How to Get the Size of Vector in Matlab
-
Get the Size of a Vector in MATLAB Using the
size()
Function -
Get the Size of a Vector in MATLAB Using the
length()
Function -
Get the Size of a Vector in MATLAB Using the
numel()
Function - Conclusion
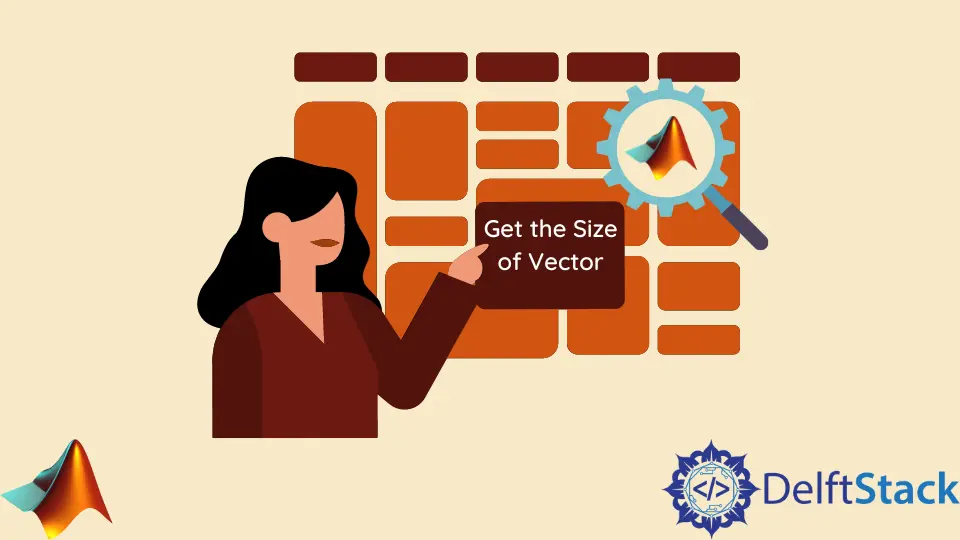
Understanding the size and the number of elements within a vector is fundamental for effective data analysis and manipulation in MATLAB.
In this article, we will explore various methods to achieve this goal using the size()
, length()
, and numel()
functions. Each function provides unique insights into the structure of vectors, offering versatility in different scenarios.
Get the Size of a Vector in MATLAB Using the size()
Function
The size()
function in MATLAB is a versatile tool that can be applied to arrays, matrices, and vectors to retrieve their dimensions.
When applied to a vector, the size()
function returns a two-element row vector containing the number of rows and columns, respectively. For a vector, the number of columns is always 1.
The basic syntax of the size()
function is as follows:
result = size(A);
Here, A
is the vector or matrix for which you want to determine the size. The function returns a 1-by-2 matrix, where the first element represents the number of rows, and the second element represents the number of columns.
Example 1: Basic Usage
Let’s start with a simple example to grasp the fundamental usage of the size()
function.
vector = [1 2 3 4];
result = size(vector)
In this example, we work with a basic vector vector
containing four elements. We use the size()
function to determine the dimensions of the vector.
When we execute the code, the result is a 1-by-2 matrix stored in the variable result
. The first element of this matrix represents the number of rows, which is 1, and the second element represents the number of columns, which is 4.
Therefore, the output indicates that our vector has 1 row and 4 columns.
Output:
result =
1 4
Example 2: Size of a 2D Matrix
Now, let’s explore the application of the size()
function on a 2D matrix.
matrix = [1 2 3; 4 5 6; 7 8 9];
result = size(matrix)
Here, we extend our understanding of the size()
function to a 2D matrix named matrix
. The matrix is defined with dimensions 3-by-3.
Upon applying the size()
function, the resulting matrix stored in the variable result
indicates that the matrix has 3 rows and 3 columns. This demonstrates the versatility of the function, as it seamlessly adapts to matrices with different dimensions.
Output:
result =
3 3
Example 3: Size of an Empty Vector
Consider the scenario of applying the size()
function to an empty vector.
empty_vector = [];
result = size(empty_vector)
In this scenario, we explore the behavior of the size()
function when applied to an empty vector empty_vector
. Upon execution, the output matrix result
reveals that the empty vector has 0 rows and 0 columns.
This showcases that the size()
function accurately handles scenarios where the input vector is devoid of elements.
result =
0 0
The size()
function is a versatile tool for extracting crucial information about the dimensions of vectors and matrices in MATLAB. Whether dealing with simple vectors or more complex matrices, the function provides a concise way to understand the structure of your data.
Get the Size of a Vector in MATLAB Using the length()
Function
While the size()
function is commonly used for this purpose, MATLAB also offers the length()
function as an alternative tool.
The length()
function in MATLAB is a simple yet powerful tool designed to provide the number of elements along the longest dimension of a vector or array. When applied to a vector, it directly returns the number of elements present in that vector.
The fundamental syntax of the length()
function is as follows:
result = length(A);
Here, A
represents the vector for which we aim to ascertain the number of elements. The function returns a single value, indicating the count of elements within the specified vector.
Example 1: Basic Usage
Let’s start with a straightforward example to grasp the basic usage of the length()
function.
vector = [1 2 3 4];
result = length(vector)
In this example, we define a vector vector
containing four elements. We apply the length()
function to this vector, and the result is stored in the variable result
.
The value held by result
signifies the count of elements in the vector, which, in this case, is 4. This demonstrates the primary purpose of the length()
function in providing a straightforward way to determine the number of elements in a given vector.
Output:
result =
4
Example 2: Assessing the Length of an Empty Vector
Now, let’s explore the behavior of the length()
function when applied to an empty vector.
empty_vector = [];
result = length(empty_vector);
In this scenario, an empty vector empty_vector
is defined. When the length()
function is employed, the resulting value stored in the variable result
represents the count of elements in the empty vector, which is 0.
This showcases the capability of the function to accurately handle scenarios where the input vector is devoid of elements.
Output:
result =
0
Example 3: Length of a 2D Matrix
While the length()
function is specifically designed for vectors, it’s important to understand its behavior when applied to a matrix.
matrix = [1 2 3; 4 5 6; 7 8 9];
result = length(matrix);
While the length()
function is specifically designed for vectors, it’s important to understand its behavior when applied to a matrix.
In this example, we define a 3-by-3 matrix named matrix
. However, when we apply the length()
function, it evaluates the longest dimension, which, in this case, is the number of columns.
Consequently, the result stored in the variable result
represents the count of columns in the matrix, emphasizing the specialized behavior of the length()
function.
Output:
result =
3
The length()
function in MATLAB provides a concise means of determining the count of elements within a vector. Its straightforward syntax and accurate handling of various scenarios make it a valuable tool for assessing the size of vectors.
Get the Size of a Vector in MATLAB Using the numel()
Function
MATLAB introduces another valuable tool to get the size and the count of elements in a vector, the numel()
function.
The numel()
function in MATLAB is specifically designed to provide the total number of elements in an array, regardless of its dimension. When applied to a vector, it directly returns the number of elements present in that vector.
The core syntax of the numel()
function is straightforward:
result = numel(A);
Here, A
represents the vector or matrix for which we want to ascertain the number of elements. The function returns a single value, representing the total count of elements within the specified array.
Example 1: Basic Usage
Let’s start with a fundamental example to grasp the basic usage of the numel()
function.
vector = [1 2 3 4];
result = numel(vector);
In this example, we define a vector vector
with four elements. The function is then applied to this vector, and the resulting value, stored in the variable result
, reflects the total count of elements in the vector.
In this case, the output is 4, showcasing the ability of the numel()
function to accurately assess the number of elements within a given vector.
Output:
result =
4
Example 2: Assessing the Number of Elements in a 2D Matrix
Now, let’s explore how the numel()
function handles a 2D matrix named matrix
.
matrix = [1 2 3; 4 5 6; 7 8 9];
result = numel(matrix);
Moving on to a more complex scenario, we explore how the numel()
function handles a 2D matrix named matrix
. This matrix is defined with dimensions 3-by-3.
When the numel()
function is applied, it calculates the total number of elements, considering both rows and columns. The resulting value stored in the variable result
indicates that the matrix has a total of 9 elements.
Output:
result =
9
This example highlights the versatility of the numel()
function in handling matrices with different dimensions.
Example 3: Assessing the Number of Elements in an Empty Vector
Now, let’s explore how the numel()
function behaves when applied to an empty vector.
empty_vector = [];
result = numel(empty_vector);
In this scenario, an empty vector empty_vector
is defined. Surprisingly, even for an empty vector, the numel()
function accurately determines that the count of elements is 0.
This demonstrates the consistency and reliability of the numel()
function across various data situations.
Output:
result =
0
The numel()
function in MATLAB provides a powerful tool for determining the total number of elements within vectors or matrices. Its versatility and accuracy make it an essential function for assessing the size and count of elements in MATLAB programming.
Conclusion
In MATLAB programming, understanding how to assess the size and number of elements in a vector is a fundamental skill. Throughout this article, we explored three functions—size()
, length()
, and numel()
—each offering a unique perspective on vector analysis.
The size()
function proves invaluable for obtaining the dimensions of a vector or matrix. It delivers a concise 1-by-2 matrix, providing a glance at the structure with the number of rows and columns.
On the other hand, the length()
function specializes in delivering the count of elements within a vector. Its simplicity and clarity make it a go-to choice for scenarios where knowing the length is paramount.
For a more comprehensive view, the numel()
function emerges as a versatile tool. Beyond merely counting elements, it extends its capability to matrices, providing a holistic assessment of the total number of elements within an array.