Color Cycle in Matplotlib
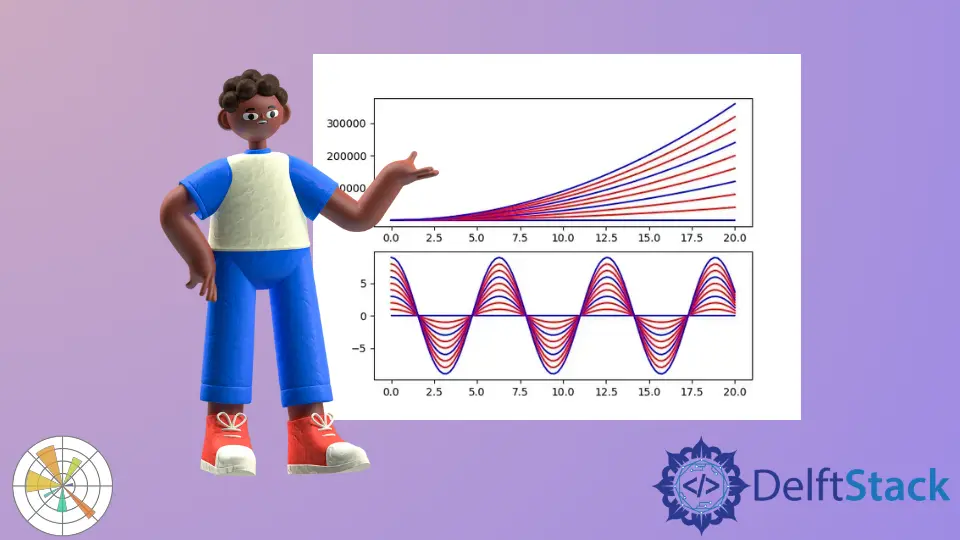
In this tutorial, we will be introduced to the color cycle and see how we can get and set the color property with the help of the color cycle in matplotlib.
Use the Color Cycle to Get and Set the Color Property in Matplotlib
When we work with a massive amount of data, we plot multiple lines, so we need to display a specific color plot. But the Matplotlib displays with its particular color track, so how do we change it?
According to the Matplotlib documentation, we cannot use the user-facing
(a.k.a public
) to access the underlying iterator. We will only be able to access it through private
methods.
However, we will not be able to get the state of an iterator without changing it.
We can set the color or property cycle in different ways. However, while working with the public-facing
method, we cannot access the iterable.
Still, we can access it by creating an object of axes and using the _get_lines
helper class instance. ax._get_lines
is confusing, but the behind-the-scenes machinery allows the plot command to process all odd and varied ways that the plot can be called.
Among other things, it is what keeps track of what colors to assign automatically. Similarly, the ax._get_patches_for_fill
method controls cycling through default fill colors and patch properties.
We have a property for working with lines in the color cycle iterable called ax._get_lines.color_cycle
and the ax._get_patches_for_fill.color_cycle
for working with patches.
Matplotlib 1.5 and the later versions have changed to use the Cycler
library. We can use the iterable called prop_cycler
instead of color_cycle
, a dict
of properties, or only color in our program.
But we can not see the state of an iterator since we can get the following item easily using the bare iterator object.
next_color = next(color_cycle)
next_color
specifies the next color we want to display in our plot. By design, we will not be able to get the state of an iterator without changing it.
In Matplotlib 1.5 or the later versions, we can access the cycler
object to use in its current state in our program. Since the cycler
object is not accessible (publicly or privately), only the itertools
.
However, the cycle instance created from the cycler
object is accessible. So, there is no way to get to the color or property cycler
; hence, we use fill_between
.
Instead, we can match the color of the previously plotted item. Rather than determining the color or property, setting the color of our new item is best on plot properties.
Example 1:
import numpy as np
import matplotlib.pyplot as plot
def custom_plot(x, y, **kwargs):
ax = kwargs.pop("ax", plot.gca())
(base_line,) = ax.plot(x, y, **kwargs)
ax.fill_between(x, 0.9 * y, 1.1 * y, facecolor=base_line.get_color(), alpha=0.5)
x = np.linspace(0, 1, 10)
custom_plot(x, x)
custom_plot(x, x * 2)
custom_plot(x, x - x, color="yellow", lw=3)
plot.show()
Output:
The following example allows us to set the default color in our plot while working with the color cycle.
Example 2:
import numpy as np
import matplotlib.pyplot as plot
import matplotlib as mpl
# Takes key value pair to set default cycle color
mpl.rcParams["axes.prop_cycle"] = mpl.cycler(color=["b", "r", "r"])
x1 = np.linspace(0, 20, 100)
fig, axes = plot.subplots(nrows=2)
for i in range(10):
axes[0].plot(x1, i * (x1 * 10) ** 2) # create a plot on zero axis
for j in range(10):
axes[1].plot(x1, j * np.cos(x1)) # # create second plot on 1 axis
plot.show()
Output:
Click here to read more about the color cycle in Matplotlib.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn