How to Get Script Directory in Bash
- Using $0 and dirname to Get Script Directory in Bash
-
Using
BASH_SOURCE
Array to Get Script Directory in Bash -
Using
readlink
Command to Get Script Directory in Bash -
Using
realpath
Command to Get Script Directory in Bash - Conclusion
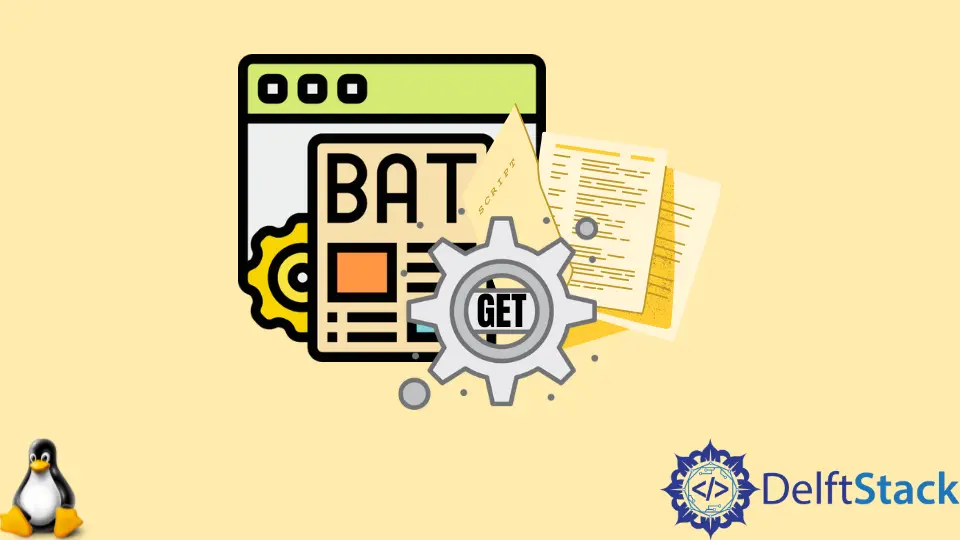
Determining the script directory in Bash is a key skill in Bash scripting, essential for navigating and manipulating directories effectively.
This article aims to demystify this concept, offering a deep dive into various methods for accurately finding the script’s location. Ideal for beginners and seasoned scripters alike, our guide provides detailed, user-friendly explanations to enhance your scripting journey in Bash.
Using $0 and dirname to Get Script Directory in Bash
Below guide explores a reliable method to determine the script’s directory using $0
and dirname
, a common requirement in many scripting scenarios.
Let’s begin with the complete working code:
#!/bin/bash
# Determine the script directory
script_dir="$(cd "$(dirname -- "$0")" && pwd)"
# Output the script directory
echo "Script directory: $script_dir"
This script efficiently finds the directory where the script resides. Now, let’s dissect each component to grasp their functions fully.
Step-by-Step Code Explanation
The script begins with the shebang line #!/bin/bash
, which is crucial for indicating that the script should be executed using the Bash shell. This ensures that no matter the environment in which the script is run, it is interpreted correctly.
Following the shebang, the script focuses on determining the script directory. The command script_dir="$(cd "$(dirname -- "$0")" && pwd)"
forms the heart of this operation. The variable $0
within this line is essential, as it holds the path to the script that’s being executed. The nature of this path, whether absolute or relative, depends on how the script is invoked.
The dirname
command is then employed to extract just the directory path from $0
, effectively ignoring the filename of the script itself. This step is crucial for isolating the directory. Next, the script uses the cd
command to change the current working directory to the one extracted by dirname
. This change of directory is executed within a subshell, denoted by $(...)
, ensuring that the main script’s working directory remains unaffected. This approach helps maintain the integrity of the script and prevents unexpected changes in behavior or environment.
Finally, the pwd
command comes into play. It prints the current working directory, which, thanks to the preceding cd
command, is now the directory where the script resides. The result of this operation, the script’s directory, is then stored in the variable script_dir
.
The last part of the script, echo "Script directory: $script_dir"
, serves to display the obtained script directory. This output is a straightforward confirmation of the script’s location, a vital piece of information for scripts that interact with files or directories relative to their own location.
When executed, this script will display the script’s directory. For instance, if the script is located in /path/to/script
, the output will be:
Script directory: /path/to/script
This output confirms the successful determination of the script’s directory, which is essential in scenarios where scripts need to access files relative to their own location or manage directory-dependent operations.
Using BASH_SOURCE
Array to Get Script Directory in Bash
The BASH_SOURCE
array offers a robust solution for this, providing a more precise way to identify the script’s location compared to traditional methods like $0
. This approach is particularly beneficial in scenarios involving source operations or when dealing with nested scripts. The following guide explains how to use the BASH_SOURCE
array to reliably obtain the script’s directory.
Let’s start by presenting the complete working code before delving into the step-by-step explanation:
#!/bin/bash
# Determine the script directory
script_dir="$(cd "$( dirname "${BASH_SOURCE[0]}" )" && pwd)"
# Display the script directory
echo "Script directory: $script_dir"
This script uses the BASH_SOURCE
array to find the directory of the currently executing script. The script begins with the shebang #!/bin/bash
, which is crucial for ensuring the script runs in the Bash environment, regardless of the user’s current shell.
The next line, script_dir="$(cd "$( dirname "${BASH_SOURCE[0]}" )" && pwd)"
, is the heart of the script. It uses ${BASH_SOURCE[0]}
, a special variable that contains the name of the script being executed. This is particularly useful in scenarios involving sourced scripts or multiple execution contexts, as it always refers to the script in which it is used.
The script employs dirname
to extract the directory from ${BASH_SOURCE[0]}
, discarding the filename. It then changes the current working directory to this script directory within a subshell, denoted by $(...)
. This approach ensures that the main script’s working directory remains unchanged, maintaining the script’s environmental stability.
The pwd
command is used within the subshell to print the current directory, now set to the script’s directory. The result of this operation is assigned to the script_dir
variable.
The final line, echo "Script directory: $script_dir"
, displays the determined script directory. This output is a straightforward confirmation of the script’s location, essential for scripts that depend on their directory for file operations or path-related tasks.
When executed, this script will display the script’s directory, providing a clear and accurate indication of its location. For instance:
Script directory: /path/to/script
This output verifies the successful retrieval of the script’s directory, illustrating the effectiveness of using the BASH_SOURCE
array in Bash scripting. This method is invaluable in ensuring script portability and reliability, especially in complex scripting scenarios.
Using readlink
Command to Get Script Directory in Bash
The readlink
command provides a robust solution to this challenge. It resolves the absolute path of a script, which is especially useful when the script is invoked through a symbolic link. This guide will walk you through using the readlink
command to reliably obtain the script’s directory.
Here’s the complete working code:
#!/bin/bash
# Get the directory of the script using readlink
SCRIPT_DIR=$(dirname "$(readlink -f "$0")")
# Display the script directory
echo "Script directory: $SCRIPT_DIR"
This script effectively utilizes the readlink
command to ascertain the directory of the currently executing Bash script. It starts with the shebang #!/bin/bash
, indicating that the script should be run in the Bash shell, a standard practice for script compatibility and execution.
The core command of the script SCRIPT_DIR=$(dirname "$(readlink -f "$0")")
serves to determine the script’s directory. The readlink -f "$0"
part of the command is key here. It resolves the full, absolute path of the script, including resolving any symbolic links that might be involved. The $0
variable represents the script’s name or path as it was called, making readlink -f
an effective tool for finding the true path of the script.
Following the resolution of the script’s full path, dirname
is used to extract just the directory part of this path. This is necessary to isolate the directory from the full script path, ensuring we only get the location where the script resides.
The script then assigns this directory path to the variable SCRIPT_DIR
. This assignment encapsulates the entire operation into a single, easily accessible variable.
Finally, the script concludes with echo "Script directory: $SCRIPT_DIR"
, a line that prints out the obtained script directory. This output serves as a straightforward confirmation of the script’s location, demonstrating the effectiveness of using readlink
in resolving the script’s directory, especially in scenarios involving symbolic links.
When executed, this script displays the script’s directory, affirming its successful operation. For example:
Script directory: /path/to/script
This output verifies the correct retrieval of the script’s directory, highlighting the utility of the readlink
command in Bash scripting for accurate path determination. This method is particularly valuable for scripts that need to handle symbolic links or require absolute path information.
Using realpath
Command to Get Script Directory in Bash
The realpath
command is a powerful tool in such scenarios, as it converts relative paths to absolute paths and resolves any symbolic links. This capability is particularly useful in complex scripting environments where scripts might be called from various locations. This guide will demonstrate how to use the realpath
command to reliably determine the script’s directory.
Here’s the complete working code for the task:
#!/bin/bash
# Determine the script's directory
SCRIPT_DIR=$(realpath "$(dirname "${BASH_SOURCE[0]}")")
# Display the script directory
echo "Script directory: $SCRIPT_DIR"
This script adeptly uses the realpath
command to ascertain the directory of the executing Bash script. The script commences with the shebang #!/bin/bash
, specifying that it should be run in the Bash shell environment, ensuring consistent behavior across different platforms.
The primary operation of the script is captured in the line SCRIPT_DIR=$(realpath "$(dirname "${BASH_SOURCE[0]}")")
. Here, ${BASH_SOURCE[0]}
is a special variable that represents the name of the script. This is a reliable way to reference the script, regardless of how it’s invoked, including in sourced scenarios.
The dirname
command is then utilized to extract the directory component from the script’s path, as indicated by ${BASH_SOURCE[0]}
. This step isolates the directory path from the full script name.
Next, the realpath
command transforms this directory path into an absolute path, resolving any symbolic links in the process. This ensures that the path is accurate and can be reliably used in various script operations.
The resultant absolute directory path is assigned to the variable SCRIPT_DIR
, centralizing the script’s location information in one place for easy access.
Finally, the script concludes with an echo
statement: echo "Script directory: $SCRIPT_DIR"
. This prints the determined script directory to the console, serving as a verification of the script’s successful execution and accurate directory resolution.
Upon execution, this script will display the absolute path of the script’s directory, affirming its functionality. For instance:
Script directory: /path/to/script
This output confirms the successful conversion of the script’s relative path to an absolute path, showcasing the effectiveness of the realpath
command in Bash scripting. Such a method proves invaluable in scenarios where absolute path resolution is critical for script operations, enhancing the robustness and reliability of script execution.
Conclusion
In this comprehensive guide, we’ve explored various methods for determining the script directory in Bash, a crucial skill for effective navigation and manipulation of directories in scripting. From using $0
and dirname
for basic scenarios to harnessing the BASH_SOURCE
array for more complex cases involving sourced scripts or multiple execution contexts, we’ve covered a range of techniques. The readlink
command’s ability to resolve absolute paths, including symbolic links, and the realpath
command’s functionality in converting relative paths to absolute paths, both offer robust solutions for accurately finding script directories in diverse environments.
Related Article - Bash Script
- How to Call Another Script From Current Script in Bash
- shebang in Bash Script
- How to Use getopts in Bash Script