在 NumPy 中按向量划分矩阵
Muhammad Maisam Abbas
2023年1月30日
NumPy
NumPy Vector
NumPy Matrix
- 在 NumPy 中用 Python 中的数组切片方法按向量划分矩阵
- 在 NumPy 中用 NumPy 中的转置方法按向量划分矩阵
-
在 NumPy 中使用
numpy.reshape()
函数按向量划分矩阵
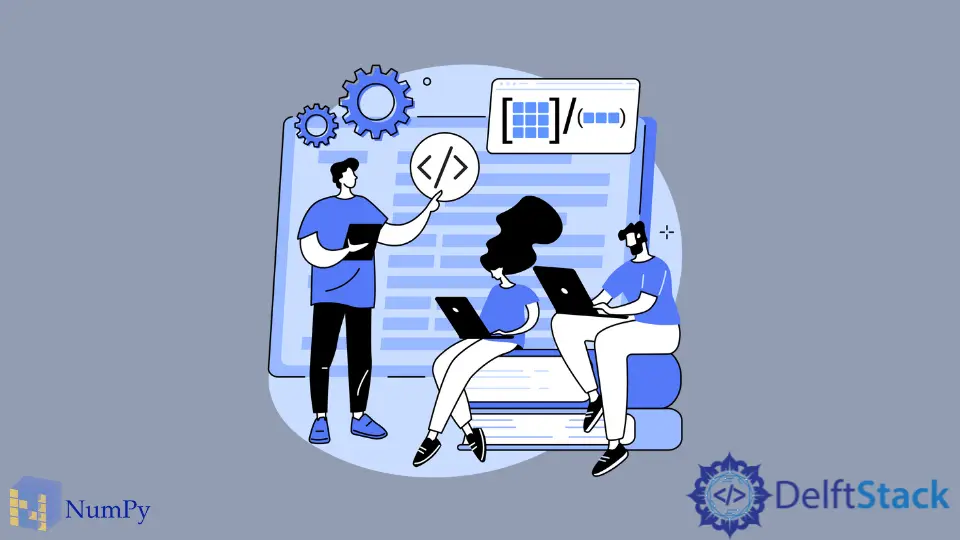
本教程将讨论在 NumPy 中将矩阵除以向量的方法。
在 NumPy 中用 Python 中的数组切片方法按向量划分矩阵
矩阵是一个二维数组,而向量只是一个一维数组。如果我们想将矩阵的元素除以每行中的向量元素,我们必须向向量添加一个新维度。我们可以使用 Python 中的数组切片方法为向量添加一个新维度。下面的代码示例向我们展示了如何使用 Python 中的数组切片方法将矩阵的每一行除以向量。
import numpy as np
matrix = np.array([[2, 2, 2], [4, 4, 4], [6, 6, 6]])
vector = np.array([2, 4, 6])
matrix = matrix / vector[:, None]
print(matrix)
输出:
[[1. 1. 1.]
[1. 1. 1.]
[1. 1. 1.]]
我们首先使用 np.array()
函数创建矩阵和向量。然后我们使用切片方法向向量添加了一个新轴。然后我们将矩阵除以数组并将结果保存在矩阵中。
在 NumPy 中用 NumPy 中的转置方法按向量划分矩阵
我们还可以转置矩阵以将矩阵的每一行除以每个向量元素。之后,我们可以转置结果以返回矩阵的先前方向。请参考以下代码示例。
import numpy as np
matrix = np.array([[2, 2, 2], [4, 4, 4], [6, 6, 6]])
vector = np.array([2, 4, 6])
matrix = (matrix.T / vector).T
print(matrix)
输出:
[[1. 1. 1.]
[1. 1. 1.]
[1. 1. 1.]]
在上面的代码中,我们对矩阵进行了转置并将其除以向量。之后,我们对结果进行转置并将其存储在 matrix
中。
在 NumPy 中使用 numpy.reshape()
函数按向量划分矩阵
这种方法背后的整个想法是我们必须首先将向量转换为二维数组。numpy.reshape()
函数可用于将向量转换为二维数组,其中每一行仅包含一个元素。然后我们可以轻松地将矩阵的每一行除以向量的每一行。
import numpy as np
matrix = np.array([[2, 2, 2], [4, 4, 4], [6, 6, 6]])
vector = np.array([2, 4, 6])
matrix = matrix / vector.reshape((3, 1))
print(matrix)
输出:
[[1. 1. 1.]
[1. 1. 1.]
[1. 1. 1.]]
在上面的代码中,我们使用 np.reshape()
函数将 vector
转换为 2D 数组。之后,我们将 matrix
除以 vector
并将结果存储在 matrix
中。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn