How to Split a String Into an Array in Kotlin
- Create a Kotlin Project
- Read Text From a File in Kotlin
-
Use the
split()
Method and theArray()
Constructor to Split a String Into an Array in Kotlin -
Use the
split()
andtoTypedArray()
Methods to Split a String Into an Array in Kotlin - Remove Leading and Trailing Whitespaces After Splitting a String Into an Array in Kotlin
- Conclusion
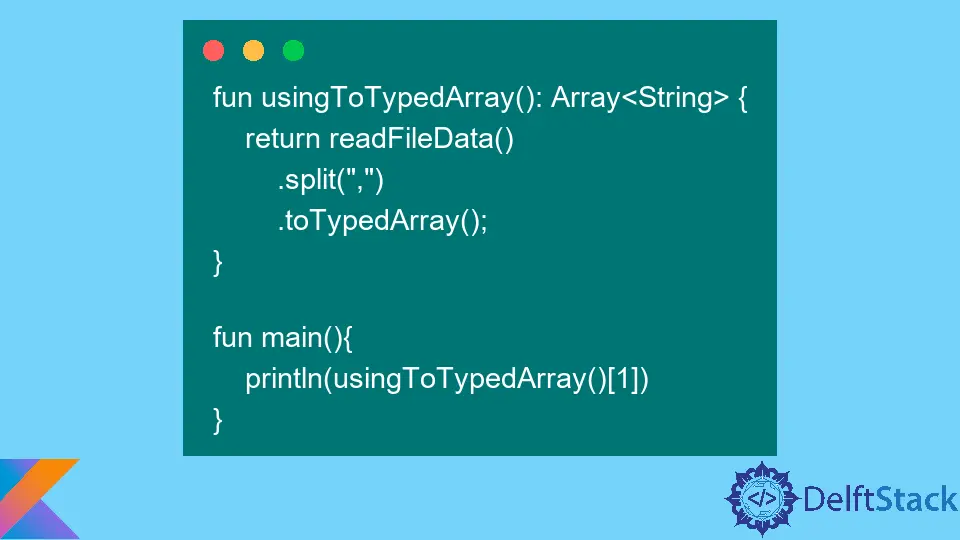
A series of character sequences always form a string, and we can retrieve each of the character sequences when there is a need to do so. For example, in a real-world application, users usually use a sequence of characters to search for a particular service or product in the application.
We do not usually use the entire sequence of characters to perform a search. We typically retrieve keywords from the search term and use them to perform the search.
This tutorial will show how to split a string into an array of strings in Kotlin. You can use this technique to search in an application, as mentioned above, by retrieving the keywords from the string array.
Create a Kotlin Project
Open IntelliJ IDEA and select File > New > Project
. On the window that opens, enter the project name as split-string
, select Kotlin
in the Language section, and select IntelliJ
in the Build System section.
Finally, press the Create
button to generate the project.
Once the project is generated, create a file named data.txt
under the src
folder and paste this text into the file.
Your, service, provider, is, wishing, you, a, good, day
This text contains a single line of a string, with each string separated from the other using a comma. We will use this file to read the string into our application.
Read Text From a File in Kotlin
Create a second file named Main.kt
under the src/main/kotlin
folder and copy and paste the following code into the file.
import java.io.File
private val fileData = File("src/data.txt");
fun readFileData(): String {
return fileData.bufferedReader()
.readLine();
}
fun main(){
println(readFileData());
}
In this code, we have created a method named readFileData()
that uses a BufferedReader
to read the line we added in the data.txt
file using the readLine()
method.
The BufferedReader
provides an efficient way of reading data from a file because the data is read into a buffer instead of reading it directly from the file. If we need this data again, the data will be obtained from the buffer.
The readLine()
method reads a single text line if one exists; else, the method returns null. The end of a line is identified by a line feed \n
, a carriage return \r
, or EOF
, which means the end of the file.
Run this code and note that our file is read successfully. The contents will be logged to the console, as shown below.
Your, service, provider, is, wishing, you, a, good, day
Use the split()
Method and the Array()
Constructor to Split a String Into an Array in Kotlin
Comment on the main()
method in the previous example and copy and paste the following code into the Main.kt
file after the readFileData()
method.
fun usingSplitAndArray(): Array<String> {
val stringData: List<String> = readFileData()
.split(",");
return Array(stringData.size) { index ->
stringData[index]
}
}
fun main(){
println(usingSplitAndArray()[0])
}
In this code, we have created a method named usingSplitAndArray()
that calls the split()
method on the string returned by the readFileData()
method to return a list of string List<String>
values.
The split()
method uses commas as delimiters for the string. Note that you can pass a variable number of delimiters in the split()
method to achieve the desired result.
Since we wish to return an array of strings Array<String>
, we use the list returned to create a new Array using the Array()
constructor. This constructor accepts two arguments: the first being the array size and the second being a lambda expression that accepts an int and returns a type T
.
Since our list can provide all these arguments, we pass the list size as the first argument of the Array()
constructor, and the second argument uses the index of each item in the list to return the array’s values.
To verify that our string was converted to an array, we have logged the value at index 0
from the returned array usingSplitAndArray()[0]
in the main()
method.
Run this code and ensure the output is as shown below.
Your
Use the split()
and toTypedArray()
Methods to Split a String Into an Array in Kotlin
Comment out the main()
method in the previous example and copy and paste the following code into the Main.kt
file after the usingSplitAndArray()
method.
fun usingToTypedArray(): Array<String> {
return readFileData()
.split(",")
.toTypedArray();
}
fun main(){
println(usingToTypedArray()[1])
}
In this code, we created a function named usingToTypedArray()
that returns an array of strings Array<String>
from the list returned by the split()
method.
In the previous example, we mentioned that the split()
method returns a list of strings List<String>
, and we can use the Array()
constructor to create an array of strings.
This example is much easier than the previous example as we only need to call the toTypedArray()
method on the returned list to return a typed array which is simply an array of strings Array<Strings>
.
In the main()
method, we logged the value at index 1
returned by the array to verify that the string was converted to an array.
Run this code and ensure the output is as shown below.
service
Remove Leading and Trailing Whitespaces After Splitting a String Into an Array in Kotlin
Comment out the main()
method in the previous example and copy and paste the following code into the Main.kt
file after the usingToTypedArray()
method.
fun usingTrimMethod(): Array<String> {
return readFileData()
.split(",")
.map(String::trim)
.toTypedArray()
}
fun main(){
println(usingTrimMethod()[1])
}
Note that our text contains leading whitespaces and the previous example returned a value with the whitespace as part of the string. To remove the leading and trailing whitespaces, we invoke the map()
method and pass the trim()
method as its argument.
The trim()
method is a transform function that removes all the leading and trailing whitespaces if any exist. This example is similar to the previous one, and its main objective is to show how to eliminate whitespaces.
Run this example and note that the value logged to the console, in this case, does not have whitespace, as shown below.
service
Conclusion
In this tutorial, we have learned how to split a string into an array. The topics we have covered include:
- Reading a line of string from a text file.
- Using the
split()
method and theArray()
constructor to return an array of strings. - Using the
split()
method andtoTypedArray()
method to return an array of strings.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub