How to Use getopts in Bash Script
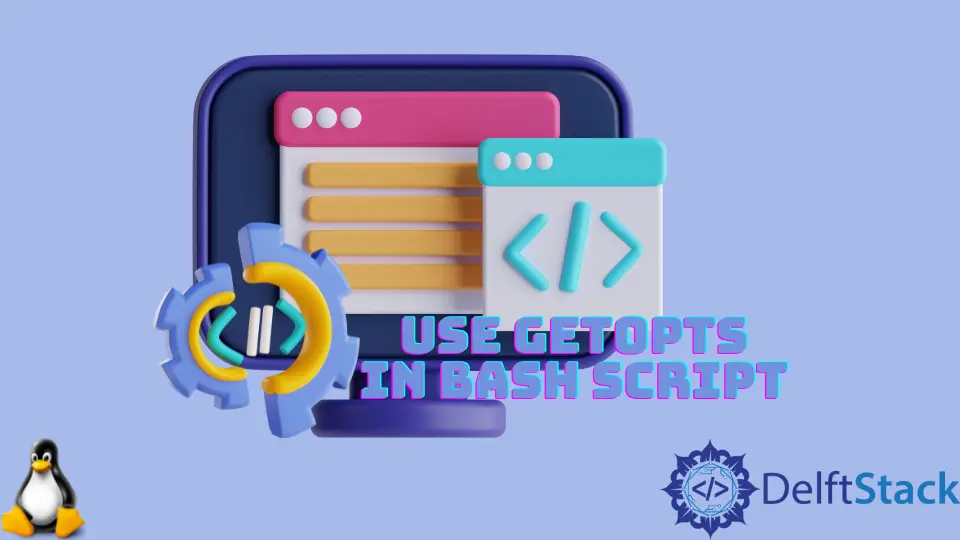
This tutorial shows the usage of getopts
in bash scripts by parsing options with arguments and parsing options without arguments.
Parse Options With Arguments in Bash getopts
The letters n
and c
both have :
in front of them. This means that we expect an argument to be provided whenever the option -n
or -c
is used. The variable opt
, holds the value of the current option that has been parsed by getopts
.
while getopts n:c: opt
do
case "${opt}" in
n) name=${OPTARG};;
c) country=${OPTARG}
esac
done
echo "I am $name";
echo "And I live in $country";
When we run the script, the -n
option provides John
as the argument while the -c
option provides Britain
as the argument.
bash flags.sh -n John -c Britain
Output:
I am John
And I live in Britain
Parse Options Without Arguments in Bash getopts
This will use a simple bash script that prints the script usage
when the -h
option is passed, and it prints the content of the folder when the -p
option is used with a specified path to the folder as the argument.
The first :
means that getopts
will not report any errors. Instead, we will handle the errors ourselves. The letter p
has a :
in front of it, while the letter h
does not. This means that whenever the option -p
is used, we expect an argument, but the -h
option can be used without an argument.
When the -h
option is passed, it invokes the usage
function. The -p
option assigns the argument passed to the path
variable, which is then passed to the list
function as an argument. The *
specifies the action to be taken whenever an option is passed that is not -h
or -p
.
#!/bin/bash
function usage {
printf "Usage:\n"
printf " -h Display this help message.\n"
printf " -p <folder path> List contents of specified folder.\n"
exit 0
}
function list {
ls -l $1
}
while getopts :p:h opt; do
case ${opt} in
h)
usage
;;
p) path=${OPTARG}
list $path
#echo $folder
;;
*)
printf "Invalid Option: $1.\n"
usage
;;
esac
done
Run the script with -h
option:
./getopts.sh -h
Usage:
-h Display this help message.
-p <folder path> List contents of specified folder.
Run the script with -p
option:
./getopts.sh -p /home/fumba/example
total 0
-rw-r--r-- 1 fumba fumba 0 Nov 1 21:43 file1.txt
-rw-r--r-- 1 fumba fumba 0 Nov 1 21:43 file2.txt
drwxr-xr-x 1 fumba fumba 4096 Nov 1 21:43 pictures
Run the script with an invalid option, -k
:
./getopts.sh -k
Invalid Option: -k.
Usage:
-h Display this help message.
-p <folder path> List contents of specified folder.