How to Remove Element From an Array in MongoDB
- MongoDB Remove Element From an Array
-
Use MongoDB
$pull
to Remove Element From an Array of Documents -
Use MongoDB
$pullAll
to Remove Element From an Array -
Use MongoDB
$pop
to Remove Element From an Array -
Use MongoDB
$unset
to Remove a Field Containing the Given Element -
Use MongoDB Positional Operator to Set Element to
null
Instead of Removing
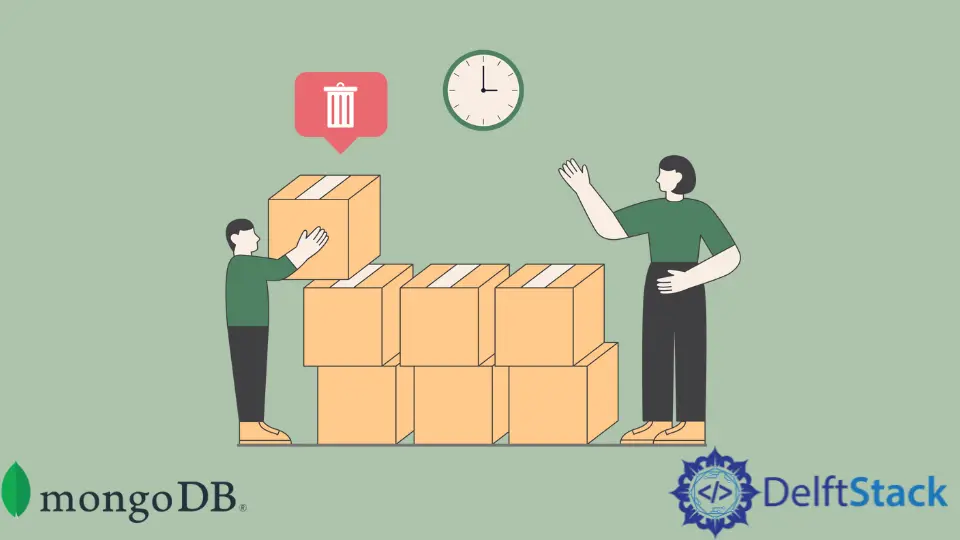
We can adopt different approaches depending on the project requirements. Today, we will learn how to use $pull
, $pullAll
, $pop
, $unset
, and the positional operator ($
) to remove an element from an array or array of documents while working in MongoDB.
MongoDB Remove Element From an Array
We must have a sample document(s) collection to practice all the mentioned ways. We will create a collection named collection
and insert one document only.
You may also use the following collection
containing one document.
Example Code:
// MongoDB version 5.0.8
> db.collection.insertMany([
{
contact: {
phone: [
{"type" : "UAN", "number" : "123456789"},
{"type" : "office", "number" : "987546321"},
{"type" : "personal", "number" : "852147963"}
]
},
services: ["Google", "Instagram", "Twitter", "Facebook"],
scores: [ 0, 1, 8, 17, 18, 8, 20, 10 ]
},
{
contact: {
phone: [
{"type" : "UAN", "number" : "456321879"},
{"type" : "office", "number" : "874596321"},
{"type" : "personal", "number" : "964785123"}
]
},
services: ["Google", "Instagram", "Twitter"],
scores: [ 1, 8, 2, 3, 8, 7, 1 ]
}
]);
If you do not have the collection named collection
, don’t worry; the query above will create that for you and populate it. Next, you may run db.collection.find().pretty()
to have a look at the inserted document.
Remember, you will not see the updated documents after executing the upcoming code examples. For that, you have to execute the db.collection.find().pretty()
command.
Use MongoDB $pull
to Remove Element From an Array of Documents
Example Code:
// MongoDB version 5.0.8
> db.collection.update(
{ _id : ObjectId("62aae796f27219958a489b89") },
{ $pull : { "contact.phone": { "number": "987546321" } } }
);
OUTPUT:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "UAN", "number" : "123456789" },
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Google", "Instagram", "Twitter", "Facebook" ],
"scores" : [ 0, 1, 8, 17, 18, 8, 20, 10 ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "UAN", "number" : "456321879" },
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Google", "Instagram", "Twitter" ],
"scores" : [ 1, 8, 2, 3, 8, 7, 1 ]
}
Here, we are using the $pull
operator to remove all the instances of a value/values from an existing array that matches the filter query or specified condition.
In the above code example, we are searching for a document where the _id
is equal to ObjectId("62aae796f27219958a489b89")
. Once the document matches the specified condition, we remove the instance from an existing array where the contact.phone.number
is equal to 987546321
.
Remember the following points while using the $pull
operator.
- If the given
value
that needs to be removed is an array, then the$pull
operator removes elements only from an array that satisfy the givenvalue
exactly (including order). - If the given
value
that needs to be removed is a document, then the$pull
operator removes elements only from an array that contains the exact fields & values. The fields’ ordering can differ here. - If the given
condition
and the elements of the array are embedded documents, then the$pull
operator will apply thecondition
as if every array element was a document in a collection. For more details on that, you may check this.
Now, we have another situation where we want to perform the remove
operation on all the documents in a collection. To do that, we use the following syntax using $pull
while {multi: true}
operates on all documents of the specified condition.
