How to Get the Current Date and Time in PHP
-
Use
date()
andtime()
Function to Get the Current Date and Time in PHP -
Use
DateTime
Object to Get the Current Date and Time in PHP
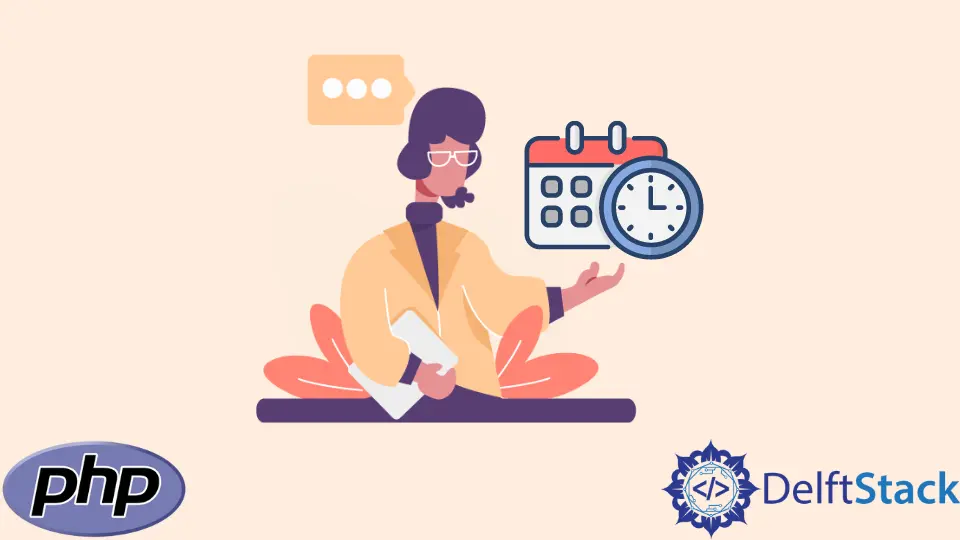
In this article, we will introduce methods to get the current date
and time
in PHP.
- Using
date()
andtime()
function - Using the
DateTime
object
Use date()
and time()
Function to Get the Current Date and Time in PHP
We could use built-in functions date()
and time()
to get the current date
and time
in PHP. These functions display the current date and time irrespective of the time zone. The correct syntax to use these two functions is as follows.
date($format, $timestamp);
The built-in function date()
has two parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
$format |
mandatory | Date format to be displayed. It has several variations. It should be a string. |
$timestamp |
optional | Timestamp to get the date. If it is omitted, then the current date is returned. |
It returns the date
that is set according to the specified format.
time();
The time()
function accepts no parameters. It returns the current PHP timestamp. The timestamp is the number of seconds calculated since the Unix Epoch.
The program below shows how to get the current date
and time
irrespective of the time zone.
<?php
$DateAndTime = date('m-d-Y h:i:s a', time());
echo "The current date and time are $DateAndTime.";
?>
The format "m-d-Y h:i:s a"
specifies the returned date
with month, day and 4-digit year value, and the time
in hours, minutes, and seconds.
Output:
The current date and time are 05-20-2020 05:44:03 pm.
One thing to note here is that the time
is not set according to some time zone. It is the time displayed using Unix timestamp. If you want to check the current PHP time
based on your time zone, you need to set the time zone manually.
For example, if you want to check the current time
in Amsterdam, you have to set the time zone for Amsterdam manually. For this purpose, the PHP function date_default_timezone_set()
is used. The correct syntax to use this function is as follows
date_default_timezone_set($timezone);
It accepts only one parameter.
Parameters | Description | |
---|---|---|
$timezone |
mandatory | It is a string that identifies the region for which we wish to set the time zone. You can check the list of supported time zones here. |
This function returns a bool value. If the time zone is set successfully, it returns true
; otherwise, it returns false
.
The program below will display the time after setting the time zone.
<?php
date_default_timezone_set('Europe/Amsterdam');
$DateAndTime = date('m-d-Y h:i:s a', time());
echo "The current date and time in Amsterdam are $DateAndTime.\n";
date_default_timezone_set('America/Toronto');
$DateAndTime2 = date('m-d-Y h:i:s a', time());
echo "The current date and time in Toronto are $DateAndTime2.";
?>
Output:
The current date and time in Amsterdam are 05-20-2020 08:08:42 pm.
The current date and time in Toronto are 05-20-2020 02:08:42 pm.
Use DateTime
Object to Get the Current Date and Time in PHP
In PHP, DateTime
class is used to deal with the problems related to the date
and time
. The format()
function of this class displays the date and time in a specified format.
We will first create a DateTime
object and then call format()
function to display the date and time.
$ObjectName = new DateTime();
$ObjectName->format($format);
The function format()
accepts one parameter.
Parameters | Description | |
---|---|---|
$format |
mandatory | Format of the date and time. It should be a string. |
The example program that displays the current date
and time
is as follows.
<?php
$Object = new DateTime();
$DateAndTime = $Object->format("d-m-Y h:i:s a");
echo "The current date and time are $DateAndTime.";
?>
The output is the date
and time
with the specified format. The time displayed is the time calculated since the Unix Epoch.
Output:
The current date and time are 20-05-2020 06:21:06 pm.
If you want to check the time according to a specific time zone, you will have to set the time zone manually. For this purpose, the PHP built-in function of DateTime
class setTimezone()
is used. The correct syntax to use this function is as follows.
$ObjectName = new DateTime();
$ObjectName->setTimezone(DateTimezone $timezone);
The function setTimezone()
accepts only one parameter. It returns a DateTime
object on success and False
on failure.
Parameter | Description | |
---|---|---|
$timezone |
mandatory | It is a DateTimezone object that specifies the time zone. You can check the list of supported time zones here. |
The example program that displays the time
according to a specific time zone is as follows.
<?php
$Object = new DateTime();
$Object->setTimezone(new DateTimeZone('Europe/Amsterdam'));
$DateAndTime = $Object->format("d-m-Y h:i:s a");
echo "The current date and time in Amsterdam are $DateAndTime.\n";
$Object->setTimezone(new DateTimeZone('America/Toronto'));
$DateAndTime = $Object->format("d-m-Y h:i:s a");
echo "The current date and time in Toronto are $DateAndTime.";
?>
Output:
The current date and time in Amsterdam are 20-05-2020 08:43:02 pm.
The current date and time in Toronto are 20-05-2020 02:43:02 pm.
Related Article - PHP Date
- How to Add Days to Date in PHP
- How to Calculate the Difference Between Two Dates Using PHP
- How to Convert DateTime to String in PHP
- How to Convert String to Date and Date-Time in PHP
- How to Convert One Date Format to Another in PHP