How to Check if a File Is Empty in Bash
-
Use the
test
Command With the-s
Option Flag to Check if a File Is Empty in Bash -
Use the
ls
Command to Check if a File Is Empty in Bash
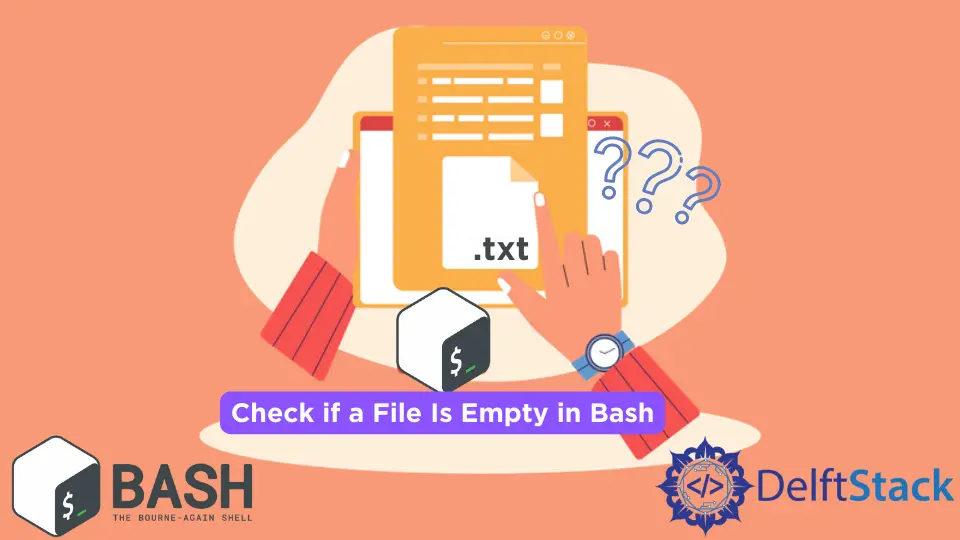
There is often a need to check if a file is empty or not, and thankfully there are a few simple methods to check that using Bash on the terminal or even with a Bash script.
Use the test
Command With the -s
Option Flag to Check if a File Is Empty in Bash
The test
command is one of the most helpful shell built-in commands and has many options for files and directories. The -s
option of the test
command checks if a file has a size greater than 0 or not.
If the file size exceeds zero, the command will return true. Otherwise, it will return false.
The use of test -s
is demonstrated below. We will explain the test
command using an example.
In this example, we have a file called newFile.txt
. We will create a Bash script titled empty_test.sh
, as follows:
#!/bin/bash
test -s newFile.txt && echo "file has something" || echo "file is empty"
If the file newFile.txt
is empty, then the shell will have the following output:
On the other hand, if newFile.txt
has any content, such as:
Hello!
Then the output will be as follows:
The above command is a straightforward method to check whether or not the selected file is empty. It will print file has something
if test -s newFile.txt
evaluates to true.
Otherwise, it will print file is empty
. This command can be used in a Bash script or run directly in the terminal.
It is important to note that if this command is used in a different directory or level than the file being checked, it will be necessary to write the complete file path.
There is an alternative way to write the above command. In Bash, [
is also a shell built-in and is equivalent to the test
command:
#!/bin/bash
[ -s newFile.txt ] && echo "file has something" || echo "file is empty"
This command is equivalent to the command we used previously.
We can also use the test -s
command to find all empty files in a given directory:
#!/bin/bash
for file in *.txt;
do if [ ! -s $file ];
then echo $file;
fi;
done
The above commands will print the names of all empty .txt
files in the directory. It can be easily customized to check different types of files by just replacing .txt
with another extension.
Use the ls
Command to Check if a File Is Empty in Bash
The ls
command lists all files and subdirectories in the current directory. The ls
command can also be used with the -s
option flag to show the files’ size next to their names.
For example, if we run the ls -s
command in the test
directory we have created with two subdirectories and a .docx
file, we get this output:
It is important to note that in macOS, this command will show the size of all subdirectories as 0. This is irrespective of whether or not the subdirectories have some other content.
It should also be noted that the default shell in use is zsh
(not the bash
). However, this is not a difference caused by the shell but by the Operating System itself.
To illustrate this result in a Linux environment, specifically using bash
, we created a test folder with two empty directories titled Test 1
and Test 2
. It also contains two text files, where test.txt
has a small string, and test2.txt
is a blank text file.
Regardless of the size of their contents, sub-directories are shown as 4 kilobytes
. This is not representative of the actual contents of the folder; this is the entry’s space occupied itself.
A better method is to use the ls
command with the -l
option flag, as this will show more information in the form of a table:
ls -l
We will run this command in the same Test
directory as before. The output is as follows:
As seen above, the ls -l
command gives more information and shows the correct size of subdirectories.
To see the size in KB, MB, and GB, use the option -lh
. The inclusion of the h
flag makes the data more human-readable.
The output will be something like the following: