How to Convert Letter Case of Strings in Bash
-
Using
tr
in Bash to Convert Letter Case of Strings - Using Inbuilt Bash 4 Feature to Convert Letter Case of Strings
-
Using
awk
in Bash to Convert Letter Case of Strings -
Using
sed
in Bash to Convert Letter Case of Strings
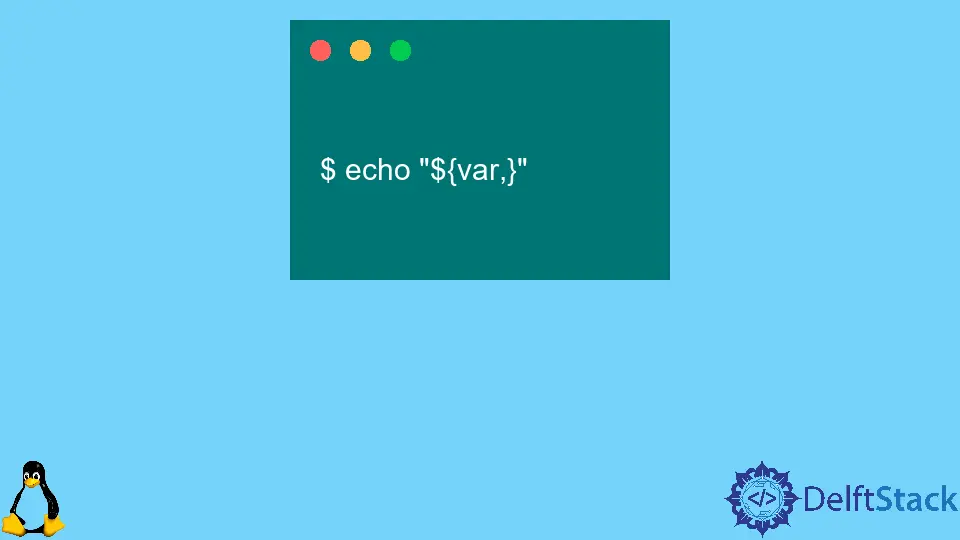
In bash commands, string data is utilized for a variety of functions.
We sometimes need to modify the string case to acquire the desired result. Upper and lower case strings can be transformed.
Using tr
in Bash to Convert Letter Case of Strings
In previous versions of bash, string data is translated using the tr
command. The term :upper
is used for uppercase letters, while the keyword :lower
is used for lowercase characters.
Let us look at an example.
echo "Changing All to LOWERCASE" | tr [:upper:] [:lower:]
Output:
changing all to lowercase
If we change the position of :upper:
and :lower:
as:
echo "Changing All to UPPERCASE" | tr [:lower:] [:upper:]
The output would be:
CHANGING ALL TO UPPERCASE
We have to put :lower:
later to convert strings into lowercase and vice-versa.
Using Inbuilt Bash 4 Feature to Convert Letter Case of Strings
Using the new features of Bash 4, you can convert string cases more easily.
The ^
symbol converts the first character of any string to uppercase, and the ^^
symbol converts the whole string to uppercase. The ,
sign is used to lowercase the initial character, while the ,,
symbol is used to lowercase the whole string.
For example, let us declare a variable used in the examples in this section.
$ var="Example String."
And we would want to make all of the letters in the variable var
uppercase.
$ echo "${var^^}"
Output:
EXAMPLE STRING.
Or perhaps we want to turn the first letter into lowercase.
$ echo "${var,}"
Output:
example String.
Now, let’s change the letter case of the character by selecting it and first changing the i
character from the string to uppercase.
$ echo "${var^^i}"
Output:
Example StrIng.
Let’s do the same for two characters, r
and i
, and convert them to uppercase.
$ echo "${var^^[r,i]}"
Output:
Example StRIng.
Use ,,
instead of ^^
to change into lowercase.
Using awk
in Bash to Convert Letter Case of Strings
Again,
$ var="Example String."
To convert all the strings into lowercase, we use the tolower()
method of awk
. It also has a toupper()
function to convert all strings into uppercase.
$x
refers to the current record’s fields. $0
is a special case that refers to the entire record.
$ echo "$var" | awk '{print tolower($0)}'
Output:
example string.
To convert all the string into uppercase:
$ echo "$var" | awk '{print toupper($0)}'
Output:
EXAMPLE STRING.
Using sed
in Bash to Convert Letter Case of Strings
The sed
script is made up of substitution commands. Substitute commands are s/old/new/
, which searches the text for something that matches the regular expression old
and replaces it with new
.
Again,
$ var="Example String."
s/.*/\L&/
matches any character in the input and replaces it with a lower case version of the same character.
$ echo "$var" | sed 's/.*/\L&/'
Output:
example string.