How to Trim Whitespace in Bash
-
Using the
xargs
Command to Trim Whitespace in Bash -
Using the
sed
Command to Trim Whitespace in Bash -
Using the
awk
Command to Trim Whitespace in Bash
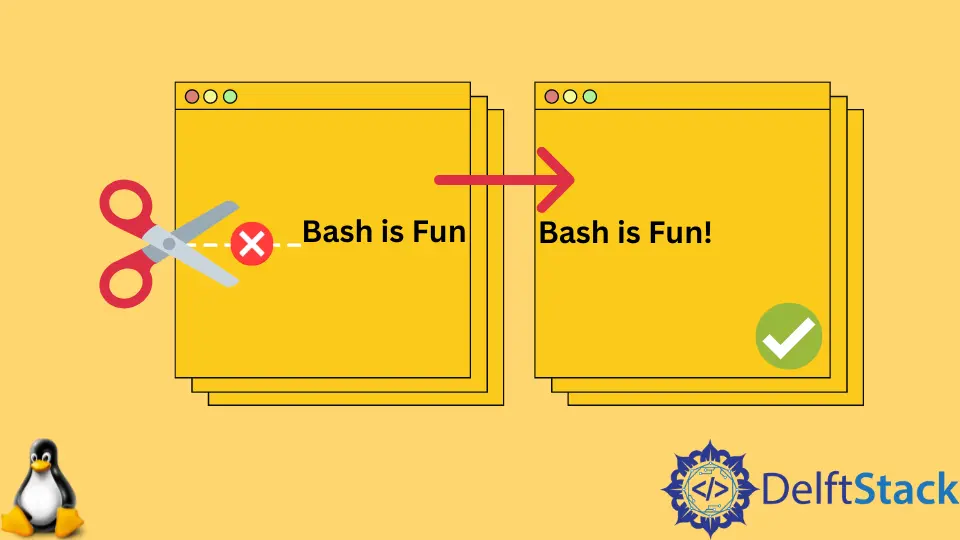
This tutorial shows trimming white spaces in bash using the xargs
command, the sed
command, and the awk
command.
Using the xargs
Command to Trim Whitespace in Bash
xargs
stands for eXtended ARGuments
. xargs
reads input from standard input and turns the input into arguments of a command. The xargs
command can also be used to remove external white spaces from a string in bash.
The example below illustrates using xargs
to trim whitespaces from a string in bash.
The xargs
command removes the spaces at the beginning and at the end of the string. It does not remove the internal whitespaces in the string. From the image above, the internal whitespace between ,
and world
has not been removed while the spaces at the beginning and the end of the string have been removed.
Using the sed
Command to Trim Whitespace in Bash
The sed
command can also be used to trim whitespaces at the beginning and the end of a string in bash. The script below uses the sed
command to trim trailing and leading whitespaces from a string in bash.
text=" Bash is Fun "
#print the original text
echo "$text"
#remove whitespaces
var=`echo $text | sed 's/ *$//g'`
echo "Hi there $var!"
Running the script displays the following output.
Bash is Fun
Hi there Bash is Fun!
Using the awk
Command to Trim Whitespace in Bash
The awk
command is also used to trim the whitespace of a file or string in bash. Using awk
, we can trim whitespaces at the beginning of a string, and we can trim white spaces at the end of the string, or both.
Let us write a bash script that uses awk
to trim whitespaces at the beginning of a string. This (/^[ \t]+/,"")
, tells the awk
command to replace the whitespace at the beginning of the text with nothing,""
.
text=" Bash is Fun "
#print the original text
echo "$text"
#print the new text after trimming the whitespace at the beginning
echo "$text" | awk '{gsub(/^[ \t]+/,""); print$0, "!"}'
Let us run the script.
bash trim_start.sh
The script produces the following output.
Bash is Fun
Bash is Fun !
From the output, we can see that the whitespace at the beginning of the string has been removed.
Let us write a bash script that removes the whitespace at the end of the string.
text=" Bash is Fun "
#print the original text
echo "$text"
#print the new text after trimming the whitespace at the end
echo "$text" | awk '{gsub(/[ \t]+$/,""); print$0, "!"}'
Let us run the script.
bash trim_end.sh
Running the script produces the following output.
Bash is Fun
Bash is Fun !
Now, let us write a bash script that trims whitespaces both at the beginning and at the end of a bash script.
text=" Bash is Fun "
#print the original text
echo "$text"
#print the new text after trimming the whitespace at the start and end
echo "$text" | awk '{gsub(/^[ \t]+| [ \t]+$/,""); print$0, "!"}'
Let us run the script.
bash trim.sh
The script displays the following output to the standard output. The leading and trailing whitespaces have been removed from the string.
Bash is Fun
Bash is Fun !