How to Assign Default Value in Bash
- Using Parameter Expansion
-
Using the
if
Statement -
Using the
:-
Operator for Default Values - Conclusion
- FAQ
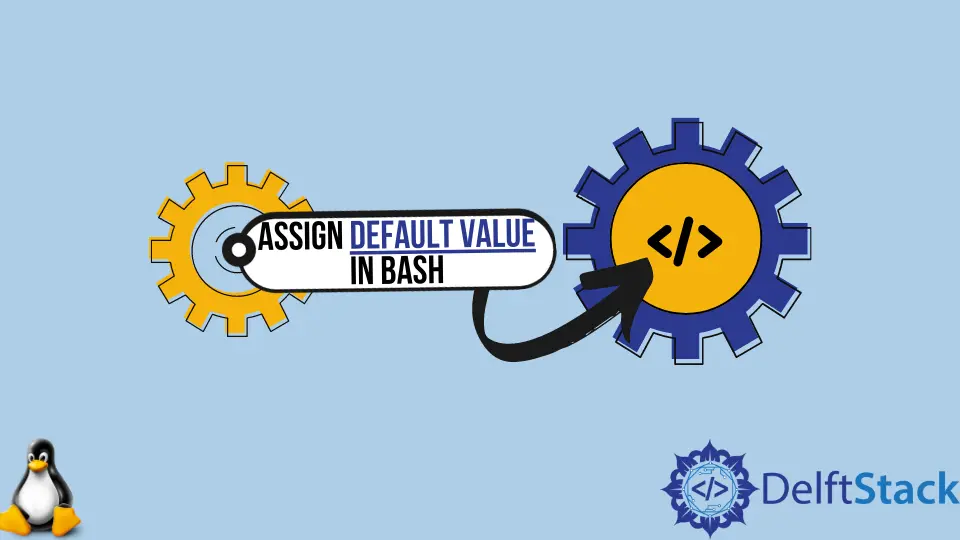
When working with Bash scripts, you may often find yourself needing to assign default values to variables. This is particularly useful when you want to ensure that a variable has a meaningful value even if the user or script fails to provide one.
In this article, we will explore various approaches to assign default values in Bash, focusing on methods that are easy to implement and understand. Whether you are scripting for automation, system administration, or development purposes, knowing how to assign default values can save you time and prevent errors. Let’s dive into the different techniques and see how they can be applied in real-world scenarios.
Using Parameter Expansion
One of the simplest ways to assign a default value to a variable in Bash is through parameter expansion. This method allows you to specify a default value that will be used only if the variable is unset or null. Here’s how it works:
#!/bin/bash
variable=${1:-"default_value"}
echo "The value of the variable is: $variable"
In this script, we use ${1:-"default_value"}
to assign the first positional parameter to variable
. If no argument is provided when the script is executed, variable
will take on the value “default_value”.
Output:
The value of the variable is: default_value
If you run the script with an argument, like ./script.sh custom_value
, the output will change accordingly:
Output:
The value of the variable is: custom_value
This method is concise and effective, making it a popular choice among Bash scripters. It ensures that your variable always has a value, which can help prevent unexpected behavior in your scripts.
Using the if
Statement
Another approach to assign default values in Bash is by using an if
statement. This method gives you more control and can be particularly useful when the logic for assigning a default value is more complex. Here’s an example:
#!/bin/bash
if [ -z "$1" ]; then
variable="default_value"
else
variable="$1"
fi
echo "The value of the variable is: $variable"
In this script, we check if the first positional parameter is empty using the -z
test. If it is, we assign “default_value” to variable
. Otherwise, we assign the value of $1
.
Output:
The value of the variable is: default_value
When the script is executed with an argument, such as ./script.sh custom_value
, the output will reflect the provided value:
Output:
The value of the variable is: custom_value
This method is particularly useful when you need to perform additional checks or logic before assigning a value to your variable, making it a versatile choice for more complex scripts.
Using the :-
Operator for Default Values
The :-
operator in Bash is another handy tool for assigning default values. This operator works similarly to parameter expansion but can be used in a slightly different context. Here’s an example:
#!/bin/bash
variable=${variable:-"default_value"}
echo "The value of the variable is: $variable"
In this script, we are checking if variable
is set. If it is not set or is null, it will be assigned “default_value”.
Output:
The value of the variable is: default_value
If you run the script after setting the variable, like variable="custom_value" ./script.sh
, the output will be:
Output:
The value of the variable is: custom_value
This method is particularly useful when you want to ensure that a variable retains its value if it has already been set, while still providing a fallback option.
Conclusion
Assigning default values in Bash is an essential skill for anyone looking to write robust and error-free scripts. Whether you choose to use parameter expansion, if
statements, or the :-
operator, each method has its advantages depending on the complexity of your script. By incorporating these techniques into your Bash scripting toolkit, you can ensure that your variables always have meaningful values, leading to smoother execution and fewer errors. Experiment with these methods to find the one that best suits your scripting style and needs.
FAQ
-
How do I assign a default value to a variable in Bash?
You can use parameter expansion,if
statements, or the:-
operator to assign default values in Bash. -
What happens if I don’t assign a default value?
If you don’t assign a default value, the variable may remain unset or null, which can lead to unexpected behavior in your scripts. -
Can I use default values with functions in Bash?
Yes, you can use the same methods to assign default values to variables within functions in Bash. -
Are there any performance differences between these methods?
Generally, the performance differences are negligible for most scripts. Choose the method that best fits your needs and coding style. -
Can I assign default values to multiple variables at once?
Yes, you can assign default values to multiple variables using the same techniques, either in a single line or with separate statements.