How to Concatenate Strings in Kotlin
-
Using the
plus()
Method to Concatenate Strings in Kotlin -
Using the
+
Operator to Concatenate Strings in Kotlin - Using String Templates to Concatenate Strings in Kotlin
- Using StringBuilder to Concatenate Strings in Kotlin
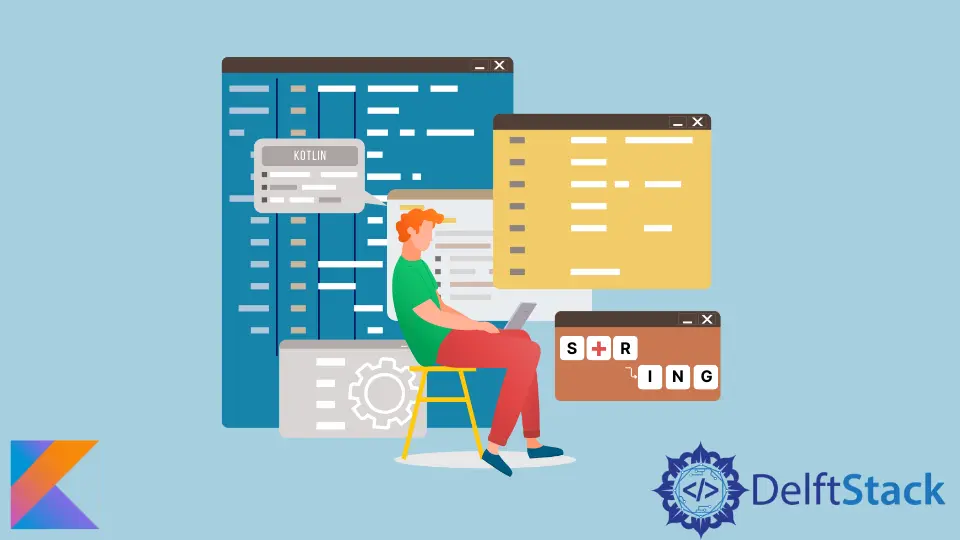
This article introduces how to correctly concatenate or combine two or multiple strings in Kotlin.
Concatenation is an operation of joining two Strigs together. We can perform concatenation using various ways in Kotlin; they are explained below.
Using the plus()
Method to Concatenate Strings in Kotlin
The string class in Kotlin contains the plus()
method to combine two strings.
Syntax:
String3 = String1.plus(String2)
plus()
method replaces the concate()
method and satisfies the purpose.Example:
fun main(args : Array<String>){
var greet = "Hey"
var message = "Good Morning"
var greetings = greet.plus(", ").plus(message)
println(greetings)
}
We first add ,
(comma) and a white space to Hey
. In the resultant string, we append the message Good Morning
using the plus()
method.
Output:
Hey, Good Morning
Using the +
Operator to Concatenate Strings in Kotlin
We can also use the + (plus) operator to add two strings in Kotlin.
Syntax:
var String3 = String1+String2
Example:
fun main(args : Array<String>){
var id = 1842
var name = "Michael"
var username = name+"_"+id
println("Your auto-generated Username : "+username)
}
In the above example, we generate a user username using the integer type and the name of the string type. We use the +
operator to join name, an underscore, and id to generate the username.
Output:
Your auto-generated Username : Michael_1842
Using String Templates to Concatenate Strings in Kotlin
We can also use String templates to concatenate strings in Kotlin. String templates contain expressions evaluated to build a String using the dollar $
sign.
Syntax:
String3 = "$String1 $String2"
Example:
fun main(args : Array<String>){
var num1 = 45
var num2 = 64
var sum = num1+num2
var result = "Sum of $num1 and $num2 is $sum"
println(result)
}
In the example above, to refer to the value of num1, num2, and sum
, we use the $
sign. It simply evaluates their values and concatenates to the current string without breaking the flow, as in the case of the plus (+) operator or the plus()
method. We can also use String templates to evaluate the expressions using curly brackets {}
.
Output:
Sum of 45 and 64 is 109
Using StringBuilder to Concatenate Strings in Kotlin
As we know, strings are immutable in Kotlin, where there is multiple string creation; we can also use StringBuilder to avoid unnecessary String objects and reduce the program’s time complexity.
Syntax:
var variable_name = StringbUilder()
variable_name.append("text to be concatenated")
Example:
fun main(args : Array<String>){
var hashtag = StringBuilder()
hashtag.append("#Kotlin\n")
hashtag.append("#Programming\n")
hashtag.append("#Strings\n")
println(hashtag)
}
We can use the append() function of the StringBuilder to concatenate texts to existing ones in String Builder. \n
moves the string to the following line.
Output:
#Kotlin
#Programming
#Strings
As we can see in the output, each hashtag is added to the existing string and moved to the next line.
Niyati is a Technical Content Writer and an engineering student. She has written more than 50 published articles on Data Structures, Algorithms, Git, DBMS, and Programming Languages like Python, C/C++, Java, CSS, HTML, KOTLIN, JavaScript, etc. that are very easy-to-understand and visualize.
LinkedIn