How to Update Multiple Documents in MongoDB
-
the
updateMany()
Method in MongoDB -
the
$set
Operator in MongoDB - Create a Collection to Be Updated in MongoDB
-
Use the
updateMany()
Method to Update Multiple Documents in MongoDB -
Use the
updateMany()
Method to Update Embedded Documents in MongoDB -
Use the
updateMany()
Method to Update Array Elements in MongoDB - Conclusion
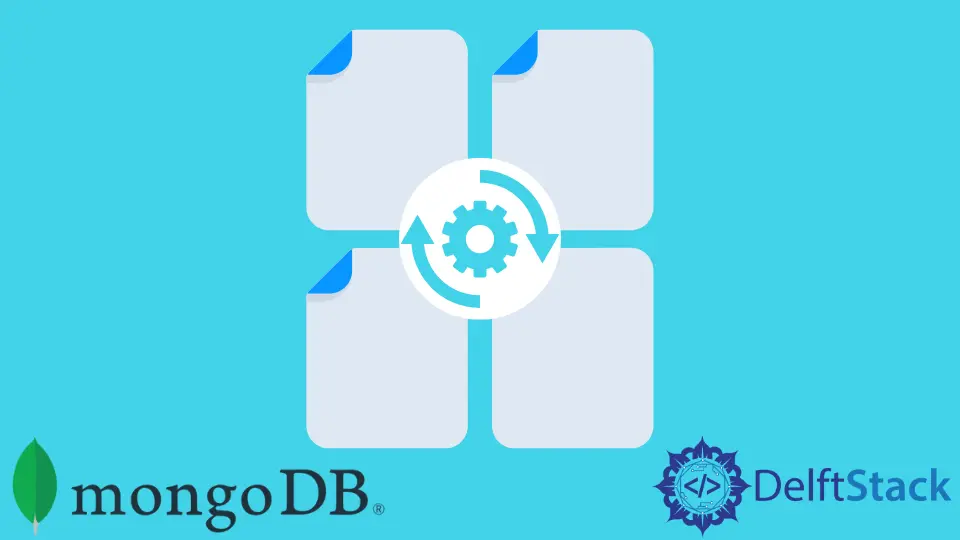
This article will discuss how to update multiple documents in MongoDB efficiently.
the updateMany()
Method in MongoDB
Using the db.collection.updateMany()
method in MongoDB, you can update several documents in a collection. This method changes all of the collection’s documents that match the specified filter.
Below are some points to note when using this method.
- This approach accepts only documents with updated operator expressions.
- The
updateMany()
method can be used in multi-document transactions. - When you update your documents, the value of the
_id
field does not change. - It also adds new fields to the documents.
Syntax:
db.collection.updateMany(
<filter>,
<update>,
{
upsert: <boolean>,
writeConcern: <document>,
collation: <document>,
arrayFilters: [ <filterdocument1>, <filterdocument2>... ],
hint: <document|string>
}
)
Parameters:
filter
: This is the method’s first parameter. It specifies the update’s selection criteria.
This parameter’s type is a document. If it contains an empty record, i.e., {}
, then this method will update all the documents of the collection with the updated document.
-
update
: This is the method’s second parameter. This parameter type is either document or pipeline, and it contains changes to the documents.It can be an updated document (containing edit operator expressions) or an aggregation pipeline (comprising just aggregation steps, such as
$addFields
,$project
, and$replaceRoot
).
Optional Parameters:
-
upsert
: This parameter’s value is either true or false. Assume this parameter’s value istrue
.The procedure will update all documents that meet the specified criteria in that scenario. This method will input a new document (i.e., an updated document) in the collection if any of the documents in the collection do not fit the supplied filter.
The default value for this option is
false
, and its type is boolean. -
writeConcern
: This is the only option when you don’t want to utilize the default write concern. This option has the parameter type document. -
collation
: It describes how the collation will be used in operations. It also allows users to establish language-specific string comparison criteria, such as lowercase and accent mark rules.This option has the parameter type of document.
