NumPy Matrix Indexing
- Indexing Single-Dimensional Arrays
- Indexing Multi-Dimensional Arrays
- Advanced Indexing Techniques
- Conclusion
- FAQ
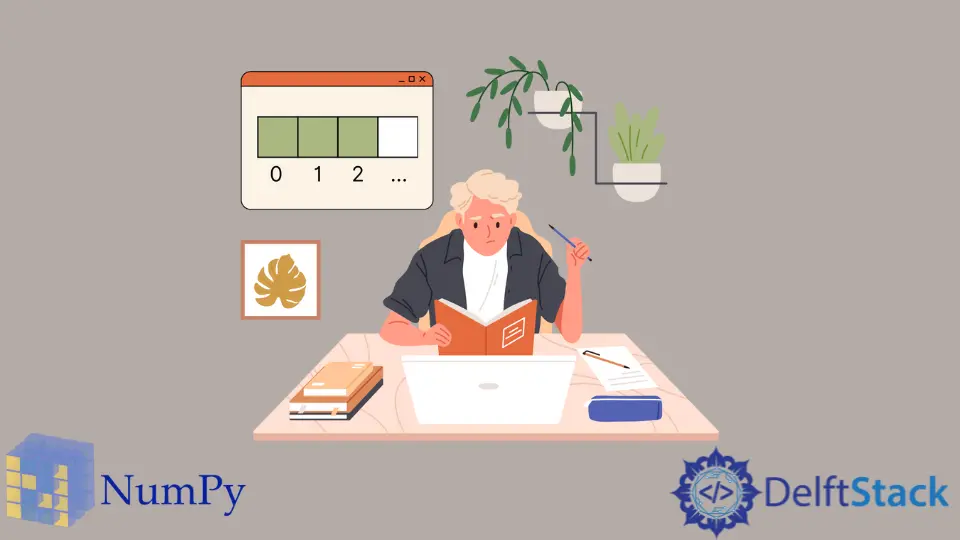
When working with numerical data in Python, NumPy is the go-to library for efficient computation. One of its most powerful features is matrix indexing, which allows you to access and manipulate data in multidimensional arrays with ease. However, understanding how indexing works can be a bit tricky, especially when comparing single-dimensional vectors to multi-dimensional matrices.
In this article, we’ll dive deep into NumPy matrix indexing, exploring various methods to access elements, rows, and columns. Whether you’re a beginner or looking to refine your skills, this guide will provide you with the insights needed to harness the full potential of NumPy.
Once NumPy is installed, you can import NumPy and create arrays. Here’s a simple example:
import numpy as np
array_1d = np.array([1, 2, 3, 4, 5])
array_2d = np.array([[1, 2, 3], [4, 5, 6]])
Output:
array_1d: [1 2 3 4 5]
array_2d: [[1 2 3]
[4 5 6]]
In this example, array_1d
is a one-dimensional array, while array_2d
is a two-dimensional matrix. Understanding this foundational concept is crucial for effective indexing.
Indexing Single-Dimensional Arrays
Indexing in single-dimensional arrays is straightforward. You can access elements using their integer index, which starts at zero. Here’s how you can do it:
single_array = np.array([10, 20, 30, 40, 50])
first_element = single_array[0]
last_element = single_array[-1]
slice_array = single_array[1:4]
Output:
first_element: 10
last_element: 50
slice_array: [20 30 40]
In the code above, single_array[0]
retrieves the first element, while single_array[-1]
gets the last element. Slicing is also possible, allowing you to extract a portion of the array. For instance, single_array[1:4]
returns elements from index 1 to 3, inclusive of the start index and exclusive of the end index. This simplicity makes single-dimensional indexing intuitive and user-friendly.
Indexing Multi-Dimensional Arrays
Multi-dimensional arrays require a slightly different approach. Each dimension is indexed separately, allowing you to access specific elements, rows, or columns. Here’s an example of how to index a two-dimensional array:
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
element = matrix[1, 2]
first_row = matrix[0, :]
second_column = matrix[:, 1]
Output:
element: 6
first_row: [1 2 3]
second_column: [2 5 8]
In this example, matrix[1, 2]
retrieves the element located in the second row and third column, which is 6. To access an entire row, you can use a colon (:
) to specify all columns, as shown with matrix[0, :]
, which returns the first row. Conversely, if you want to get an entire column, you can specify all rows while selecting a particular column, like matrix[:, 1]
, which returns the second column. This flexibility allows for powerful data manipulation.
Advanced Indexing Techniques
NumPy also offers advanced indexing techniques that can be incredibly useful for more complex operations. For instance, you can use boolean indexing and fancy indexing to filter and access data. Let’s explore both methods.
Boolean Indexing
Boolean indexing allows you to select elements based on certain conditions. Here’s how it works:
array = np.array([10, 20, 30, 40, 50])
condition = array > 25
filtered_array = array[condition]
Output:
filtered_array: [30 40 50]
In this example, we create a boolean condition to filter elements greater than 25. The resulting filtered_array
contains only those elements that meet the condition. This method is particularly useful for data analysis, as it enables you to quickly extract relevant information based on specific criteria.
Fancy Indexing
Fancy indexing allows you to access multiple array elements simultaneously using integer arrays. Here’s an example:
array = np.array([10, 20, 30, 40, 50])
indices = np.array([0, 2, 4])
fancy_array = array[indices]
Output:
fancy_array: [10 30 50]
In this code snippet, we define an array of indices and use it to retrieve multiple elements from array
. The result, fancy_array
, contains the elements at the specified indices. This technique is beneficial when you need to access non-contiguous elements efficiently.
Conclusion
NumPy matrix indexing is a powerful tool that can significantly enhance your data manipulation capabilities in Python. By understanding the differences between single-dimensional and multi-dimensional indexing, as well as advanced techniques like boolean and fancy indexing, you can unlock new levels of efficiency in your code. Whether you’re working on data analysis, machine learning, or scientific computing, mastering these indexing methods will make your work easier and more effective. With practice, you’ll find that NumPy is not just a library; it’s a game-changer in the world of numerical computing.
FAQ
- What is NumPy matrix indexing?
NumPy matrix indexing refers to the methods used to access and manipulate elements within NumPy arrays, particularly in single-dimensional and multi-dimensional formats.
-
How do I access elements in a one-dimensional NumPy array?
You can access elements in a one-dimensional array using their index, starting from zero, likearray[0]
for the first element. -
What is the difference between slicing and indexing?
Indexing retrieves a specific element, while slicing extracts a subset of the array. For example,array[1:4]
slices elements from index 1 to 3. -
Can I use conditions to filter elements in a NumPy array?
Yes, you can use boolean indexing to filter elements based on conditions, such asarray[array > 25]
. -
What is fancy indexing in NumPy?
Fancy indexing allows you to access multiple elements from an array using an array of indices, enabling efficient retrieval of non-contiguous elements.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn