How to Normalize a Vector in Python
- Understanding Vector Normalization
- Method 1: Using NumPy
- Method 2: Using Pure Python
- Method 3: Using Scikit-learn
- Conclusion
- FAQ
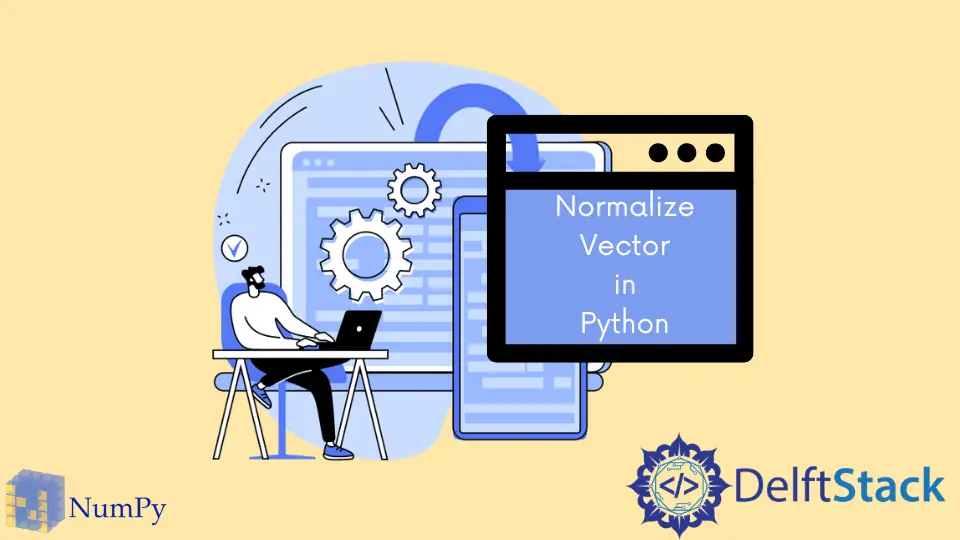
Normalizing a vector is a fundamental operation in various fields, including machine learning, physics, and computer graphics. In simple terms, vector normalization transforms a vector into a unit vector, which retains its direction but has a length of one. This process is crucial for ensuring that different vectors can be compared on a common scale.
In this tutorial, we will explore how to normalize a vector in Python using various methods. By the end of this article, you will have a solid understanding of vector normalization and practical examples to implement it in your projects.
Understanding Vector Normalization
Before diving into the code, let’s clarify what vector normalization means. A vector can be represented as an array of numbers. The normalization process involves dividing each element of the vector by its magnitude, which is calculated using the Euclidean norm. The formula for the magnitude of a vector ( \mathbf{v} ) is:
[
\text{magnitude} = \sqrt{v_1^2 + v_2^2 + … + v_n^2}
]
Once we have the magnitude, normalizing the vector ( \mathbf{v} ) is straightforward:
[
\mathbf{u} = \frac{\mathbf{v}}{\text{magnitude}}
]
Now, let’s explore different methods to normalize a vector in Python.
Method 1: Using NumPy
NumPy is a powerful library for numerical computations in Python. It provides an efficient way to handle arrays and perform mathematical operations. Normalizing a vector using NumPy is both simple and efficient.
import numpy as np
vector = np.array([3, 4])
magnitude = np.linalg.norm(vector)
normalized_vector = vector / magnitude
print(normalized_vector)
Output:
[0.6 0.8]
In this code snippet, we first import the NumPy library. We then define our vector as a NumPy array. The np.linalg.norm()
function computes the magnitude of the vector. Finally, we divide the original vector by its magnitude to obtain the normalized vector. The output shows that the new vector retains the same direction but has a length of one.
Using NumPy for vector normalization is efficient, especially when dealing with large datasets or multidimensional arrays. This method is widely used in data science and machine learning applications due to its speed and simplicity.
Method 2: Using Pure Python
If you prefer not to use external libraries, you can normalize a vector using pure Python. While this method may not be as efficient as using NumPy, it is straightforward and easy to understand.
import math
vector = [3, 4]
magnitude = math.sqrt(sum(x**2 for x in vector))
normalized_vector = [x / magnitude for x in vector]
print(normalized_vector)
Output:
[0.6, 0.8]
In this example, we use the built-in math
library to calculate the magnitude. The sum()
function computes the sum of the squares of each element in the vector. We then create a new list comprehension to divide each element by the magnitude. The result is the normalized vector, which is the same as in the previous method.
While this method is less efficient for large vectors, it provides a clear understanding of how normalization works under the hood. It’s a great option for educational purposes or small-scale applications.
Method 3: Using Scikit-learn
Scikit-learn is a popular machine learning library that includes a variety of preprocessing functions, including vector normalization. This method is beneficial when you are already using Scikit-learn in your project.
from sklearn.preprocessing import normalize
vector = [[3, 4]]
normalized_vector = normalize(vector)
print(normalized_vector)
Output:
[[0.6 0.8]]
In this code, we import the normalize
function from the Scikit-learn library. We wrap the vector in an additional list to match the expected input format. The normalize()
function takes care of calculating the magnitude and dividing each element accordingly. The output is a 2D array, which is consistent with the input format.
Using Scikit-learn’s normalization is particularly useful when working with datasets that require preprocessing before feeding into machine learning models. It saves time and ensures that your data is properly normalized.
Conclusion
In this tutorial, we explored three different methods to normalize a vector in Python: using NumPy, pure Python, and Scikit-learn. Each method has its advantages, depending on your project requirements and coding preferences. Whether you choose to use a powerful library like NumPy or stick with pure Python, normalizing vectors is a crucial skill in data analysis, machine learning, and scientific computing. Now that you have a solid understanding of vector normalization, you can apply these techniques in your own projects with confidence.
FAQ
-
What is vector normalization?
Vector normalization is the process of converting a vector into a unit vector, which has a magnitude of one while retaining its direction. -
Why is vector normalization important?
Normalization is important for comparing vectors on a common scale, especially in machine learning and data analysis. -
Can I normalize a vector without using external libraries?
Yes, you can normalize a vector using pure Python by calculating the magnitude and dividing each element manually. -
What is the difference between NumPy and pure Python for normalization?
NumPy is optimized for performance and can handle large datasets efficiently, while pure Python is more straightforward but may be slower for large vectors. -
Is Scikit-learn’s normalization suitable for machine learning projects?
Yes, Scikit-learn’s normalization is ideal for preprocessing data before feeding it into machine learning models.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn