How to Find Files and Folders in Linux
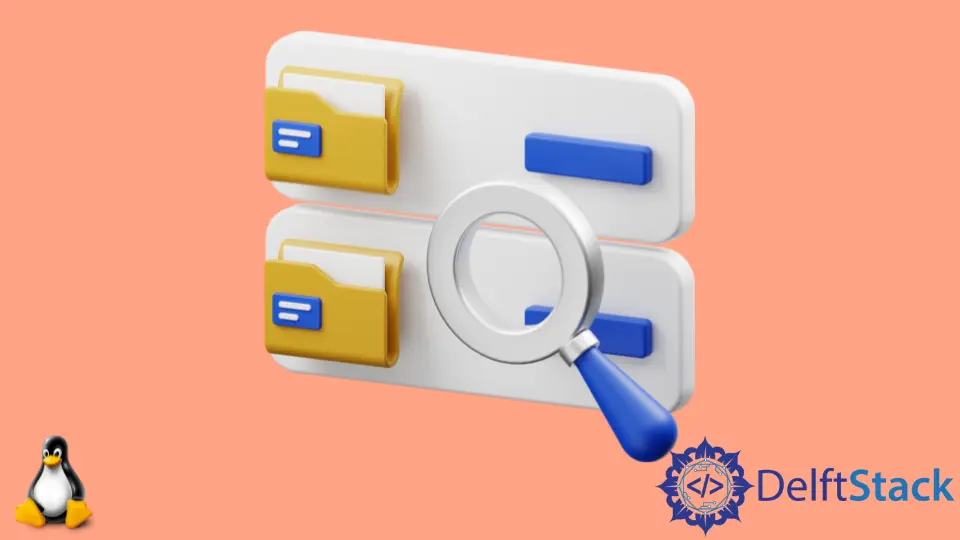
What do you do when you’re on the Bash command line and need to find a specific type of file, or a folder starting with a certain name, in a specified location? The find
command can help you locate files and folders that may fit a wide set of criteria, and as a result, is a powerful command-line utility in Linux.
This tutorial will explain the use of the find
command in a Bash shell context, going over the basic usage, advanced use cases, and usage combined with other Bash shell features.
Basic Uses of the find
Command in Bash
A find
command is a versatile tool used to find anything from a plain file to multiple files and folders that satisfy REGular EXpressions. The simplest use case, to find a specific file or folder in the current directory only, can be achieved as follows.
find filename.ext
find foldername
After executing the corresponding command, their names will print out if the file or folder exists.
The find
command can also search through the directory, entering subdirectories to find the specified file or folder. Suppose we have many files named test
scattered in folders in our current directory.
To see where they are located, we can type the following.
find . -name test -type f
The code should return the paths to all files named test
in the current directory and subdirectories. The use of the parameter -type f
ensures that we don’t catch directories also named test
- if you’re a programmer, you’re bound to have many of these.
The -name
parameter is self-explanatory, but if you would like case-insensitivity while searching for the specified name to catch names like tEST, Test, or TeST, we may use the -iname
parameter instead, where i
stands for insensitive.
Bash globs is not strictly related to the use of the find
command. You can use an asterisk positioned in your pattern in cases where you may not know which characters or how many of them exist in your pattern.
For example, if you’d like to catch the following files: test, test2, test_3, testt1, you could use a glob to capture these phrases based on the fact that they all start with the word test.
The following command does this.
find . -name test*
The code should return any files or directories (note the removal of the -type f
parameter) that begin with the word test.
Advanced Uses of the find
Command in Bash
The find
commands can be extended to match various criteria. If you would like to capture files based on multiple globs or names, you may want to consider using the -o
or OR function.
For example, if you would like to find both PDF and Word DOC/DOCX files in a directory, you can do that with the following.
find -iname '*.pdf' -o -iname '*.doc' -o -iname '*.docx'
Insensitive name parameters to catch files whose extension has been set to uppercase can be the case with certain programs.
If you’re more familiar with RegEx, you may use a pattern to specify multiple criteria as well, using the -regex
or -iregex
parameters.
To catch the same extensions as above, and additionally look for files starting with test, we can use the following pattern:
find -regex '.*/test*.\(pdf\|doc\|docx\)' -type f
The use of backslashes for special Regex characters like parentheses and the pipe symbol prevents Bash from processing them and passes the entire string directly into the find
command to prevent our RegEx from failing.
Finally, some discussion about how to use the output of the find
command - you could count how many occurrences of the matched files and folders there are by piping into wc
, which is a character/word/line counting utility.
find -regex '.*/test*.\(pdf\|doc\|docx\)' -type f | wc -l
You could save the data into a variable to be parsed by a command later in your script.
DOCS=$(find -regex '.*/test*.\(pdf\|doc\|docx\)' -type f)
Keep in mind that special characters, including spaces, newlines, and parentheses, may exist in file paths, resulting in weird behavior when passing such a variable to a command. Make sure to set the IFS delimiter accordingly while processing such data.
The find
command also provides per-item command execution similar to xargs
operation. You can achieve this using the -exec
parameter.
If you’d like to get the permissions for all the files and folders you match, for example, you can do that by doing the following:
find -regex '.*/test*.\(pdf\|doc\|docx\)' -type f -exec ls -l {} \;
Related Article - Bash File
- How to Write to a File in Bash
- How to Overwrite File in Bash
- How to Open HTML File Using Bash
- How to Search for Files With a Filename Beginning With a Specified String in Bash
- How to Check if a File Is Empty in Bash
- How to Read File Into Variable in Bash