How to Get SimpleName in Kotlin
-
Get SimpleName in Kotlin Using
KClass
-
Get SimpleName in Kotlin Using
::class.simpleName
-
Get SimpleName in Kotlin Using
::class.java.simpleName
- Create Our Class and Get Its SimpleName
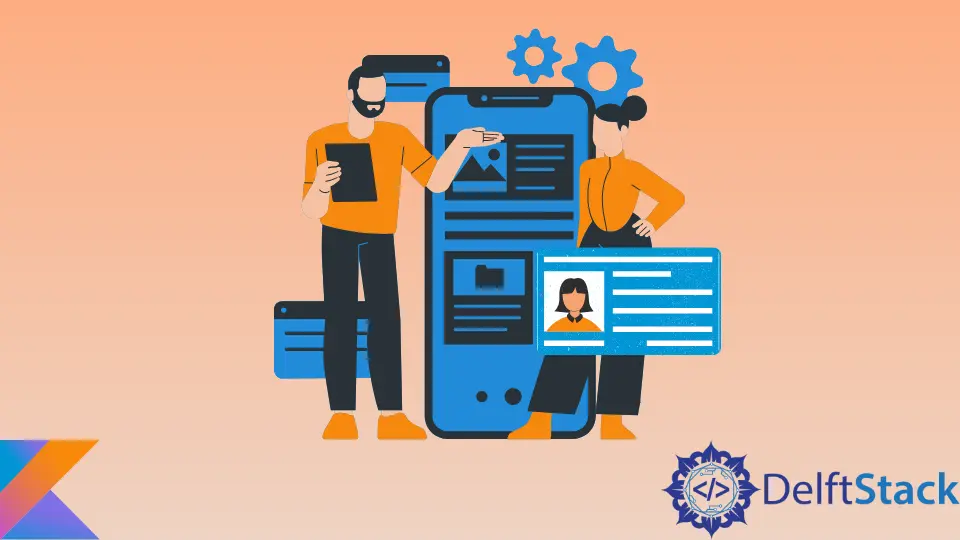
Jave’s getSimpleName()
method allows us to retrieve the SimpleName of the class of an object. We can use the method in the following way to get a class’s name.
String var = objectName.getClass().getSimpleName();
But when we try to convert this code to Kotlin and run it to get a class’s name, it throws an error.
So how do we get the SimpleName in Kotlin? This post introduces equivalent ways to get a class’s SimpleName in Kotlin.
Get SimpleName in Kotlin Using KClass
The easiest way to get a class’s SimpleName in Kotlin is using KClass
. The KClass
holds references to Kotlin’s classes and offers introspection capabilities.
We can use the ::class
reference to use the KClass
to retrieve the SimpleName of a class.
Now, to get the SimpleName, there are two ways. We can either use ::class.simpleName
or we can use ::class.java.simpleName
. We will go through both ways.
Get SimpleName in Kotlin Using ::class.simpleName
To retrieve the class’s SimpleName, we need to use the ::class.simpleName
along with the variable or object whose class’s name we want to get. Here’s an example of the same:
fun main() {
val v = "Android programming!"
println(v::class.simpleName)
}
Output:
Get SimpleName in Kotlin Using ::class.java.simpleName
Here, we only need to add the word java
. It does the same thing as class.simpleName
and gets the SimpleName of the Kotlin class.
fun main() {
val v = "Android programming!"
println(v::class.java.simpleName)
}
Output:
Create Our Class and Get Its SimpleName
We can also create our own class and use KClass
to get its SimpleName. We might need to get the class SimpleName we created while working on large projects.
If we are working on a project with multiple programmers, we might need to get the class SimpleName the other programmer might have built to use it for running different methods.
Here’s an example code to demonstrate the use of ::class.simpleName
in a class we define.
class exampleClass {
private var v: String = "Kotlin"
}
fun main(args: Array<String>) {
val ob1 = exampleClass() // Object ob1 of exampleClass
println(ob1::class.simpleName)
}
Output:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn