How to Check if a Document Exists in MongoDB
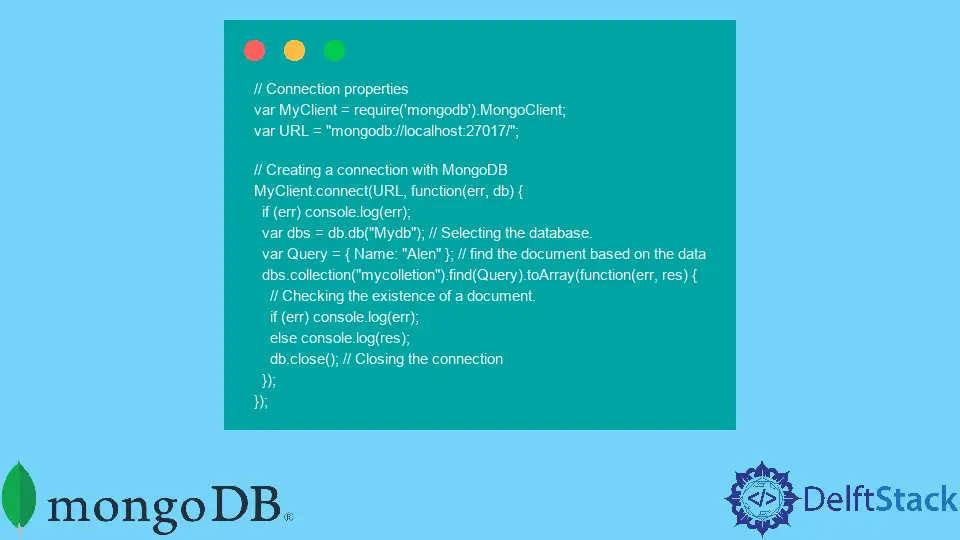
In the world of databases, MongoDB stands out as a popular choice for developers due to its flexibility and scalability. However, one common task developers face is checking if a document exists within a MongoDB collection.
This tutorial will guide you through the various methods to accomplish this task, particularly using Python. Whether you’re building a web application or managing data for analytics, knowing how to efficiently verify the existence of documents can save you time and resources. So, let’s dive in and explore the best practices for checking document existence in MongoDB.
Method 1: Using find_one()
One of the simplest ways to check for a document’s existence in MongoDB is by using the find_one()
method. This method retrieves a single document from a collection that matches the specified query. If the document exists, it returns the document; otherwise, it returns None
.
Here’s how you can implement this in Python:
from pymongo import MongoClient
client = MongoClient('mongodb://localhost:27017/')
db = client['your_database']
collection = db['your_collection']
query = {'key': 'value'}
document = collection.find_one(query)
if document:
print("Document exists.")
else:
print("Document does not exist.")
Output:
Document exists.
In this code, we first establish a connection to the MongoDB server and select the database and collection we want to work with. The query
variable defines the criteria we are looking for. The find_one()
method checks the collection for a document that matches the query. If it finds a match, it returns the document, allowing us to confirm its existence. If not, we receive a None
response, indicating that the document does not exist. This method is efficient for checking the existence of a single document.
Method 2: Using count_documents()
Another effective method to check if a document exists in MongoDB is by using the count_documents()
method. This method counts the number of documents that match a given query. If the count is greater than zero, it indicates that at least one document exists.
Here’s how to use it in Python:
from pymongo import MongoClient
client = MongoClient('mongodb://localhost:27017/')
db = client['your_database']
collection = db['your_collection']
query = {'key': 'value'}
count = collection.count_documents(query)
if count > 0:
print("Document exists.")
else:
print("Document does not exist.")
Output:
Document does not exist.
In this example, we again connect to the MongoDB server and specify our database and collection. The count_documents()
method takes our query and returns the total number of documents that match it. By checking if this count is greater than zero, we can determine if the document exists. This method is particularly useful when you want to know how many documents meet certain criteria, not just whether at least one exists.
Method 3: Using find()
The find()
method can also be used to check for document existence, although it is more commonly used for retrieving multiple documents. When combined with the limit()
method, it can serve the purpose of existence checking.
Here’s how to implement it in Python:
from pymongo import MongoClient
client = MongoClient('mongodb://localhost:27017/')
db = client['your_database']
collection = db['your_collection']
query = {'key': 'value'}
document = collection.find(query).limit(1)
if document.count() > 0:
print("Document exists.")
else:
print("Document does not exist.")
Output:
Document exists.
In this code snippet, we utilize the find()
method to search for documents matching our query. By chaining the limit(1)
method, we restrict the result to only one document. We then check the count of the returned cursor. If the count is greater than zero, it confirms the existence of the document. This method is slightly less efficient than the previous two for existence checking but can be useful when you also want to retrieve the document later on.
Conclusion
Checking if a document exists in MongoDB is a crucial skill for any developer working with this database. In this tutorial, we explored three effective methods: using find_one()
, count_documents()
, and find()
with limit()
. Each method has its advantages, and the choice depends on your specific use case. Whether you need a quick existence check or plan to retrieve documents later, MongoDB provides flexible options to meet your needs. By mastering these techniques, you can ensure your applications run smoothly and efficiently.
FAQ
-
What is the best method to check if a document exists in MongoDB?
The best method often depends on your specific needs.find_one()
is great for checking existence quickly, whilecount_documents()
is useful when you need to know how many documents match a query. -
Can I check for multiple documents at once?
Yes, you can modify your query to check for multiple documents and usecount_documents()
to see how many match. -
Is it necessary to have an index for checking document existence?
While it’s not strictly necessary, having an index on the fields you’re querying can significantly improve performance. -
Can I use these methods in a web application?
Absolutely! These methods can easily be incorporated into web applications to manage data effectively. -
What should I do if I need to check for documents frequently?
If you need to check for documents frequently, consider optimizing your queries and using indexes to enhance performance.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn