更新 MongoDB 中的多個文件
-
MongoDB 中的
updateMany()
方法 -
MongoDB 中的
$set
運算子 - 在 MongoDB 中建立要更新的集合
-
使用
updateMany()
方法更新 MongoDB 中的多個文件 -
使用
updateMany()
方法更新 MongoDB 中的嵌入式文件 -
使用
updateMany()
方法更新 MongoDB 中的陣列元素 - まとめ
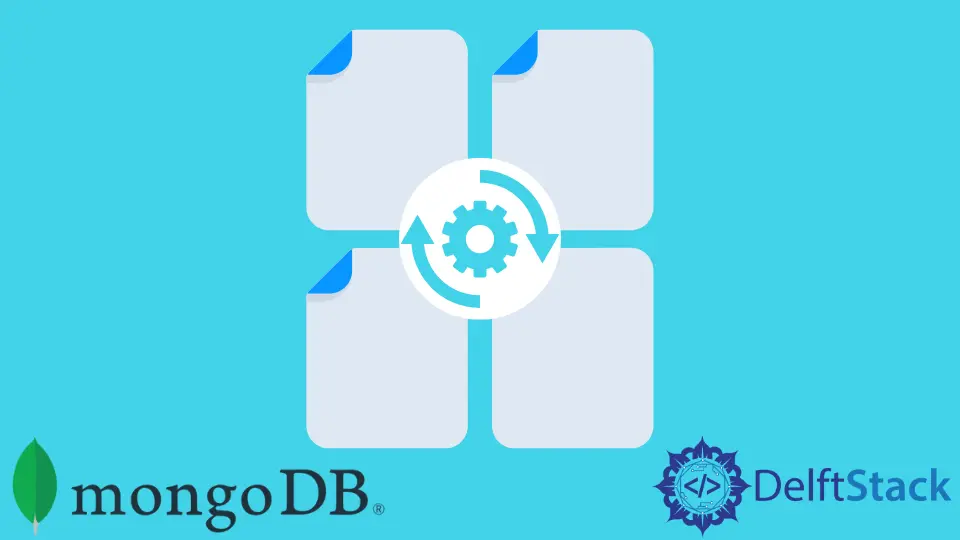
本文將討論如何高效地更新 MongoDB 中的多個文件。
MongoDB 中的 updateMany()
方法
使用 MongoDB 中的 db.collection.updateMany()
方法,你可以更新集合中的多個文件。此方法更改與指定過濾器匹配的所有集合文件。
以下是使用此方法時的一些注意事項。
- 此方法僅接受具有更新的運算子表示式的文件。
updateMany()
方法可用於多文件事務。- 當你更新文件時,
_id
欄位的值不會改變。 - 它還為文件新增了新欄位。
語法:
db.collection.updateMany(
<filter>,
<update>,
{
upsert: <boolean>,
writeConcern: <document>,
collation: <document>,
arrayFilters: [ <filterdocument1>, <filterdocument2>... ],
hint: <document|string>
}
)
引數:
-
filter
:這是方法的第一個引數。它指定更新的選擇標準。此引數的型別是文件。如果它包含一條空記錄,即
{}
,則此方法將使用更新後的文件更新集合的所有文件。 -
update
:這是方法的第二個引數。此引數型別是文件或管道,它包含對文件的更改。它可以是更新的文件(包含編輯運算子表示式)或聚合管道(僅包含聚合步驟,例如
$addFields
、$project
和$replaceRoot
)。
可選引數:
-
upsert
:此引數的值為真或假。假設此引數的值為true
。該過程將更新滿足該方案中指定標準的所有文件。如果集合中的任何文件不適合提供的過濾器,此方法將在集合中輸入新文件(即更新的文件)。
此選項的預設值為
false
,其型別為布林值。 -
writeConcern
:當你不想使用預設的寫入關注點時,這是唯一的選擇。此選項具有引數型別文件。 -
collation
:它描述排序規則將如何在操作中使用。它還允許使用者建立特定於語言的字串比較標準,例如小寫和重音標記規則。此選項具有文件的引數型別。
-
arrayFilters
:這是一個過濾器文件陣列,告訴你要更改哪些陣列元素以進行陣列欄位更新。該引數具有陣列型別。 -
hint
:它是一個文件或欄位,指示過濾器將用於支援它的索引。它可以接受索引規範文件或索引名稱字串,如果你選擇不存在的索引,它將返回錯誤。 -
Return
: 此方法將返回一個布林值為true
(如果writeconcern
啟用)或false
(如果writeconcern
禁用)的文件,匹配文件數量的matchedCount
值,modifiedCount
修改記錄數的值,以及更新插入文件的_id
的upsertedId
值。
MongoDB 中的 $set
運算子
你通常使用 $set
運算子來形成更新引數。它用使用者給定的值替換欄位的值。
語法:
{ $set: { <field1>: <value1>, <field2>: <value2>, ...}}
假設 field
不存在。然後,$set
運算子將使用記錄中提供的值建立一個新欄位。
如果你使用點符號定義 field
,例如 embededDoc.field,
,並且資料庫中不存在 field
,$set
運算子會建立嵌入文件。
在 MongoDB 中建立要更新的集合
你可以使用以下名為 products
的集合。
db={
"products": [
{
"_id": 1,
"name": "xPhone",
"price": 799,
"specs": {
"ram": 4,
"screen": 6.5,
"cpu": 2.66
},
"color": [
"white",
"black"
],
"storage": [
64,
128,
256
]
},
{
"_id": 2,
"name": "xTablet",
"price": 899,
"specs": {
"ram": 16,
"screen": 9.5,
"cpu": 3.66
},
"color": [
"white",
"black",
"purple"
],
"storage": [
128,
256,
512
]
},
{
"_id": 3,
"name": "SmartTablet",
"price": 899,
"specs": {
"ram": 12,
"screen": 9.7,
"cpu": 3.66
},
"color": [
"blue"
],
"storage": [
16,
64,
128
]
},
{
"_id": 4,
"name": "SmartPad",
"price": 699,
"specs": {
"ram": 8,
"screen": 9.7,
"cpu": 1.66
},
"color": [
"white",
"orange",
"gold",
"gray"
],
"storage": [
128,
256,
1024
]
},
{
"_id": 5,
"name": "SmartPhone",
"price": 599,
"specs": {
"ram": 4,
"screen": 5.7,
"cpu": 1.66
},
"color": [
"white",
"orange",
"gold",
"gray"
],
"storage": [
128,
256
]
}
]
}
使用 updateMany()
方法更新 MongoDB 中的多個文件
以下示例中使用 updateMany()
方法在價格欄位值為 899.
時更新記錄。
db.products.updateMany(
{ price: 899},
{ $set: { price: 895 }}
)
此查詢中的 filter
輸入是 {price: 899},
,它指定要更新的文件。要應用的更改是 {$set: { price: 895}}
,它利用 set
運算子將 price
欄位值分配給 895.
。
輸出:
該結果文件中的 matchedCount
欄位表示匹配記錄的數量,而 modifiedCount
欄位儲存更新文件的數量。
為了驗證修改,使用 find()
方法選擇 price
欄位值為 895,
的文件,如下所示:
db.products.find({
price: 895
}, {
name: 1,
price: 1
})
輸出:
上圖顯示 price
欄位值已成功更新。
使用 updateMany()
方法更新 MongoDB 中的嵌入式文件
以下查詢使用 find()
方法來發現 price
欄位值大於 700
的文件。
db.products.find({
price: { $gt: 700}
}, {
name: 1,
price: 1,
spec: 1
})
輸出:
updateMany()
方法用於這些文件的 spec
嵌入式文件中,用於更新 ram
、screen
和 cpu
欄位的值:
db.products.updateMany({
price: { $gt: 700}
}, {
$set: {
"spec.ram": 32,
"spec.screen": 9.8,
"spec.cpu": 5.66
}
})
輸出:
影象中的結果表明三個文件已成功更新。
使用 updateMany()
方法更新 MongoDB 中的陣列元素
updateMany()
方法在以下示例中用於更新 storage
文件陣列的第一個和第二個元素,其中 _id
為 1
、2
和 3
。
db.products.updateMany({
_id: {
$in: [1, 2, 3]
}
}, {
$set: {
"storage.0": 16,
"storage.1": 32
}
})
輸出:
如果你從 products
集合中查詢 _id
為 1
、2
和 3
的文件,storage
陣列的第一個和第二個元件已被修改。
db.products.find(
{ _id: { $in: [1,2,3]}},
{ name: 1, storage: 1}
)
輸出:
まとめ
通過本教程文章,你學習瞭如何使用 updateMany()
函式更新集合中滿足條件的所有記錄,並使用 $set
運算子將欄位的值替換為特定值。