How to Write to a File in Bash
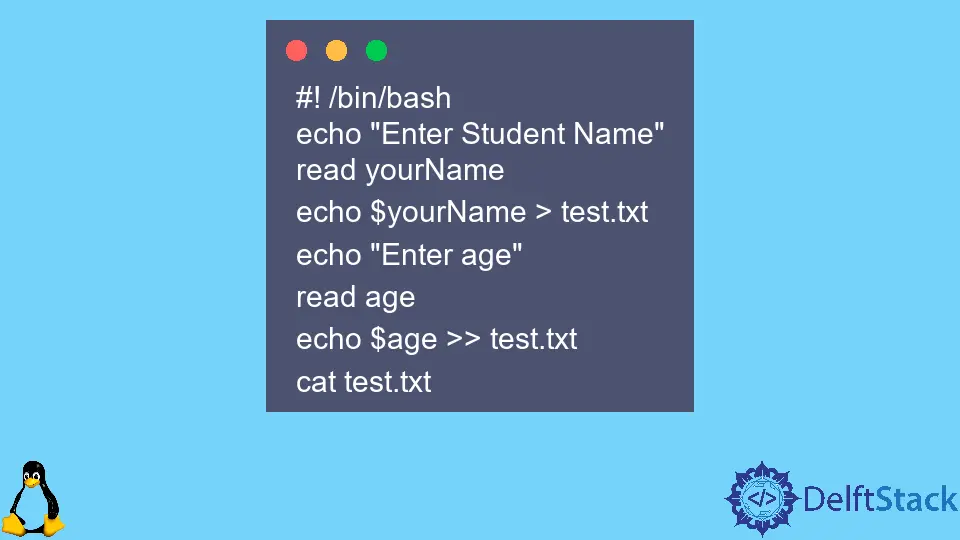
This tutorial will let us explore different approaches to writing files in bash. A file can be appended or overwritten based on the requirements. Let’s see how we can do it.
Different Ways to Write/Overwrite a File in Bash
We will see multiple operators, such as >
and >>
, to overwrite and append outputs to the files. Moreover, We will explore the tee
command to overwrite and append single and multiple files.
Use the >
Operator to Overwrite a File
The >
operator is used to overwrite the file. For example, the following code is used to overwrite a file.
echo "Overwriting in the file" > test.txt
The above command overwrites the test.txt
file if it exits; otherwise, it creates a new one. The echo
usually is not used because it does not support the text formatting.
We use printf
for formatted text instead of echo
. The following example shows the file overwriting using printf
.
printf "overwriting using the printf \n now in next line" > test.txt
The above command will format the string and add the new line after the printf
.
Use the >>
Operator to Write to a File
The >>
operator is used to append content at the end of the file. For example, the following command is used to append the data to the file named test.txt
.
echo "Append in the file" >> test.txt
Use the vim
Command to Write to a File
The vim
editor is also used to edit the file’s contents. For example, the following code will open the test.sh
file in the vim
editor.
sudo vim test.sh
After opening the vim editor, add the following script to perform the file writing operations.
#! /bin/bash
echo "Enter Student Name"
read yourName
echo $yourName > test.txt
echo "Enter age"
read age
echo $age >> test.txt
cat test.txt
After adding the code, press ESC and type w to close the file. Now run the file using the bash test.sh
.
The above script shows two approaches for file writing. In line 4, we used the >
operator, which overwrites the file. While line 7, use the >>
operator, which does not overwrite the previous content and appends the string to the new line.
Use the tee
Command to Write to a File
We can use the tee
command if we want to perform writing operations to the file and console simultaneously. It simultaneously takes the input and writes the string to the file and console.
Using the tee
command, we can see the content on the console that is being written in the file. The tee
command is also used to overwrite and append the file.
Use tee
Command to Overwrite a File
The following command is used for overwriting using the tee
command.
echo "Testing the Tee Command" | tee test.txt
The above command will take the string input from the pipe (|
) and write it simultaneously on the console and the file.
Use the tee
Command to Append to a File
The -a
flag is used with the tee
command to append a file. For example, the following code will append Testing the Tee Command
to the test.txt
file.
echo "Testing the Tee Command" | tee -a test.txt
Sometimes, we may need to write the content in multiple files simultaneously. We can use the tee
command to achieve this by specifying the names of all the files separated by a single space.
Following the bash command demonstrates writing the content to multiple files:
echo "Writing text to the multiple files" | tee test1.txt test2.txt test3.txt
The above command writes the input string on the console and the three text files simultaneously.