MATLAB Transpose
Ammar Ali
Apr 24, 2021
MATLAB
MATLAB Matrix
-
Calculate the Transpose of a Matrix Using the
transpose()
Function in MATLAB -
Calculate the Complex Conjugate Transpose of a Matrix Using the
ctranspose()
Function in MATLAB
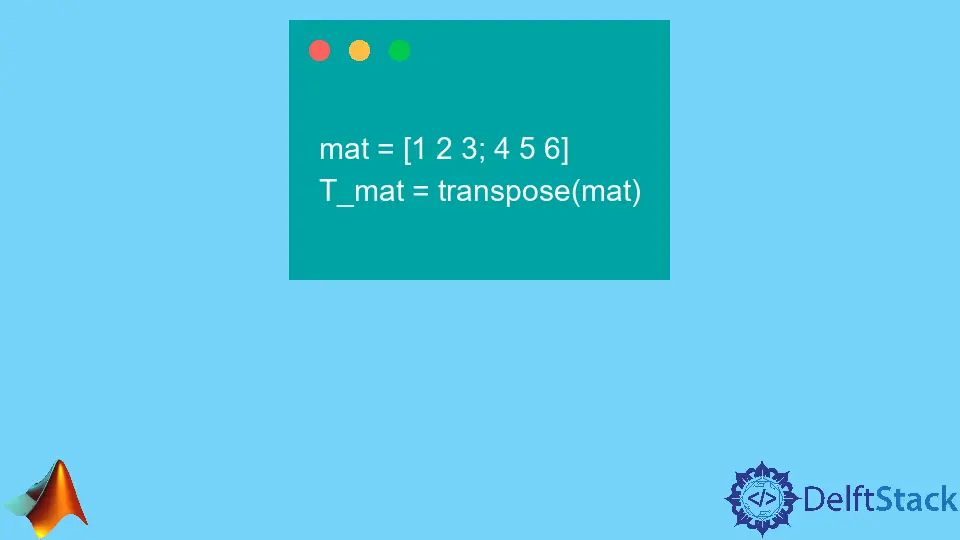
In this tutorial, we will discuss how to take the transpose of a matrix using the transpose()
and ctranspose()
function in MATLAB.
Calculate the Transpose of a Matrix Using the transpose()
Function in MATLAB
The transpose()
function is used to take the transpose of a vector or a matrix in MATLAB. You can also use the .'
operator instead of this function which performs the same as the transpose()
function. For example, let’s take the transpose of a matrix using the transpose()
function. See the code below.
mat = [1 2 3; 4 5 6]
T_mat = transpose(mat)
Output:
mat =
1 2 3
4 5 6
T_mat =
1 4
2 5
3 6
As you can see in the output, the second matrix is the transpose to of the first matrix. You can also use the .'
operator to take the transpose of a given matrix. For example, see the code below.
T_mat = mat.'