How to Divide Matrix by Vector in NumPy
- Divide Matrix by Vector Using NumPy Broadcasting in Python
- Divide Matrix by Vector Using the NumPy Transpose Method in Python
-
Divide Matrix by Vector Using the
numpy.reshape()
Function - Conclusion
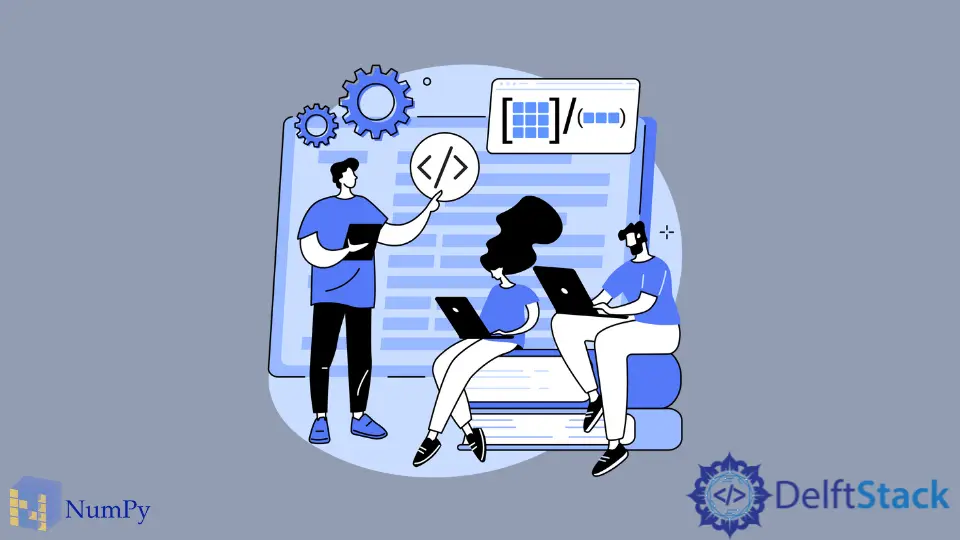
This tutorial will discuss the methods to divide a matrix by a vector in NumPy. We will walk you through the process step by step, demonstrating various methods to achieve this operation.
Divide Matrix by Vector Using NumPy Broadcasting in Python
NumPy broadcasting allows arrays with different shapes to be combined in arithmetic operations. This facilitates element-wise operations on arrays even if their shapes are not identical, making it easy to perform operations like matrix division by a vector.
In the context of matrix division by a vector, broadcasting allows us to expand the dimensions of the vector to match the shape of the matrix, making element-wise division possible.
We’ll follow the following steps to divide a matrix by a vector using NumPy broadcasting:
-
Begin by importing the NumPy library, which provides essential functions for numerical operations.
-
Define the matrix and vector that you want to use for the division operation.
-
Adjust the dimensions of the vector using NumPy functions to make it compatible with the matrix for broadcasting.
-
Perform the division operation using the expanded vector and the matrix. NumPy will automatically apply the operation element-wise, thanks to broadcasting.
Let’s walk through the implementation of the above method:
import numpy as np
matrix = np.array([[2, 2], [4, 4]])
vector = np.array([2, 4])
result_broadcast = matrix / vector[:, np.newaxis]
result_divide = np.divide(matrix, vector[:, np.newaxis])
print("Result using broadcasting:")
print(result_broadcast)
print("Result using np.divide():")
print(result_divide)
Output:
Result using broadcasting:
[[1. 1.]
[1. 1.]]
Result using np.divide():
[[1. 1.]
[1. 1.]]
In this code, the NumPy library is imported and aliased as np
to facilitate numerical operations. A matrix and a vector are defined using NumPy arrays, where the matrix is a 2x2 array with specified integer values, and the vector is a 1D array.
The code demonstrates two methods of element-wise division between the matrix and the vector.
The first method utilizes broadcasting, a NumPy feature that enables operations between arrays of different shapes. Specifically, the matrix
is divided by the vector
after reshaping the latter into a 2D column vector using broadcasting.
For the second method, the code employs the np.divide()
function to achieve the same result as the first method.
Finally, the resulting arrays from both methods are printed to the console, showcasing the division outcomes achieved through broadcasting and the np.divide()
function.
Divide Matrix by Vector Using the NumPy Transpose Method in Python
Another approach involves transposing the matrix. The transpose of a matrix involves switching its rows and columns.
When we divide a matrix by a vector using the transpose method, we essentially divide each row of the matrix by each element of the vector. After performing this element-wise division, we transpose the result back to the original matrix orientation.
Let’s see the implementation of this method:
import numpy as np
matrix = np.array([[2, 2, 2], [4, 4, 4], [6, 6, 6]])
vector = np.array([2, 4, 6])
matrix = (matrix.T / vector).T
print(matrix)
Output:
[[1. 1. 1.]
[1. 1. 1.]
[1. 1. 1.]]
After defining the matrix and the vector, the code then performs element-wise division of the transpose of the matrix by the vector and assigns the result back to the matrix.
The .T
operation transposes the matrix, enabling element-wise division to be performed on each row of the matrix using the corresponding value from the vector.
The transpose is taken again to restore the original matrix shape. Finally, the resulting matrix is printed.
Divide Matrix by Vector Using the numpy.reshape()
Function
The numpy.reshape()
method allows us to modify the shape of an array without changing its data. When dividing a matrix by a vector using this method, we reshape the vector to have dimensions compatible with the matrix, enabling element-wise division.
Take a look at the implementation of this method:
import numpy as np
matrix = np.array([[2, 2, 2], [4, 4, 4], [6, 6, 6]])
vector = np.array([2, 4, 6])
matrix = matrix / vector.reshape((3, 1))
print(matrix)
Output:
[[1. 1. 1.]
[1. 1. 1.]
[1. 1. 1.]]
The code above performs element-wise division of the matrix by the vector. However, before performing the division, the vector is reshaped to have dimensions (3,1)
to align with the matrix’s dimensions.
This reshaping ensures that the division is carried out element-wise for each corresponding element in the matrix and vector, with the vector being broadcasted accordingly.
Finally, the resulting matrix, obtained through element-wise division, is printed.
Conclusion
In this article, we’ve uncovered three different approaches to dividing a matrix by a vector using NumPy, showcasing the library’s flexibility and power in handling operations with mismatched shapes.
Whether you choose broadcasting, the transpose method, or the numpy.reshape()
function, you now have the tools at your disposal to perform element-wise matrix-vector division with ease.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn