How to Remove an Event Listener in jQuery
-
Use jQuery
off()
API to Remove Event Listener -
Use jQuery
unbind()
API to Remove Event Listener -
Use jQuery
prop()
andattr()
API to Remove Event Listener - Use Element Cloning to Remove Event Listener
-
Use jQuery
undelegate()
API to Remove Event Listener
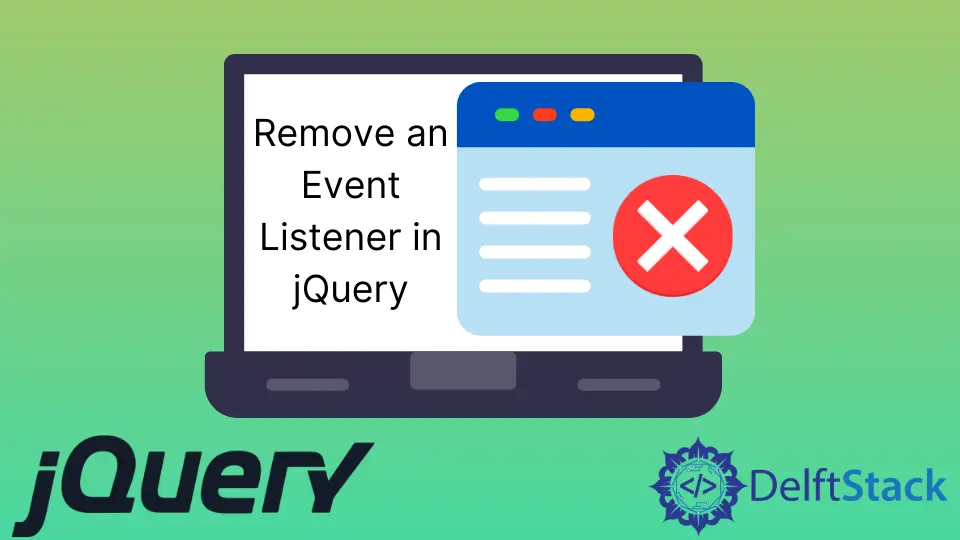
This article teaches you five ways that show you how to remove an event listener in jQuery. Depending on how you added the event listener, you can use any of the methods.
All our examples have code and detailed comments that you can run in your web browser.
Use jQuery off()
API to Remove Event Listener
jQuery off()
API, as the name implies, will remove an event handler from the selected element. The following code has a form input that accepts a number between zero and 10 inclusive.
When a user submits the form, we check if this is true; if not, we remove the button submit event using the off()
API. At the same time, we disable the submit button as a sign that the button no longer works.
Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
input[disabled="disabled"]{
cursor: not-allowed;
}
</style>
</head>
<body>
<main>
<form id="form">
<label for="number">Enter a number between 1 and 10:</label>
<input type="number" name="number" id="input-number" required>
<input type="submit" name="submit-form" id="submit-form">
</form>
</main>
<script>
$(document).ready(function(){
$("#form").submit(function(event) {
// Prevent the form from submitting
event.preventDefault();
// Coerce the form input to a number using the
// Number object. Afterward, we perform a basic
// form validation.
let input_number_value = Number($("#input-number").val());
if (typeof(input_number_value) == 'number' &&
input_number_value >= 0 &&
input_number_value <= 10
) {
console.log($("#input-number").val());
} else {
// If the user enters below or above 10,
// we disable the submit button and remove
// the "submit" event listener.
$("#submit-form").attr("disabled", true);
$("#form").off('submit');
console.log("You entered an invalid number. So we've disabled the submit button.")
}
})
})
</script>
</body>
</html>
Output:
Still, on the off()
API, you can use it to remove a click event from a dynamic element. You’ll create such an element based on user interaction, for example, a click event.
At the same time, you could add a click event to this element using $(document).on()
. Due to this, you can only remove this click event using $(document).off()
.
Code Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01_02-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
main {
width: 50%;
margin: 2em auto;
font-size: 1.2em;
}
main button {
font-size: 1em;
}
.box {
background-color: #1560bd;
width: 100px;
height: 100px;
margin-bottom: 12px;
color: #ffffff;
text-align: center;
padding: 0.2em;
cursor: pointer;
}
[disabled="disabled"]{
cursor: not-allowed;
}
</style>
</head>
<body>
<main>
<div id="div_board">
<p>Click the button to add a div!</p>
</div>
<button id="add_div">Add a Div</button>
</main>
<script>
$(document).ready(function() {
$("#add_div").click(function() {
// Append a new div, then disable the button
// and remove the click event using the ".off()"
// API.
