How to Create Directory in Bash
- Create a Folder if It Doesn’t Exist in Bash
-
Use
while
Loop to Create a Folder in Bash - Use the Recursion Method to Create a Folder in Bash
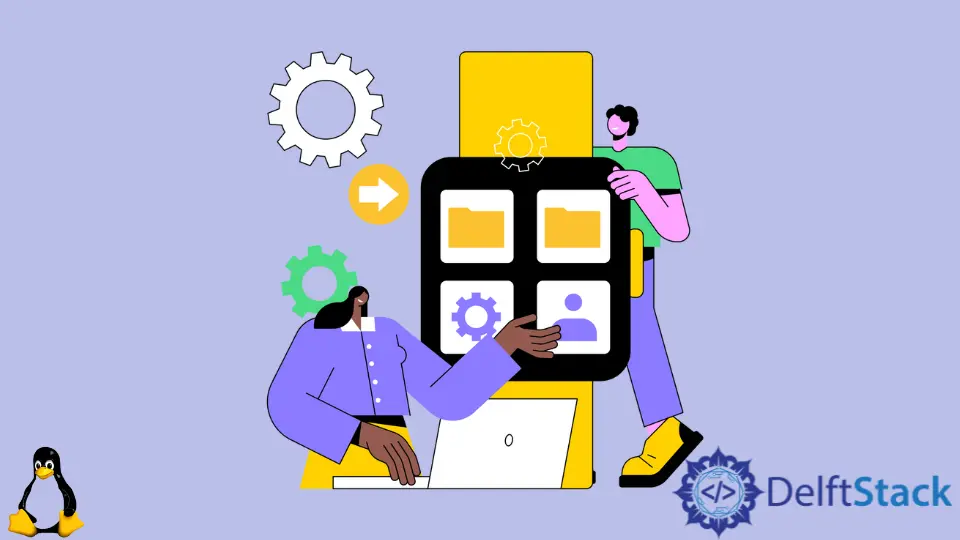
This article demonstrates how to create a folder if it doesn’t already exist in Bash.
Create a Folder if It Doesn’t Exist in Bash
We use the mkdir
command to create a new directory in Linux. We use mkdir -p
to avoid the error message.
Using the mkdir
command, you have to make every file one by one but, with the mkdir-p
, it will create it at once.
We can create a folder if it doesn’t already exist in Bash in two different ways. We can do that by using a while
loop in the function or the recursion method.
We can check the condition of whether the directory already exists or not by using this if[ -d $DirName ]
in the Bash script. We will create a Bash function that will take the directory name as input from the user.
It will create a new directory if the directory doesn’t exist. If the directory already exists, it will prompt the user to enter a different name.
Use while
Loop to Create a Folder in Bash
In the while
loop, we will get the input from the user by using the read
command in the Bash script. The user will enter the directory name that he wants to create.
If the entered directory name already exists in that case, the if[ -d $Dirname]
condition will return true. It will display a message to the user that the directory already exists.
It will prompt the user to enter a different directory name. This checking condition will continue until the user enters a directory name in the input that doesn’t already exist.
When the user enters a directory name that doesn’t already exist in that case, the if[ -d $Dirname]
condition will return false, and the else
block will execute that will create the new directory.
It will display the new directory has been successfully created
to the user, and the loop will break.
Example code:
#!/bin/bash
function mkdirectory() {
while [ true ]
do
if [ -d $DirName ]
then
echo "Directory Already exists:"
echo "Enter Different Name: "
read DirName
else
mkdir "$DirName"
echo " the new directory has been successfully created: $DirName"
break
fi
done
}
echo "Enter New DirName: "
read DirName
mkdirectory
Use the Recursion Method to Create a Folder in Bash
In the recursion method, the user gives the input through arguments. The user inputs the directory name like this, ./rcrdir.sh Dir
.
The rcrdir.sh
is the Bash file name, and Dir
is the directory name the user wants to create. The $1
is used to get the first argument (directory name).
If the user inputs a directory name that already exists in that case, the if[ -d $1]
condition will return true. It will display a message to the user that the directory already exists.
It will prompt the user to enter a different directory name. The user enters another name.
This checking will continue until the user enters a unique directory name in the input.
When the user enters a directory name that doesn’t already exist in that case, the if[ -d $1 ]
condition will return false, and the else
block will execute that will create the new directory.
It will display the new directory has been successfully created
to the user, and the loop breaks.
Example code:
#! /bin/bash
function mkdirectory(){
if [ -d $1 ]
then
echo "Directory aleady exists: "
echo "Enter different name:"
read directory
mkdirectory $directory
else
mkdir $1
echo " the new directory has been successfully created: $1"
fi
}
mkdirectory $1
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn