在 PHP 中使用 DatePicker
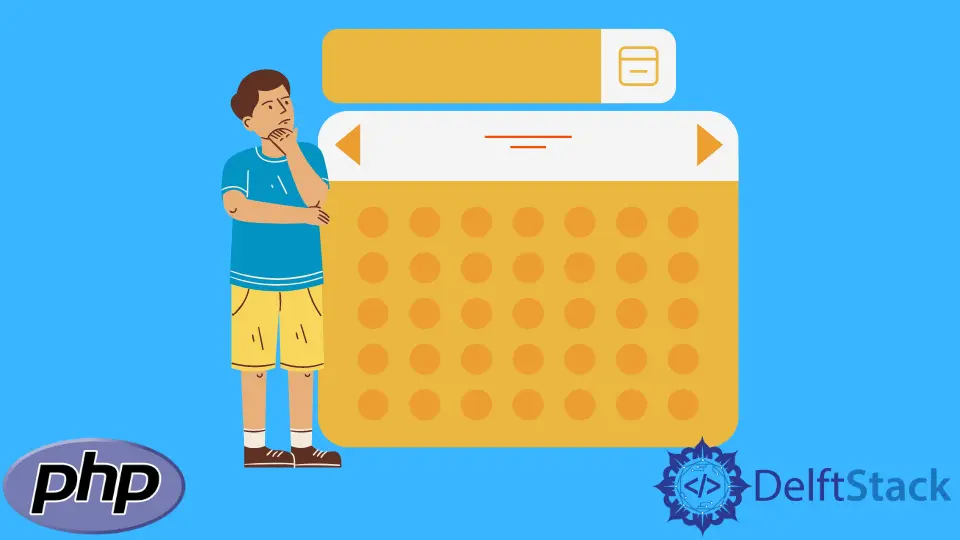
当我们在任何站点中填写表单时,我们可能需要使用 DatePicker 来选择日期。例如,出生日期或课程完成日期。
为此,你可以使用 HTML 和 JavaScript。但是,要使用日期,我们需要比较用户在服务器端选择的日期。
我们不能在客户端使用 PHP,但可以在服务器端比较 DatePicker 的值。在本文中,我们将使用两个函数来比较我们从客户端的 DatePicker 和 DatePicker 的 PHP 库中获得的值。
使用 strtotime()
和 DateTime()
在 PHP 中创建日期
使用 JS DatePicker
,用户选择的日期的值很可能存储在字符串中。我们将使用 strtotime()
函数来实现良好的使用。
在此示例中,我们可以将用户的时间与基线日期进行比较,以了解用户是否在 18 岁以上。
代码:
<html>
<head>
<title>PHP Test</title>
</head>
<body>
<?php
function above_18($arg) {
$baseline_date = strtotime("2004-03-26");
$users_date = strtotime($arg);
if ($user_date < $baseline_date) {
return "You are below the age of 18.";
} else {
return "You are above the age of 18. Continue with your registration process";
}
}
// Obtain the date of birth of user
// and place as the argument for the above_18() function
$notification = above_18("2007-10-29");
print_r($notification);
?>
</body>
</html>
输出:
You are below the age of 18.
现在,让我们使用 DateTime()
方法来实现相同的结果,其中 DatePicker
值与服务器端的值不同。
代码:
<html>
<head>
<title>PHP Test</title>
</head>
<body>
<?php
function above_18($arg) {
$baseline_date = new DateTime("2004-03-26");
$users_date = new DateTime($arg);
if ($user_date < $baseline_date) {
return "You are below the age of 18.";
} else {
return "You are above the age of 18. Continue with your registration process";
}
}
// Obtain the date of birth of user
// and place as the argument for the above_18() function
$notification = above_18("07-10-29");
print_r($notification);
?>
</body>
</html>
输出:
You are below the age of 18.
使用 Essential JS for PHP 实现 DatePicker
功能
除了比较 JS DatePicker
值之外,我们还可以使用名为 Essential JS for PHP 的第三方库来创建 PHP DatePicker
。
在下面的示例中,我们将通过调用 EJ
命名空间中的 PHP 包装类并通过 minDate
和 maxDate
方法设置最小和最大日期来创建一个 PHP DatePicker
控件。
代码:
<?php
$date = new EJ\DatePicker("datePicker");
echo $date->value(new DateTime())->
minDate(new DateTime("11/1/2016"))->
maxDate(new DateTime("11/24/2016"))->
render();
?>
输出:
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn