How to Format String in Kotlin
- Format String in Kotlin
- Use String Templates to Format String in Kotlin
-
Use
String.format()
to Format String in Kotlin
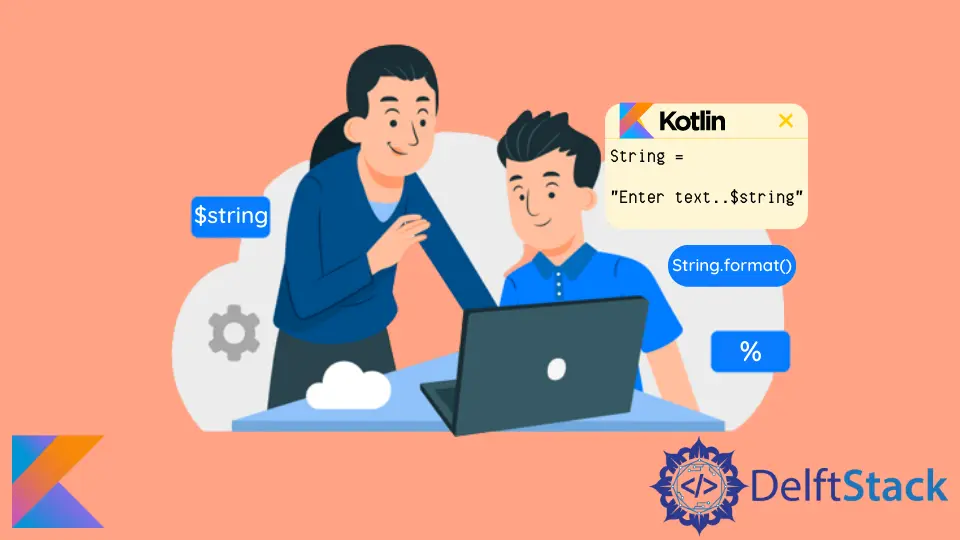
This tutorial teaches how to format string in Kotlin with string templates and the format()
method.
Format String in Kotlin
The string
class in Kotlin represents character strings. All the strings in Kotlin are objects of the String
class.
Objects of the String
class are immutable as in Java and can be initialized using double quotes ""
.
Formatting string lets the developer define a template that can be used with multiple objects. There are two ways to format string in Kotlin: the String Templates
and String.format()
method.
Use String Templates to Format String in Kotlin
String templates allow us to insert variable values and functions returning values directly into String
. The $
(dollar) sign is used before mentioning the expression.
It evaluates the expression and concatenates the result to the string.
Let us look at the syntax and examples to understand string templates better.
Syntax:
// for variable
var var_name : String = "Enter any text..$var1"
// for function
var var_name : String = "Enter any text..${function here}"
Let us see an example to print a welcome message by including the user’s name.
fun main(){
println("Enter your Name")
var name = readLine()
// printing name using String Template
var welcome_message = "Welcome to Kotlin Strings! $name"
println(welcome_message)
}
We first ask the user for their name and store it in the name
variable. This variable is later used in welcome_message
with the $
(dollar) sign to concatenate the value of the name variable to the string.
Output:
Enter your Name
Programmer
Welcome to Kotlin Strings! Programmer
As we see in the output, the user’s name is printed instead of the "$name"
. Thus we don’t need to break the string flow for inserting variable’s value.
We can attach them using the $
symbol before the variable name.
Similarly, we can use functions as well in the string initialization. Let us take an example of factorial.
// function to calculate factorial
fun fact(n : Int) : Int{
if(n==0 || n==1) return 1
return n*fact(n-1)
}
// main function
fun main(){
println("Enter number")
var num =-1
// reading user input
num = Integer.parseInt(readLine())
// using function in string
println("Factorial of $num = ${fact(num)}")
}
Output:
Enter number
5
Factorial of 5 = 120
As in the above example, we firstly created a function to calculate the factorial of the given number. If the number is 0 or 1, 1 is returned.
If the number is greater than 1, then a recursive call is made, and the current n
is multiplied with the fact
function and n-1
as a parameter. In the main method, we take user input for num
whose factorial we are supposed to find.
In println
, we use $num
to print the value of num, and in ${fact(num)}
, fact(num)
is a valid Kotlin expression; thus, we don’t escape the double-quotes. It returns the Int
value, which is concatenated to the string by ${}
.
The string template parser starts solving it from the most nested template evaluates it until the result is formed.
Use String.format()
to Format String in Kotlin
String.format()
method in Kotlin is present in the String
class and is borrowed from Java. We can use this method to pre-define format some particular string.
The number of arguments must equal the number of format specifiers; Else, an exception is raised.
Type specifiers:
We can use as many specifiers as required in a string. Here’s a list of specifiers.
%b
- Boolean%c
- Character%d
- Signed Integer%e
- Float in Scientific Notation%f
- Float in Decimal Format%g
- Float in Decimal or Scientific Notation, depending on the value%h
- Hashcode of the supplied argument%n
- Newline separator%o
- Octal Integer (base 8)%s
- String%t
- Date or Time%x
- Hexadecimal Integer (base 16)
Syntax:
var my_str = String.format("Some text..%specifier_here", variables for specifiers)
String.format()
is called using String
or an object of string
class. It takes a string containing specifiers and variables as the major string as parameters in the same order.
It returns a String
as defined. It also throws an exception in case of a mismatch of specifiers or if specifiers don’t equal the number of variables.
Let us look at an example of String.format()
to display various details of a student.
fun main(){
// studnt details
var name = "Taylor Swift"
var roll_no = 4676
var percentage = 96.3
var grade = 'A'
var school = "Delhi Public School"
// formating using String.format() method
var student_data = String.format("Name: %s%nRoll No.: %d%nPercentage: %.2f%%%nGrade: %c%nSchool Name: %s", name, roll_no, percentage, grade, school)
println(student_data)
}
Output:
Name: Taylor Swift
Roll No.: 4676
Percentage: 96.30%
Grade: A
School Name: Delhi Public School
All the details of a student are stored in variables. We use type specifiers to concatenate variables to the string.
The first %s
refers to a string variable, i.e., name. The %d
refers to an integer value, i.e., roll_no
and so on.
The %n
is used for the next line. Also, to print the %
symbol in the output, we have used %%
in the code for percentage.
The %.2f
is for precision, i.e., the number of digits after the decimal, in this case, is 2.
We can also define the default format. Instead of using the string in String.format()
, we can create a String
class object, say obj_str
, and use it to format and print various variables in a string.
Let us take an example of a map where we store "How to say hello in different languages"
, where keys are hello in the particular language and value is the name of the language.
fun main(){
var say_hello = mapOf(
"Hello" to "English",
"Goedendag" to "Dutch",
"Namaste" to "Hindi",
"Bonjour" to "French",
"Hola" to "Spanish"
)
// defining default format
var string_format = "%s\t - %s%n"
println("Greetings\tLanguage")
// printing contenets of map using default format
for((k, v) in say_hello)
print(string_format.format(k,v))
}
Output:
Greetings Language
Hello - English
Goedendag - Dutch
Namaste - Hindi
Bonjour - French
Hola - Spanish
In the above example, we defined a format for a string named string_format
, and instead of String.format
, we used string_format.format(//variables..)
that prints key, tab, dash(-
), value and next line for each key-value pair.
Niyati is a Technical Content Writer and an engineering student. She has written more than 50 published articles on Data Structures, Algorithms, Git, DBMS, and Programming Languages like Python, C/C++, Java, CSS, HTML, KOTLIN, JavaScript, etc. that are very easy-to-understand and visualize.
LinkedIn