-
arrayFilters
: It’s an array of filter documents that tell you which array elements to change for an array field update. This parameter has the type of array. -
hint
: It’s a document or field that indicates the index that the filter will use to support it. It can accept an index specification document or an index name string, and it will return an error if you select an index that does not exist. -
Return
: This method will return a document with a boolean value oftrue
(ifwriteconcern
is enabled) orfalse
(ifwriteconcern
is disabled), amatchedCount
value of the number of matched documents, amodifiedCount
value of the number of modified records, and aupsertedId
value of the upserted document’s_id
.
the $set
Operator in MongoDB
You typically use the $set
operator to form the update argument. It replaces a field’s value with a value given by the user.
Syntax:
{ $set: { <field1>: <value1>, <field2>: <value2>, ...}}
Suppose the field
doesn’t exist. Then, the $set
operator will create a new field with the supplied value in the record.
If you use the dot notation to define the field,
for example, embededDoc.field,
and the field
does not exist in the database, the $set
operator creates the embedded document.
Create a Collection to Be Updated in MongoDB
You can use the following collection called products
.
db={
"products": [
{
"_id": 1,
"name": "xPhone",
"price": 799,
"specs": {
"ram": 4,
"screen": 6.5,
"cpu": 2.66
},
"color": [
"white",
"black"
],
"storage": [
64,
128,
256
]
},
{
"_id": 2,
"name": "xTablet",
"price": 899,
"specs": {
"ram": 16,
"screen": 9.5,
"cpu": 3.66
},
"color": [
"white",
"black",
"purple"
],
"storage": [
128,
256,
512
]
},
{
"_id": 3,
"name": "SmartTablet",
"price": 899,
"specs": {
"ram": 12,
"screen": 9.7,
"cpu": 3.66
},
"color": [
"blue"
],
"storage": [
16,
64,
128
]
},
{
"_id": 4,
"name": "SmartPad",
"price": 699,
"specs": {
"ram": 8,
"screen": 9.7,
"cpu": 1.66
},
"color": [
"white",
"orange",
"gold",
"gray"
],
"storage": [
128,
256,
1024
]
},
{
"_id": 5,
"name": "SmartPhone",
"price": 599,
"specs": {
"ram": 4,
"screen": 5.7,
"cpu": 1.66
},
"color": [
"white",
"orange",
"gold",
"gray"
],
"storage": [
128,
256
]
}
]
}
Use the updateMany()
Method to Update Multiple Documents in MongoDB
The updateMany()
method is used in the following example to update records when the price field value is 899.
db.products.updateMany(
{ price: 899},
{ $set: { price: 895 }}
)
The filter
input in this query is {price: 899},
which specifies the documents to update. The change to be applied is {$set: { price: 895}}
which utilizes the set
operator to assign the price
field value to 895.
Output:
The matchedCount
field in this result document represents the number of matching records, whereas the modifiedCount
field stores the number of updated documents.
To verify the modification, use the find()
method to select the documents with the price
field value of 895,
as shown below:
db.products.find({
price: 895
}, {
name: 1,
price: 1
})
Output:
The image above shows that the price
field values have been successfully updated.
Use the updateMany()
Method to Update Embedded Documents in MongoDB
The following query employs the find()
method to discover documents with a price
field value greater than 700
.
db.products.find({
price: { $gt: 700}
}, {
name: 1,
price: 1,
spec: 1
})
Output:
The updateMany()
method is used in the spec
embedded documents of these documents for updating the values of the ram
, screen
, and cpu
fields:
db.products.updateMany({
price: { $gt: 700}
}, {
$set: {
"spec.ram": 32,
"spec.screen": 9.8,
"spec.cpu": 5.66
}
})
Output:
The result in the image indicates that the three documents had been updated successfully.
Use the updateMany()
Method to Update Array Elements in MongoDB
The updateMany()
method is used in the following example to update the first and second elements of the storage
array of documents where the _id
is 1
, 2
, and 3
.
db.products.updateMany({
_id: {
$in: [1, 2, 3]
}
}, {
$set: {
"storage.0": 16,
"storage.1": 32
}
})
Output:
The first and second components of the storage
array have been modified if you query the documents whose _id
is 1
, 2
, and 3
from the products
collection.
db.products.find(
{ _id: { $in: [1,2,3]}},
{ name: 1, storage: 1}
)
Output:
Conclusion
Through this tutorial article, you’ve learned how to use the updateMany()
function to update all of the records in a collection that meet a condition and use the $set
operator to replace a field’s value with a specific value.