How to Convert a Date to a Timestamp in PHP
-
Use
strtotime()
Function to Convert a Date to a Timestamp in PHP -
Use
strptime()
Function to Convert a Date to a Timestamp in PHP -
Use
getTimestamp()
Function to Convert a Date to a Timestamp in PHP -
Use
format()
Function to Convert a Date to a Timestamp in PHP
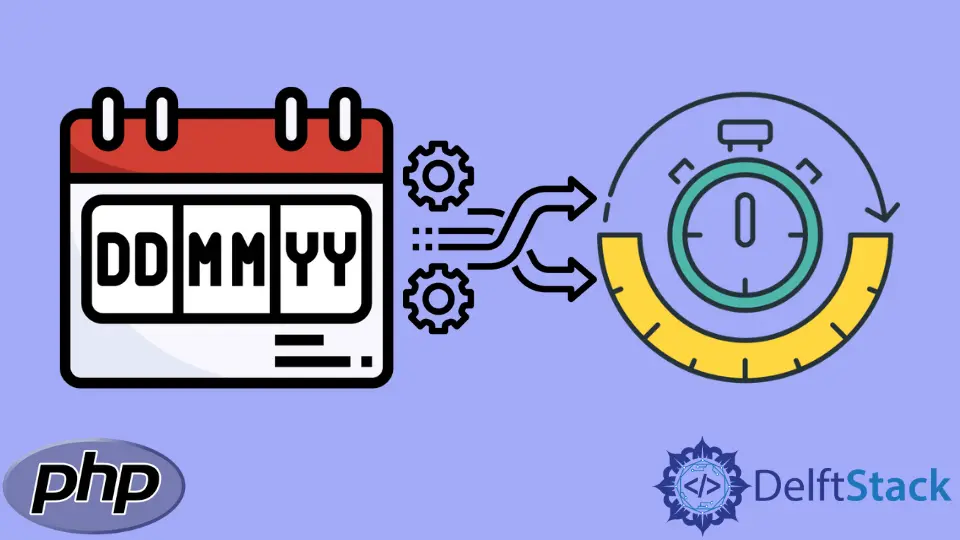
In this article, we will introduce methods to convert a date
to a timestamp
in PHP.
- Using
strtotime()
function - Using
strptime()
function - Using
getTimestamp()
function - Using
format()
function
Use strtotime()
Function to Convert a Date to a Timestamp in PHP
The built-in function strtotime()
converts a date to a Unix timestamp
. A Unix timestamp is the total number of seconds calculated from the Unix epoch(January 1st, 1970). The correct syntax to use this function is as follows
strtotime($dateString,$timeNow);
This function has two parameters. $dateString
is the date/time string that should comply with PHP valid format. It is a mandatory parameter. The other parameter $timeNow
is optional, it is the timestamp that is used for calculating relative dates. The current time now
is the default value if the second parameter is omitted.
<?php
$timestamp = strtotime("23-04-2020");
echo "The timestamp is $timestamp.";
?>
The date here is in the format "d-m-Y"
. We have only passed a single parameter as it will convert the date
to a Unix timestamp
.
Output:
The timestamp is 1587600000.
Use strptime()
Function to Convert a Date to a Timestamp in PHP
This is another function to convert a date to a Unix timestamp
. It does not convert the date
directly to a timestamp
. It returns an array that tells about the seconds, minutes, hours, and several other details. We can use these details to convert a date
to a timestamp
.
strptime($dateString, $format);
It has two mandatory parameters. $dateString
is the date string and $format
is the format to parse $dateString
.
<?php
$array = strptime('23-04-2020', '%d-%m-%Y');
$timestamp = mktime(0, 0, 0, $array['tm_mon']+1, $array['tm_mday'], $array['tm_year']+1900);
echo "The timestamp is $timestamp.";
?>
Output:
The timestamp is 1587600000.
After generating the array, mktime()
function converts the date
to a timestamp
.
The syntax of mktime()
function is
mktime(hour, minute, second, month, day, year, is_dst)
is_dst
specifies whether the date-time is a daylight saving time, but is removed from PHP 7.0.0.
$array['tm_mon']
and then use the sum as parameter of month
in mktime
, because $array['tm_mon']
starts from 0
, or in other words, $array['tm_mon']
of January is 0.Use getTimestamp()
Function to Convert a Date to a Timestamp in PHP
getTimestamp()
method of DateTime
object is a simple method to convert a date to a timestamp. It has another way of representation date_timestamp_get()
that is the procedural style representation.
$datetimeObject->getTimestamp();
We will create a Datetime
object to call this function. This is the object-oriented style of calling a function.
<?php
$date = new DateTime('2020-04-23');
$timestamp = $date->getTimestamp();
echo "The timestamp is $timestamp.";
?>
The object $date
of Datetime class has called the method getTimestamp()
to convert the date
to a Unix timestamp
.
Output:
The timestamp is 1587600000.
Use format()
Function to Convert a Date to a Timestamp in PHP
We can also use the format()
method of DateTime
to convert a date
to a timestamp
. This method has another representation date_format()
that is the procedural style representation of the format()
function.
$datetimeObject->format("U");
To convert a date
to a timestamp
, the format that we will pass as a string is "U"
.
<?php
$dateObject = new DateTime('2020-04-23');
$timestamp = $dateObject->format("U");
echo "The timestamp is $timestamp.";
?>
The object $dateObject
of the Datetime
class has called the function format()
to convert the date to a Unix timestamp
.
Output:
The timestamp is 1587600000.
Related Article - PHP DateTime
- How to Get the Last Day of the Month With PHP Functions
- How to Calculate the Difference Between Two Dates Using PHP
- How to Convert DateTime to String in PHP
- How to Get the Current Year in PHP
- How to Convert a Timestamp to a Readable Date or Time in PHP