New York Timezone in PHP
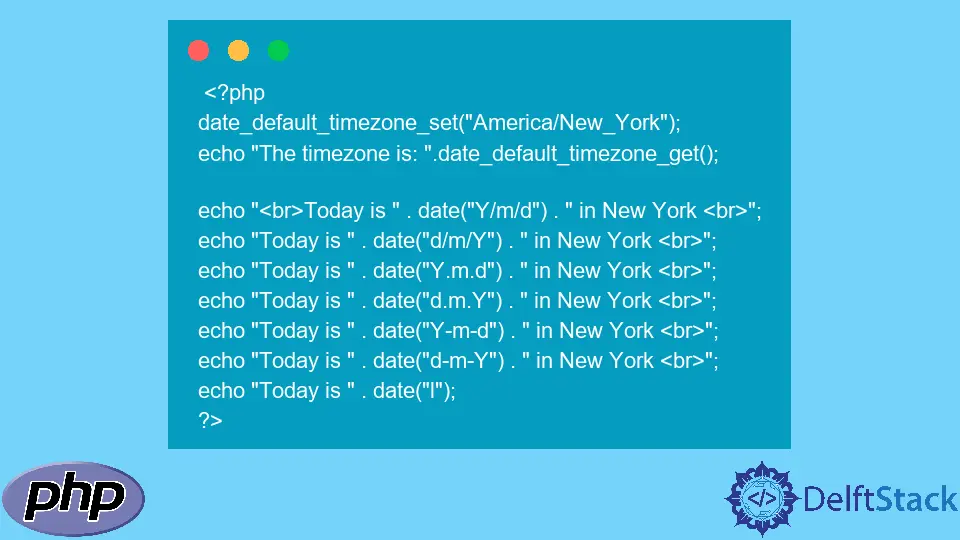
This tutorial demonstrates how to get the time in the New York timezone in PHP.
New York Timezone in PHP
The PHP manual provides the keywords for each timezone which can be found here. According to the PHP manual, the keyword for the New York timezone is America/New_York
.
But how do we get the time from the New_York
timezone in PHP? For that, we need to use two methods, first to set the timezone and second to get the timezone.
- The first method is
date_default_timezone_set()
, which takes thetimezone
keyword as a parameter and sets the timezone. The method will returnfalse
if the keyword value is not valid. - The second method is
date_default_timezone_get()
, which is used to get the current timezone.
Let’s set the timezone as New_York
and then show the current timezone.
<?php
date_default_timezone_set("America/New_York");
echo date_default_timezone_get();
?>
The code above will set the timezone and then echo
it by the date_default_timezone_get()
method. The output is:
America/New_York
Now let’s try to get the date and time for the New York timezone. To get the date and time for a timezone, we need to use the date()
method of PHP, which is used to format the date and time; it includes keywords, as described below.
d
- Used for the day of the month (01 to 31)m
- Used to get a month (01 to 12)Y
- Used to get a year (in four digits)l
- Used to get the day of the week (Lowercase L)H
- Used to get the time in the 24-hour format of an hour (00 to 23)h
- Used to get the time in a 12-hour format of an hour with leading zeros (01 to 12)i
- Used to get the minutes with leading zeros (00 to 59)s
- Used to get the seconds with leading zeros (00 to 59)a
- Used for the Ante meridiem and Post meridiem (am or pm)
Now let’s see how to get the date in a different format for the New_York
timezone.
<?php
date_default_timezone_set("America/New_York");
echo "The timezone is: ".date_default_timezone_get();
echo "Today is " . date("Y/m/d") . " in New York <br>";
echo "Today is " . date("d/m/Y") . " in New York <br>";
echo "Today is " . date("Y.m.d") . " in New York <br>";
echo "Today is " . date("d.m.Y") . " in New York <br>";
echo "Today is " . date("Y-m-d") . " in New York <br>";
echo "Today is " . date("d-m-Y") . " in New York <br>";
echo "Today is " . date("l");
?>
The code above will show the date for the New_York
timezone in different formats. See output:
The timezone is: America/New_York
Today is 2022/09/19 in New York
Today is 19/09/2022 in New York
Today is 2022.09.19 in New York
Today is 19.09.2022 in New York
Today is 2022-09-19 in New York
Today is 19-09-2022 in New York
Today is Monday
Now let’s see how to get the time for the New_York
timezone.
<?php
date_default_timezone_set("America/New_York");
echo "The timezone is: ".date_default_timezone_get();
echo "The current time in New York is " . date("h:i:sa");
?>
The code above will show the current time in New_York
. See output:
The timezone is: America/New_York
The current time in New York is 03:32:52am
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook