How to Check and Uncheck a Checkbox in jQuery
-
Use the
prop()
Method to Check and Uncheck a Checkbox in jQuery -
Use the
attr()
Method to Check and Uncheck a Checkbox in jQuery
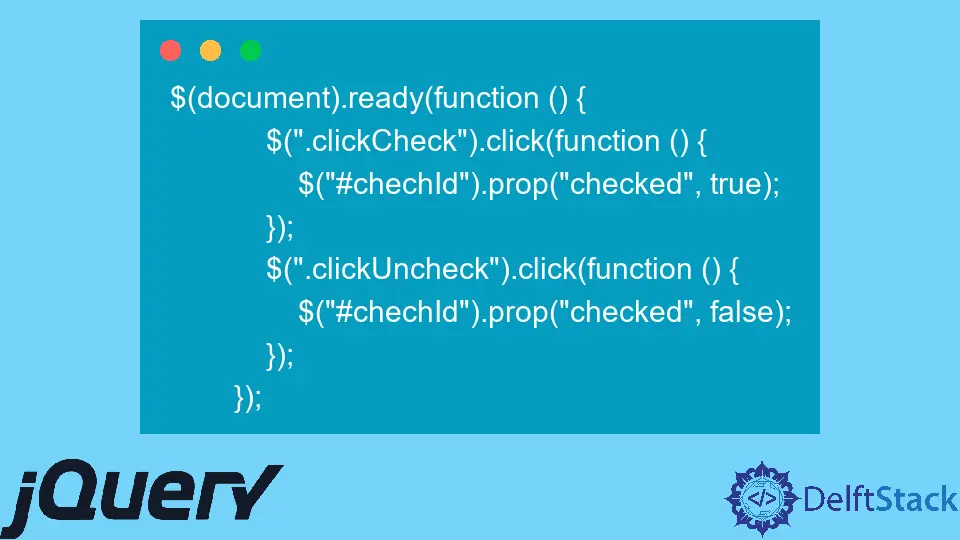
There are two ways to check and uncheck a checkbox. For the versions of jQuery above 1.6, the prop()
function is used, and for versions below 1.6, the attr()
function is used.
Use the prop()
Method to Check and Uncheck a Checkbox in jQuery
The prop()
method is used to access the input and sets its property. This method manipulates the checked property, fixing it true
or false
based on whether we want to check or uncheck a checkbox.
Syntax:
$("#checkbox_name").prop("checked", true);
According to the W3C form’s definition, the checked
attribute is a boolean attribute, implying that the associated property is actual if the element is present - even if the quality has no value or is set to false
.
Example:
$(document).ready(function () {
$(".clickCheck").click(function () {
$("#chechId").prop("checked", true);
});
$(".clickUncheck").click(function () {
$("#chechId").prop("checked", false);
});
});
clickCheck
is the class name of a button Yes
whereas clickUncheck
is the class name of a button No
. Similarly, #chechId
is the id name of the checkbox used here, which is used to check and uncheck a checkbox.
Output:
Here, you can see two buttons, i.e., Yes
and No
. When you click the button Yes
it will check the checkbox as it triggers the checkbox property, i.e., true
.
Likewise, when you click the button No
, it will uncheck the checkbox as it triggers the checkbox property, i.e., false
.
Use the attr()
Method to Check and Uncheck a Checkbox in jQuery
It is identical to the earlier method but is more appropriate for earlier jQuery versions. The attr()
method is used to access the input and sets its properties.
We must change the checked
property to true
or false
depending on what we want to check or uncheck the checkbox.
When changing the attribute to true
, it is required to provide a click()
method to ensure that the option in the option group is modified.
Syntax:
$("#checkbox_name").attr("checked", true);
Example:
$(document).ready(function() {
$(".clickCheck").click(function() {
$("#chechId").attr("checked", true);
});
$(".clickUncheck").click(function() {
$("#chechId").attr("checked", false);
});
});
The output using the attr()
method is the same as that from the prop()
method.
Output After Running the Code:
Output After Clicking the Yes
Button:
Output After Clicking the No
Button:
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn