How to Convert One Date Format to Another in PHP
-
Use
date()
andstrtotime()
Functions to Convert One Date Format to Another in PHP -
Use
createFromFormat()
andformat()
Functions to Convert One Date Format to Another in PHP
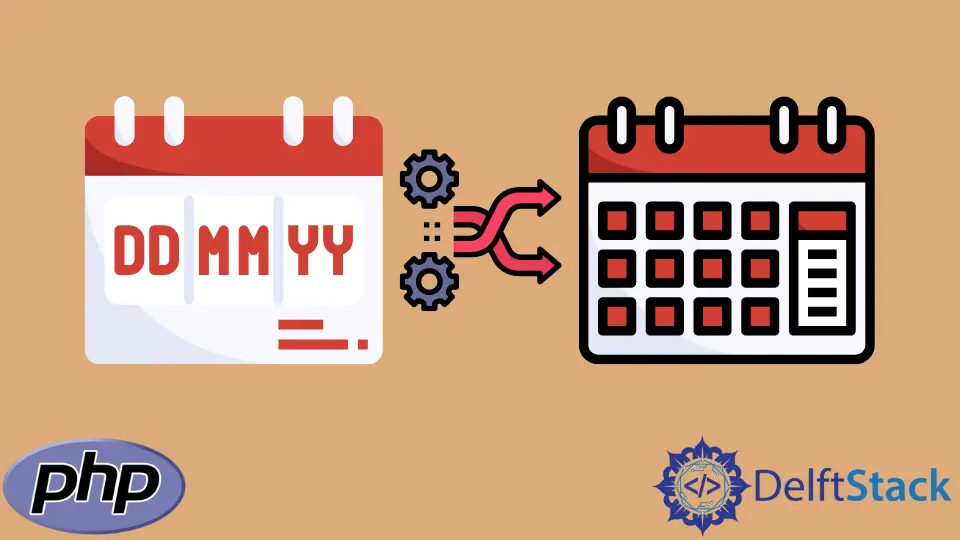
In this article, we will introduce methods to convert one Date
format to another in PHP.
- Using
date()
andstrtotime()
function - Using
createFromFormat()
andformat()
function
Use date()
and strtotime()
Functions to Convert One Date Format to Another in PHP
The date()
function converts a timestamp to a date
. The correct syntax to use this function is as follows
date( $format, $timestamp);
$format
is the specific format in which the date
is converted.
$timestamp
is an optional parameter. It gives the date
according to the timestamp passed. If it omitted, then we will get the current date
.
The function strtotime()
is a built-in function in PHP. This function converts a date
to the time. The correct syntax to use this function is as follows.
strtotime($dateString, $timeNow);
$dateString
is a mandatory parameter, and it is the string representation of a date.
$timeNow
is an optional parameter. It is the timestamp that is used for calculating relative dates.
<?php
$originalDate = "2020-04-29";
//original date is in format YYYY-mm-dd
$timestamp = strtotime($originalDate);
$newDate = date("m-d-Y", $timestamp );
echo "The new date is $newDate.";
?>
We have used date()
and strtotime()
function to convert one date
format to another. The function strtotime()
has converted the original date to a timestamp. This timestamp is then converted to date
of the required format using date()
function.
Output:
The new date is 04-29-2020.
Use createFromFormat()
and format()
Functions to Convert One Date Format to Another in PHP
The function createFromFormat()
is a built-in function in PHP. This function converts a timestamp or date string to a DateTime
object. The correct syntax to use this function is as follows.
DateTime::createFromFormat($format, $time, $timezone);
The variable $format
is the format of the date, $time
is the time or date
given in string, and $timezone
gives the time zone. The first two parameters are the mandatory parameters.
The format()
function is used to format a date
to the required format. The correct syntax to use this function is
$datetimeObject->format($formatString);
The parameter $formatString
specifies the required format.
<?php
$originalDate = "2020-04-29";
//original date is in format YYYY-mm-dd
$DateTime = DateTime::createFromFormat('Y-m-d', $originalDate);
$newDate = $DateTime->format('m-d-Y');
echo "The new date is $newDate.";
?>
Here, we have created a DateTime
object using createFromFormat()
function. The DateTime
object then calls the format()
function to convert one date
format to another.
Output:
The new date is 04-29-2020.