$("#div_board").append("<div class='box'>I am a new DIV. Click me!</div>");
$("#add_div").off('click');
$("#add_div").attr("disabled", true);
});
});
// We attach a dynamic click event to the new div.
// After the user clicks it, we use $(document).off()
// to remove the "click" event. Other techniques like
// "unbind" will not work
$(document).on('click', 'div.box', function() {
alert('You clicked me');
// This will remove the click event because
// we added it using $(document).on
$(document).off('click', 'div.box');
// Tell them why the box can no longer receive a
// click event.
console.log("After clicking the box, we removed the click event. As a result, you can no longer click the box.")
/*This will not work, and the click event will
not get removed
$('div.box').unbind('click');*/
})
</script>
</body>
</html>
Output:
Use jQuery unbind()
API to Remove Event Listener
The unbind()
API will remove all event handlers attached to an element. If you want, you can pass the name of the event you want to remove.
We’ve done that in the following code; we remove a click event using the unbind()
API. This will happen if the user fails a verification implemented in the code.
This verification checks the number of characters for the alternate image text. If this number does not fall between 20 and 50 characters, the code removes the click event using the unbind()
API.
Code Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#test-image {
cursor: pointer;
}
</style>
</head>
<body>
<div>
<!-- You can Download the test image from Unsplash:
https://unsplash.com/photos/lsdA8QpWN_A -->
<img id="test-image" src="test-image.jpg" alt="">
</div>
<script>
$(document).ready(function(){
$("#test-image").click(function() {
let image_alt_text = prompt("Enter between 20 and 50 characters for the alt text", "");
if (image_alt_text !== null) {
if (image_alt_text.length >= 20 && image_alt_text.length <= 50) {
if ($("#test-image").attr("alt") === '') {
$("#test-image").attr("alt", image_alt_text);
} else {
console.log("Image already has an alt text.");
}
return
} else {
// User entered an invalid alt text
// Remove the click event using the unbind
// method.
$("#test-image").unbind("click");
// Tell them why they no longer have access to the
// prompt dialog box.
console.log("The dialog box that allows you to enter an alt text is no longer available because you entered an invalid alt text. Thank you.")
}
}
})
});
</script>
</body>
</html>
Output:
Use jQuery prop()
and attr()
API to Remove Event Listener
If the click event on your element is from the onclick()
attribute, you can remove it using the prop()
and attr()
API. In the following example, the paragraph has an onclick()
event that activates a function.
After the function shows a message, it will remove the click event by setting it to null
.
Code Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>03-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<p id="big-text" style="font-size: 3em;" onclick="say_thank_you()">I am a very big text! Click me!</p>
<script>
function say_thank_you() {
alert("You clicked me! Good bye!");
// Remove the onclick event handler
$("#big-text").prop("onclick", null).attr("onclick", null);
}
</script>
</body>
</html>
Output:
Use Element Cloning to Remove Event Listener
Element cloning using jQuery clone()
API is another method to remove an event listener. To get started, clone the element using the clone()
API; the cloned element does not have the event handler.
Still, it only exists in memory, and the original element with the event handler is still on the page. To fix this, you’ll use the replaceWith()
API to replace the original element with the clone.
Code Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>04-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#click-once {
cursor: pointer;
}
</style>
</head>
<body>
<p id="click-once" style="font-size: 3em;">Click for your one-time welcome message.</p>
<script>
$(document).ready(function() {
$("#click-once").click(function() {
alert("Thanks for your visit!");
// Replace the original paragraph with a
// clone. This clone will not have any event
// handler.
let original_paragraph;
let cloned_paragraph;
original_paragraph = $("#click-once");
cloned_paragraph = original_paragraph.clone();
original_paragraph.replaceWith(cloned_paragraph);
// If the user wants the one-time message, tell
// them how to get it back.
console.log("Your one-time message is no longer available. If you want to see it again, reload the page and click the text on the web page.")
})
});
</script>
</body>
</html>
Output:
Use jQuery undelegate()
API to Remove Event Listener
When you assign a click event to an element using the HTML <body>
as a proxy, you can use the undelegate()
API to remove it. In the following, we use $('body').on('click')
to assign a click event to an element having the .main
class.
As a result, you’ll need the undelegate()
API to remove the click event.
Code Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>05-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
main {
background-color: #1a1a1a;
color: #ffffff;
padding: 2em;
height: 50vh;
}
p {
cursor: pointer;
}
</style>
</head>
<body>
<main class="main">
<p>Hello World, click me!</p>
</main>
<script>
$(document).ready(function() {
$('body').on("click", '.main', function() {
alert("Hello and Good Bye!");
// Remove the click event using the
// undelegate() method.
$('body').undelegate('.main', 'click');
})
});
</script>
</body>
</html>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn