How to Extract Substring in Bash
-
Use the
cut
Command to Extract Substring in Bash - Use Substring Expansion to Extract Substring in Bash
-
Use
IFS
to Extract Substring in Bash
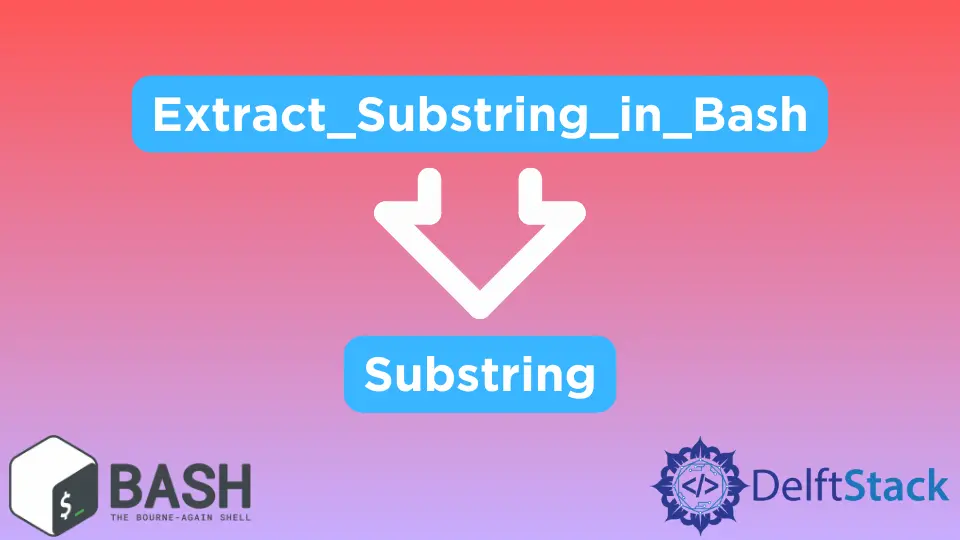
This tutorial demonstrates different ways to extract a substring in bash using the cut
command, substring expansion, and Internal Field Separator (IFS
).
Use the cut
Command to Extract Substring in Bash
The script below uses the cut
command to extract a substring. The -d
option specifies the delimiter to use to divide the string into fields and the -f
option sets the number of the field to extract.
In our case, the string is divided using _
as the delimiter, and to access the third field, we pass the argument 3
to the -f
option.
#!/bin/bash
printf "Script to extract a substring!\n\n"
numbers='one_two_three_four_five'
printf "full string: $numbers\n"
substring=$(echo $numbers | cut -d '_' -f 3)
printf "substring: $substring\n"
Run the bash script as follows.
bash substring.sh
From the output, a substring three
has been extracted from the string one_two_three_four_five
.
Script to extract a substring!
full string: one_two_three_four_five
substring: three
Use Substring Expansion to Extract Substring in Bash
Substring expansion is a built-in bash feature. It uses the following syntax.
$(variable:offset:length)
The variable
is the variable name containing the string. The offset
is used to specify the position from where to start the extraction of a string. The length
is used to specify the range of characters to be extracted, excluding the offset
.
The script below sets the variable name to numbers
, the offset
is set to 4
, and the length
of the string to be extracted is set to 3
.
#!/bin/bash
printf "Script to extract a substring!\n\n"
numbers='one_two_three_four_five'
substring=${numbers:4:3}
printf "full string: $numbers\n"
printf "substring: $substring\n"
Run the bash script as follows.
bash substring.sh
From the output, a substring two
has been extracted from the string one_two_three_four_five
.
Script to extract a substring!
full string: one_two_three_four_five
substring: two
Use IFS
to Extract Substring in Bash
IFS
stands for Internal Field Separator. The IFS
is used for word splitting after expansion and to split lines into words with the built-in read command.
In the script below, the IFS has been set to _
. This means it should split the string in the variable numbers
using _
as the delimiter. Once the string has been split, we can access the words using the syntax $[integer]
. The first word can be accessed by $1
, the second word by $2
, the third word by $3
, and so on.
#!/bin/bash
printf "Script to extract a substring!\n\n"
numbers='one_two_three_four_five'
IFS="_"
set $numbers
printf "full string: $numbers\n"
printf "substring: $2\n"
Run the bash script as follows.
bash substring.sh
From the output, a substring two
has been extracted from the string one_two_three_four_five
.
Script to extract a substring!
full string: one_two_three_four_five
substring: two