotime()
.
The strtotime()
gets the UNIX seconds value of the dates and using abs()
to have the absolute value of the first date.
The function floor()
is used to round off the seconds down to the nearest integer.
To get the year, multiply 365 days to 60 minutes, 60 seconds and 24 hours, then divided it to the difference.
To get the months, multiply 30 days to 60 minutes, 60 seconds and 24 hours and divide it to the difference of the date difference multiplied to the year and 365 days, 60 minutes, 60 seconds and 24 hours.
Then to get the day difference, multiply 60 minutes, 60 seconds and 24 hours then divide it to the difference of the date difference, product of years, 365 days, 60 minutes, 60 seconds and 24 hours and the products of months, 30 days, 60 minutes and 60 seconds and 24 hours.
Finally, put it all together in a variable to print the final difference.
Use DateTime()
and DateInterval()
Objects for PHP 5.3 and Above
This method is an object-oriented style to get the difference between two dates, this is also the easiest since it doesn’t require the manual computation of the dates and recommended since it’s from the newest version of PHP.
Example:
$firstDate = new DateTime("2019-01-01");
$secondDate = new DateTime("2020-03-04");
$intvl = $firstDate->diff($secondDate);
echo $intvl->y . " year, " . $intvl->m." months and ".$intvl->d." day";
echo "\n";
// Total amount of days
echo $intvl->days . " days ";
//output: 1 year, 2 months and 1 day
// 428 days
In the example above, unlike using strtotime()
and more other functions and computations, DateTime()
and DateInterval()
objects made it more easier.
First, declare the dates using new DateTime()
. Then, get the difference’s integer value using the DateInterval()
object diff()
on the first date and pass the second date as the parameter.
Then finally, to get the year
just use the object y
. Use m
to get the months and d
for the day.
Related Article - PHP Date
- How to Add Days to Date in PHP
- How to Convert DateTime to String in PHP
- How to Convert String to Date and Date-Time in PHP
- How to Convert One Date Format to Another in PHP
- How to Get the Current Date and Time in PHP