How to Select by Data Attribute in jQuery
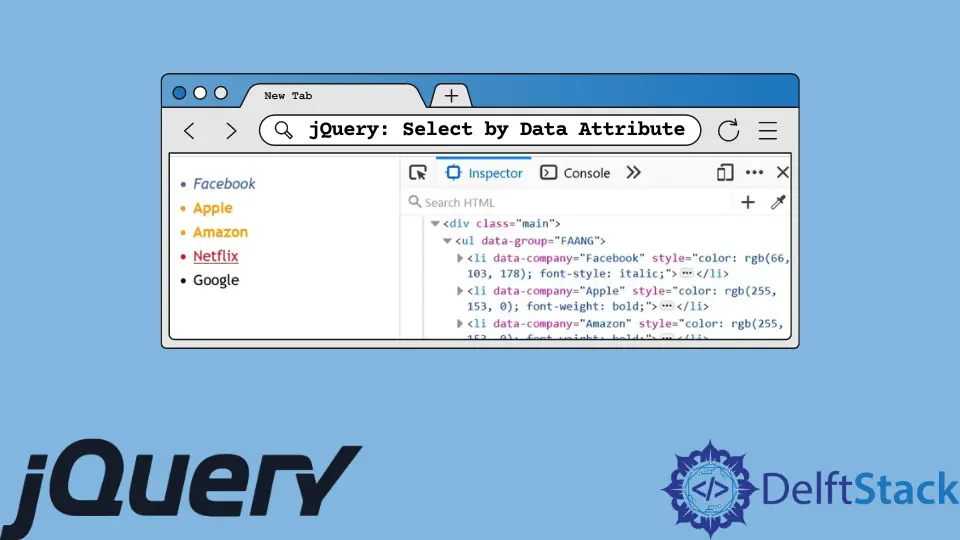
This article teaches two methods to select custom HTML5 data attributes using jQuery. The first method will select the data attributes using their surrounding HTML tags.
For the second method, you’ll use jQuery Selectors defined in the jQuery API documentation.
Select Custom Data Attributes Using HTML Tags
Using their surrounding HTML tags as a proxy, you can select custom data attributes. You can use a typical CSS attribute selector to select the data attributes.
In the following, the HTML part of the code contains an HTML unordered list marked with the <ul>
tag. This list has a data-group
attribute and a value; you’ll use the former and latter in the jQuery code.
Also, the list items have a data-company
attribute and values. You can select these attributes using the HTML tags; that’s what we’ve done in the following code.
<!DOCTYPE html>
<html lang="en">
<head>
<title>01-jQuery-select-data-by-attribute</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
ul {
font: 1.2em/1.618 "Trebuchet MS", Verdana, Helvetica, sans-serif;
}
</style>
</head>
<body>
<div class="main">
<ul data-group="FAANG">
<li data-company="Facebook">Facebook</li>
<li data-company="Apple">Apple</li>
<li data-company="Amazon">Amazon</li>
<li data-company="Netflix">Netflix</li>
<li data-company="Google">Google</li>
</ul>
</div>
<script>
// Select the data-company attribute that belongs
// to Google, and leave out the rest.
$("ul[data-group='FAANG'] li[data-company='Google']").css({
'color': '#db4437',
'font-weight': 'bold'
});
// Select all data-company attributes except that
// of Google. The li:not() selector makes this
// possible.
$("ul[data-group='FAANG'] li:not([data-company='Google'])").css('color', '#1560bd');
</script>
</body>
</html>
Output:
Select Custom Data Attributes Using jQuery Selectors
The jQuery library has selectors that you can use to select custom HTML5 data attributes. These selectors work like pattern matching using Regular Expressions.
As a result, you can select data attributes that have related characteristics. In the following, we show you selectors that match different data attributes.
<!DOCTYPE html>
<html lang="en">
<head>
<title>02-jQuery-select-data-by-attribute</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
ul {
font: 1.2em/1.618 "Trebuchet MS", Verdana, Helvetica, sans-serif;
}
</style>
</head>
<body>
<div class="main">
<ul data-group="FAANG">
<li data-company="Facebook">Facebook</li>
<li data-company="Apple">Apple</li>
<li data-company="Amazon">Amazon</li>
<li data-company="Netflix">Netflix</li>
<li data-company="Google">Google</li>
</ul>
</div>
<script>
//stored selector
let parent_list = $('ul[data-group="FAANG"]');
// Select data-company that starts with "A"
let company_that_start_with_letter_a = $('[data-company^="A"]', parent_list).css({
'color': '#ff9900',
'font-weight': 'bold'
});
// Select data-company that contains "Face"
let company_that_contains_face = $('[data-company*="Face"]', parent_list).css({
'color': '#4267b2',
'font-style': 'italic'
});
// Select data-company that ends with "flix"
let company_that_ends_with_flix = $('[data-company$="flix"]', parent_list).css({
'color': '#e50914',
'text-decoration': 'underline'
});
</script>
</body>
</html>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn