How to Use forEach() to Update an Array Field in MongoDB Shell
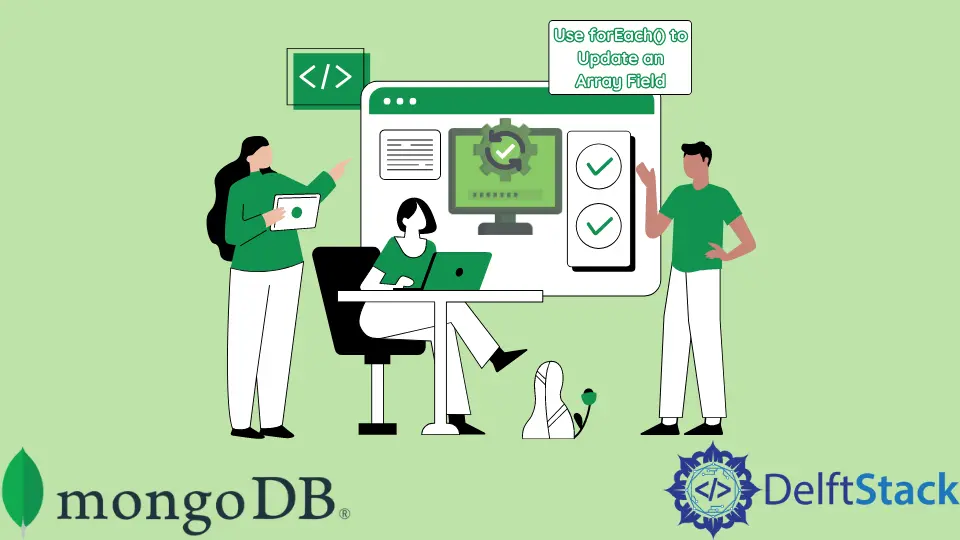
Today, we’ll learn how to use forEach()
to update an array field while using the MongoDB shell.
Use forEach()
to Update an Array Field in MongoDB Shell
To use forEach()
, let’s prepare a sample collection named collection
containing two documents. You may also use the following queries to continue.
Example Code:
> use updateArrayField
> db.createCollection('collection');
> db.collection.insertMany([
{
"name": "Mehvish",
"gender": "Female",
"fields" : [
{ "_id" : 1, "items" : [ 1,3,4,5,6,7 ] }
]
},
{
"name": "Thomas Christopher",
"gender": "Male",
"fields" : [
{ "_id" : 1, "items" : [ 1,3,4,5,6,7 ] }
]
}
]);
Next, we use these documents to update the array field named fields.items
from
"items" : [ 1,3,4,5,6,7]
to
"items" : [
{item: 1, key: 0},
{item: 3, key: 0},
{item: 4, key: 0},
{item: 5, key: 0},
{item: 6, key: 0},
{item: 7, key: 0}
]
For that, we can use a nested forEach()
, as given below.
Example Code:
> var table = db.collection.find();
> table.forEach(function( oneRow ) {
var newFields = [];
oneRow.fields.forEach( function( oneField ){
var newItems = [];
oneField.items.forEach( function( item ){
var aNewItem = { item: parseInt(item), key: 0 };
newItems.push( aNewItem );
} );
newFields.push({ _id: oneField._id, items: newItems });
} )
db.collection.update(
{ _id: oneRow._id },
{ "$set": { "fields": newFields } }
);
});
Execute the following query on the mongo shell to see the updated documents.
Example Code:
> db.collection.find()
OUTPUT:
{
"_id" : ObjectId("62a708054eb9c63e48daeba4"),
"name" : "Mehvish",
"gender" : "Female",
"fields" : [
{
"_id" : 1,
"items" : [
{ "item" : 1, "key" : 0 },
{ "item" : 3, "key" : 0 },
{ "item" : 4, "key" : 0 },
{ "item" : 5, "key" : 0 },
{ "item" : 6, "key" : 0 },
{ "item" : 7, "key" : 0 }
]
}
]
}
{
"_id" : ObjectId("62a708054eb9c63e48daeba5"),
"name" : "Thomas Christopher",
"gender" : "Male",
"fields" : [
{
"_id" : 1,
"items" : [
{ "item" : 1, "key" : 0 },
{ "item" : 3, "key" : 0 },
{ "item" : 4, "key" : 0 },
{ "item" : 5, "key" : 0 },
{ "item" : 6, "key" : 0 },
{ "item" : 7, "key" : 0 }
]
}
]
}
To get the above output, we read all data from the collection
and use forEach()
to iterate over every single document of the collection
. Next, we use another forEach()
to loop over every single field of the specified document.
Then, the third forEach()
is used to iterate over the values of the fields.items
field from the collection
. We use every value to form the desired update and save it into the aNewItem
variable, which is further inserted into the newItems
array using the push()
method.
After that, we make a document using the oneField._id
and newItems
array, which is pushed into the newFields
array that is further used to update the collection
.
Related Article - MongoDB Array
- How to Find a Specific Document With Array in MongoDB
- How to Add Element to Array in MongoDB
- How to Remove Element From an Array in MongoDB