How to Use the NPM jQuery Module
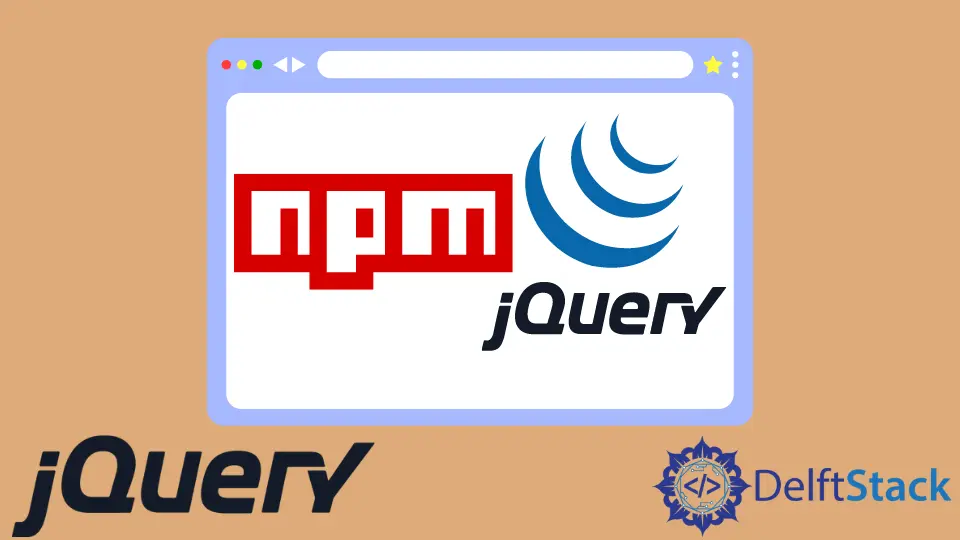
NPM is a part of the node.js
package.
This tutorial demonstrates installing and using the npm
jQuery module.
Use the NPM jQuery Module
Install Node.js With NPM
To use the npm
jQuery module first, we need to install the npm
module, which is included in the node.js
package. Go to this link and download your installer.
Once the installer is downloaded, the next step is to run the installer.
After running the installer, press Next
and accept the agreement; after that, press the Next
three times.
Check the option automatically install the necessary tools
, click Next
and then click Install
.
Once the installation is completed, click Finish
, and the Node.js with npm is installed.
Verify the installation through command prompt or PowerShell. Run the following command.
npm -v
Once the Node.js with npm is installed, the next step is to install the jquery
module with npm.
Install jquery
With NPM
Ensure you install the module jquery
, not the jQuery
. Follow the steps below.
Step 1: Make the package.json
file by using the following command in the command prompt to keep track of dependencies and modules.
npm init -y
The output for the above command will be something like this:
C:\>npm init -y
Wrote to C:\package.json:
{
"name": "",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Step 2: Install the jquery
module; use the following command.
npm install jquery
Step 3: Once the jquery
module is installed, the next step is to install jsdom
because jQuery is a front-end library; that is why it needs a window for backend operations. Use the following command to install jsdom
.
npm install jsdom
Step 4: Import the jsdom
module in JavaScript.
const dom = new jsdom.JSDOM("")
Step 5: Create a new jsdom
window created by the JSDOM
object with HTML code as a parameter. See the example below.
const dom = new jsdom.JSDOM("")
Step 6: Now import jquery
and provide it with a window. Use the following code.
const jquery = require('jquery')(dom.window)
Now let’s try an example to use jquery
with npm. See example:
// Import the jsdom module
const jsdom = require('jsdom');
// Create a window with a document
const dom = new jsdom.JSDOM(`<!DOCTYPE html>
<body>
<h2> DELFTSTACK </h2>
<p>This is delftstack.com</p>
</body>
`);
// Import the jquery with window
const jquery = require('jquery')(dom.window);
// Append a HTML element to the body
jquery('body').append('<p> The Best Tutorial Site </p>');
// Get the content of the body
const Body_Content = dom.window.document.querySelector('body');
// Print the content of body
console.log(Body_Content.textContent);
The code above will use npm jquery
to append some HTML content to the body. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook