How to Add Element to Array in MongoDB
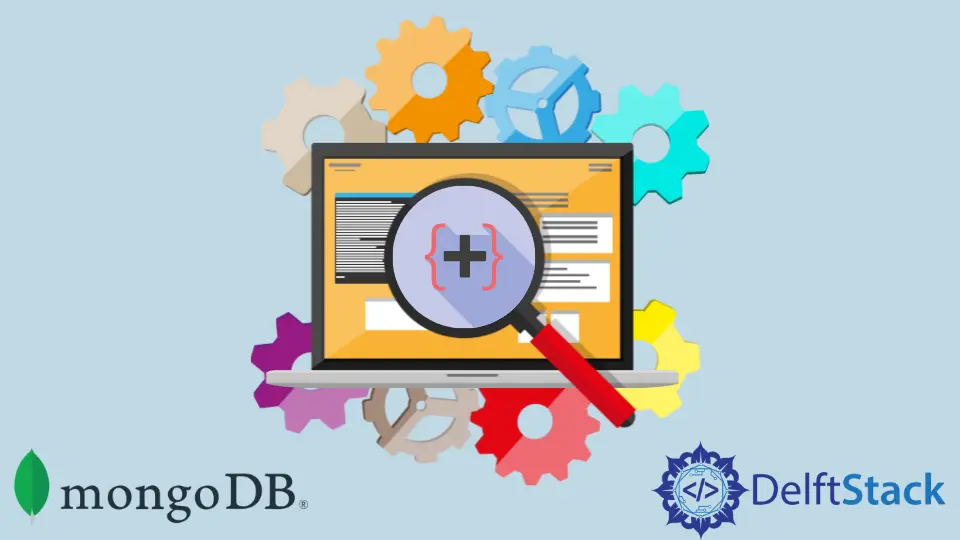
This article will look at many methods to add to an array in MongoDB.
Add to Array in MongoDB
Use the $push
Operator to Add Value to an Array
The $push
operator is one of the various array update operators MongoDB offers to update the contents of array fields in documents. The $push
operator can add a specific value to an array.
The $push
operator adds items to the end of the array. This action will fail if the field specified by the $push
operator is not an array.
Syntax:
{
$push: {
<field1>: <value1>,
<field2>: <value2>,
...
}
}
In this case, the field
can be specified using arrays, embedded/nested documents, or dot notation. Depending on your needs, you can combine this operator with methods like update()
, findAndModify()
, etc.
The $push
operator will add the array field with the value as its elements if the provided field is not already in the document.
The entire array will be added as a single element if the value of the $push
operator is an array. Additionally, you may use the $each
modifier and the $push
operator if you wish to add each value component independently.
Consider the following example to help you better understand the prior idea.
db.employees.update(
{name: "John Doe"},
{$push: {'professional_skills.$.technical': { skill: "C++", exp: '1' }}}
)
In the above example, we are changing the user "John Doe"
in the employees
collection. The array of professional_skills
contains the technical skills, communication skills, etc. is what we are storing in this place.
We are pushing an employee’s new technical expertise into technical skill by integrating their experience as an object. Run the above line of code in MongoShell, which is compatible with MongoDB.
Output:
{
"_id" : ObjectId("54f612b6029b47919a90cesd"),
"email" : "johndoe@exampledomain.com",
"name" : "John Doe",
"country" : "USA",
professional_skills: [
{
technical:[
{ skill:'javascript', exp: 4},
{ skill:'c', exp: 3}
]
},
]
}
Use the $addToSet
Operator to Add Value to an Array
When adding a value to an array, the $addToSet
operator checks to see if the value already exists in the array; otherwise, it does nothing. This operator does not add duplicate items to the array and does not affect duplicate items already there.
The values’ order is irrelevant in this situation.
Syntax:
{
$addToSet: {
<field1>: <value1>,
<field2>: <value2>,
...
}
}
In this case, the field
can be specified using arrays, embedded/nested documents, or dot notation. Depending on your needs, you can combine this operator with methods like update()
, findAndModify()
, etc.
Fields other than arrays cannot be used with this operator. The $addToSet
operator generates an array field in the document with values or items if the requested field does not exist.
Consider the following example to help you better understand the prior idea.
db.employees.update(
{name: "John Doe"},
{$addToSet: {'professional_skills.$.technical': { skill: "C++", exp: '1' }}}
)
In the above example, we are changing the user "John Doe"
in the employees
collection. The array of professional_skills
contains the technical skills, communication skills, etc. is what we are storing in this place.
We are pushing an employee’s new technical expertise into technical skill by integrating their experience as an object. The primary difference between them is that $addToSet
will add the element if it does not already exist, whereas $push
will append the new element to the array or list.
Run the above line of code in MongoShell, which is compatible with MongoDB.
Output:
{
"_id" : ObjectId("54f612b6029b47919a90cesd"),
"email" : "johndoe@exampledomain.com",
"name" : "John Doe",
"country" : "USA",
professional_skills: [
{
technical:[
{ skill:'javascript', exp: 4},
{ skill:'c', exp: 3}
]
},
]
}
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn