How to Display Date and Time According to Timezone in PHP
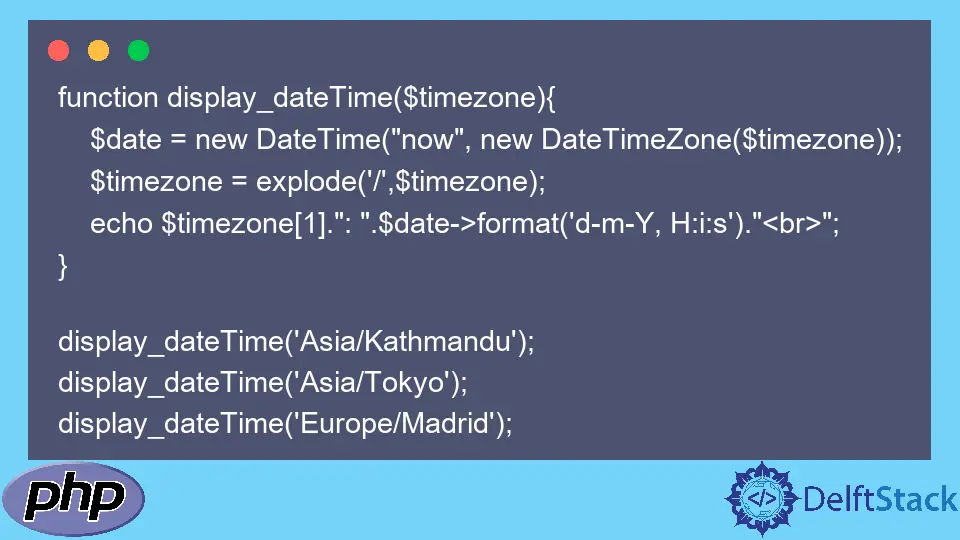
Timezone varies according to the place.
This article will teach us how to display time and date according to timezone in PHP.
Use the DateTime
Class to Display Time and Date According to Timezone in PHP
PHP provides a powerful class DateTime
to handle date and time operations. We can set the time and date using the DateTime
class.
The class returns an instance that contains the date, timezone type and timezone. The constructor function of the class takes two parameters.
The first parameter is the date/time string. The parameter should be in a valid format defined by PHP.
We can also use the now
string to get the current date/time if we use the timezone parameter as the second parameter. The second parameter is timezone.
We can use the instance of the DateTimeZone
class to get the specific timezone. We can set the timezone as the parameter of the constructor of the DateTimeZone
class.
Only the predefined timezone by PHP can be used. Using the DateTime
class with these parameters will obtain the current date/time according to the timezone.
For example, create a function display_dateTime()
with a parameter $timezone
. Inside the function, create an object of the DateTime
class.
Use "now"
as the first parameter and create an instance of the DateTimeZone()
class as the second parameter. Supply the $timezone
variable as the parameter of the DateTimeZone()
class.
Next, call the format()
method of the DateTime
class and supply the format as d-m-Y, H:i:s
. Use the echo
function to print it.
Outside the function, call the function multiple times with different timezones.
Use the timezones Asia/Kathmandu
, Asia/Tokyo
and Europe/Madrid
as the function arguments. These timezones should be a string.
Example Code:
function display_dateTime($timezone){
$date = new DateTime("now", new DateTimeZone($timezone));
$timezone = explode('/',$timezone);
echo $timezone[1].": ".$date->format('d-m-Y, H:i:s')."<br>";
}
display_dateTime('Asia/Kathmandu');
display_dateTime('Asia/Tokyo');
display_dateTime('Europe/Madrid');
We have trimmed the city’s name in the example above using the explode()
function. We have used the slash /
as the separator.
For instance, "Asia/Kathmandu"
will be formed as ["Asia, Kathmandu"]
as an array. We extracted the first index from the array to get only the city name.
As a result, we can see the different times according to the time zones.
Output:
Kathmandu: 07-06-2022, 11:27:35
Tokyo: 07-06-2022, 14:42:35
Madrid: 07-06-2022, 07:42:35
This way, we can set the time and date according to the timezones using PHP’s DateTime
class.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedInRelated Article - PHP Date
- How to Add Days to Date in PHP
- How to Calculate the Difference Between Two Dates Using PHP
- How to Convert DateTime to String in PHP
- How to Convert String to Date and Date-Time in PHP
- How to Convert One Date Format to Another in PHP
- How to Get the Current Date and Time in PHP