How to Get a File Extension in PHP
-
Use the
pathinfo()
Function to Get File Extension in PHP -
Use
SplFileInfo()
Construct andgetExtension()
Function to Get File Extension in PHP -
Use the
explode()
Function to Get File Extension in PHP -
Use the
substr()
andstrrpos()
Functions to Get File Extension in PHP -
Use the
preg_replace()
Function to Get File Extension in PHP
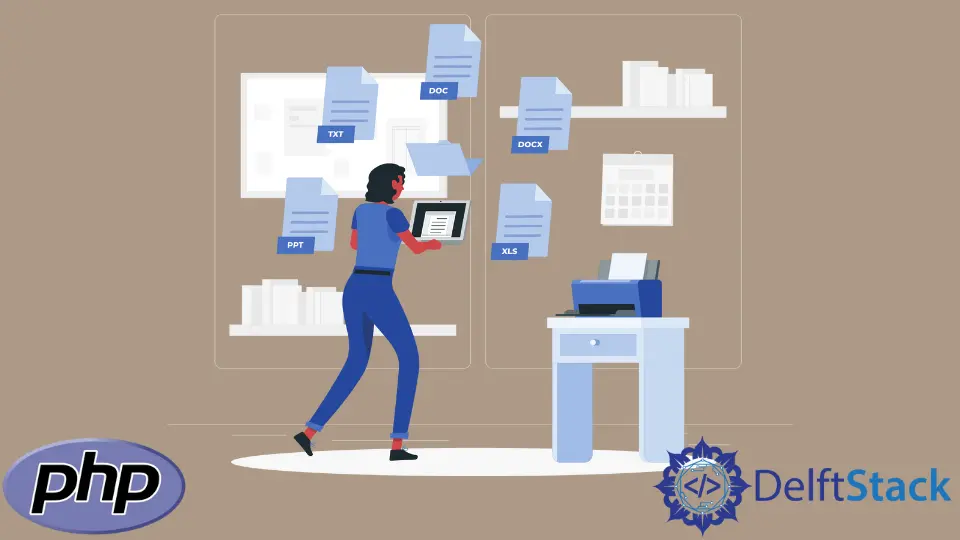
In this article, we will explore different techniques, including the use of built-in functions and regular expressions, to extract file extensions from file names.
These methods will empower you to efficiently manage and manipulate files based on their extensions.
Use the pathinfo()
Function to Get File Extension in PHP
We will use the built-in function pathinfo()
to get the file extension. This function extracts the path information from the given path. The correct syntax to use this function is as follows.
pathinfo($pathName, $options);
The built-in function pathinfo()
has two parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
$pathName |
mandatory | It is the string containing the path with file name and extension. We will extract path info from this string . |
$options |
optional | This parameter specifies the path elements. For example, if we want to find a file name only, we can pass PATHINFO_FILENAME as an option. The other options are PATHINFO_DIRNAME , PATHINFO_BASENAME , and PATHINFO_EXTENSION . |
This function returns an associative array containing a directory name, base name, extension, and file name. If the $option
parameter is passed, it returns a string.
The program below shows can we use the pathinfo()
function to get file extension.
<?php
$path = "E:\work\CM\myppt.ppt";
$extension = pathinfo($path, PATHINFO_EXTENSION);
echo("The extension is $extension.");
?>
We have passed the $option
parameter. The function has returned a string containing file extension.
Output:
The extension is ppt.
If we don’t pass the $option
parameter, the function will return an associative array.
<?php
$path = "E:\work\CM\myppt.ppt";
$array = pathinfo($path);
echo("The associative array is: \n");
var_dump($array);
?>
Output:
The associative array is:
array(4) {
["dirname"]=>
string(1) "."
["basename"]=>
string(20) "E:\work\CM\myppt.ppt"
["extension"]=>
string(3) "ppt"
["filename"]=>
string(16) "E:\work\CM\myppt"
}
Use SplFileInfo()
Construct and getExtension()
Function to Get File Extension in PHP
In PHP, we can also use SplFileInfo()
construct to get the file extension. This construct will create a new SplFileInfo
object. After that we can use getExtension()
function to get the file extension. The correct syntax to use this construct is as follows:
$variableName = new SplFileInfo($pathName);
The construct SplFileInfo()
accepts one parameter. The detail of its parameter is as follows
Parameters | Description | |
---|---|---|
$pathName |
mandatory | It is the string that contains our file’s path. We will use this string to extract file extension. |
We will use getExtension()
function to get the file extension. The correct syntax to use this function is as follows:
getExtension(void);
This function accepts no parameters. It returns file extension.