Example Code:
// MongoDB version 5.0.8
> db.collection.update({},
{ $pull: { "contact.phone": { "type": "UAN" } } },
{ multi: true }
);
OUTPUT:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Google", "Instagram", "Twitter", "Facebook" ],
"scores" : [ 0, 1, 8, 17, 18, 8, 20, 10 ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Google", "Instagram", "Twitter" ],
"scores" : [ 1, 8, 2, 3, 8, 7, 1 ]
}
To get the above output, we remove the array element from all the documents in a collection where the contact.phone.type
is equal to the "UAN"
.
Our next task is to remove elements from the scores
array where the values are greater than or equal to 2
and less than or equal to 6
( 2 <= value <= 6
). Execute the following query to do it for all the documents of the selected collection.
Example Code:
// MongoDB version 5.0.8
> db.collection.update(
{},
{ $pull : { scores : {$gte: 2, $lte: 6} } },
{ multi: true }
)
OUTPUT:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Google", "Instagram", "Twitter", "Facebook" ],
"scores" : [ 0, 1, 8, 17, 18, 8, 20, 10 ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Google", "Instagram", "Twitter" ],
"scores" : [ 1, 8, 8, 7, 1 ]
}
To practice with string-type values, we can do it the following way.
Example Code:
// MongoDB version 5.0.8
> db.collection.update(
{},
{ $pull: { "services": { $in: [ "Google", "Instagram" ] }, "scores": 1 } },
{ multi: true }
)
OUTPUT:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Twitter", "Facebook" ],
"scores" : [ 0, 8, 17, 18, 8, 20, 10 ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Twitter" ],
"scores" : [ 8, 8, 7 ]
}
For this code example, we use the $in
comparison operator to have an array of values and remove them from the services
array if any of them is present.
Further, we remove 1
from the scores
array if it is there while we are using the $pull
operator to perform all this remove operation.
Use MongoDB $pullAll
to Remove Element From an Array
Example Code:
// MongoDB version 5.0.8
> db.collection.update(
{ _id: ObjectId("62aae796f27219958a489b8a") },
{ $pullAll: { "scores": [8] } }
)
OUTPUT:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Twitter", "Facebook" ],
"scores" : [ 0, 8, 17, 18, 8, 20, 10 ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Twitter" ],
"scores" : [ 7 ]
}
For this code, we are using the $pullAll
operator to remove all the occurrences (instances) of the given value from an already existing array. For instance, if the number 8
occurs twice in the scores
array, both 8
values will be removed.
Like the $pull
operator, which removes the array elements by specifying the filter query, the $pullAll
deletes/removes the array elements that match the listed values.
We use the dot notation to handle embedded documents of the array.
Use MongoDB $pop
to Remove Element From an Array
We can use the $pop
operator if we are interested in removing the first or last element from an array. For this, we pass the -1
or 1
to the $pop
operator to remove an array element from first or last.
Example Code:
// MongoDB version 5.0.8
> db.collection.update(
{ _id: ObjectId("62aae796f27219958a489b89") },
{ $pop: { "scores": -1 } }
)
OUTPUT:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Twitter", "Facebook" ],
"scores" : [ 8, 17, 18, 8, 20, 10 ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Twitter" ],
"scores" : [ 7 ]
}
Use MongoDB $unset
to Remove a Field Containing the Given Element
Example Code:
// MongoDB version 5.0.8
> db.collection.update(
{ _id : ObjectId("62aae796f27219958a489b89") },
{ $unset : {"scores" : 8 }}
)
OUTPUT:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Twitter", "Facebook" ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Twitter" ],
"scores" : [ 7 ]
}
Here, we use the $unset
operator to remove a specific field that contains the given value. For instance, we are removing the scores
field that matches the filter query and contains 8
as an array element.
Remember that when we use the $unset
operator with a positional operator ($
), it does not remove the matching element but sets it to null
. See the following section to practice that.
Use MongoDB Positional Operator to Set Element to null
Instead of Removing
Example Code:
// MongoDB version 5.0.8
> db.collection.update(
{"services": "Facebook"},
{ $unset: {"services.$" : 1}}
)
OUTPUT:
{
"_id" : ObjectId("62aae796f27219958a489b89"),
"contact" : {
"phone" : [
{ "type" : "personal", "number" : "852147963" }
]
},
"services" : [ "Twitter", null ]
}
{
"_id" : ObjectId("62aae796f27219958a489b8a"),
"contact" : {
"phone" : [
{ "type" : "office", "number" : "874596321" },
{ "type" : "personal", "number" : "964785123" }
]
},
"services" : [ "Twitter" ],
"scores" : [ 7 ]
}
This code snippet uses $unset
with the positional operator to set the element of the array to null
instead of removing it. We use $
to update the first element of the array where the value is Facebook
in the services
array.
This approach can be used when we don’t know the position of an element of the array. On the other hand, we can also use the $[]
(all positional operators) if we want to update all the elements in the given array field.
Related Article - MongoDB Array
- How to Find a Specific Document With Array in MongoDB
- How to Add Element to Array in MongoDB
- How to Use forEach() to Update an Array Field in MongoDB Shell