The program that gets the file extension is as follows:
<?php
$path = "E:\work\CM\myppt.ppt";
$file = new SplFileInfo($path);
$extension = $file->getExtension();
echo("The extension is: $extension.");
?>
Output:
The extension is: ppt.
Use the explode()
Function to Get File Extension in PHP
The explode()
function in PHP allows you to split a string into an array of substrings based on a specified delimiter.
In the context of extracting file extensions, you can split the file name using the dot (.
) as the delimiter. The last part of the resulting array will be the file extension.
Here’s how you can do it step by step.
Steps
- Specify the File Name: Assume you have a file name as shown below:
$filename = 'image.jpg';
- Split the File Name: Use the
explode()
function to split the file name using the dot (.
) delimiter. Retrieve the last part of the resulting array to get the file extension.
<?php
$parts = explode('.', $filename);
$extension = end($parts);
echo "File: $filename, Extension: $extension"
?>
- Output: Run the program, and you’ll see the file name along with its corresponding file extension printed in the console.
Complete Code Example
Here’s the complete code example that demonstrates how to use the explode()
function to extract a file extension from a file name:
<?php
$filename = 'image.jpg';
$parts = explode('.', $filename);
$extension = end($parts);
echo "File: $filename, Extension: $extension"
?>
When you run the program, you’ll see the following output:
File: image.jpg, Extension: jpg
Use the substr()
and strrpos()
Functions to Get File Extension in PHP
The substr()
function in PHP is used to extract a substring from a given string. It takes three parameters: the input string, the starting position of the substring, and the length of the substring to extract.
In the context of extracting file extensions, you can use the substr()
function to target the portion of the string that represents the extension.
Steps
- Find the Position of the Last Dot: Use the
strrpos()
function to find the position of the last dot (.
) in the file name. This position will be the starting point for extracting the file extension.
$dotPosition = strrpos($filename, '.');
- Extract the Extension: Use the
substr()
function to extract the substring starting from the position of the last dot to the end of the string.
$extension = substr($filename, $dotPosition + 1);
- Output: Print the extracted file extension along with the original file name.
echo "File: $filename, Extension: $extension" . PHP_EOL;
Complete Code Example
Here’s the complete code example that demonstrates how to use the substr()
function to extract a file extension from a file name:
<?php
$filename = 'image.jpg';
$dotPosition = strrpos($filename, '.');
$extension = substr($filename, $dotPosition + 1);
echo "File: $filename, Extension: $extension" . PHP_EOL;
When you run the program, you’ll see the following output:
File: image.jpg, Extension: jpg
Use the preg_replace()
Function to Get File Extension in PHP
The preg_replace()
function in PHP is primarily used for performing regular expression-based replacements in strings. While not its primary purpose, it can also aid in extracting specific parts of a string based on a pattern, making it useful for isolating file extensions.
Step-by-Step Guide
Here’s a comprehensive guide on using the preg_replace()
function to extract a file extension from a file name:
-
Regular Expression Pattern: You can create a regular expression pattern to match the last dot (
.
) and everything that follows it. This pattern will capture the file extension.$pattern = '/\.[^.]*$/';
-
Perform the Replacement: Apply the
preg_replace()
function to replace the matched pattern with an empty string. This effectively removes everything except the file extension.$extension = preg_replace($pattern, '', $filename);
-
Output the Result: Print the extracted file extension alongside the original file name.
echo "File: $filename, Extension: $extension" . PHP_EOL;
Complete Code Example
Here’s the complete code example illustrating the use of preg_replace()
to extract a file extension from a file name:
<?php
$filename = 'picture.jpg';
$pattern = '/\.[^.]*$/';
$extension = preg_replace($pattern, '', $filename);
echo "File: $filename, Extension: $extension"
?>