How to Create an On-Page Load Event in jQuery
- Understanding the On-Page Load Event
-
Method 1: Using jQuery’s
$(document).ready()
-
Method 2: Using the Shorter Version of
$(document).ready()
-
Method 3: Using
$(window).on('load', ...)
- Conclusion
- FAQ
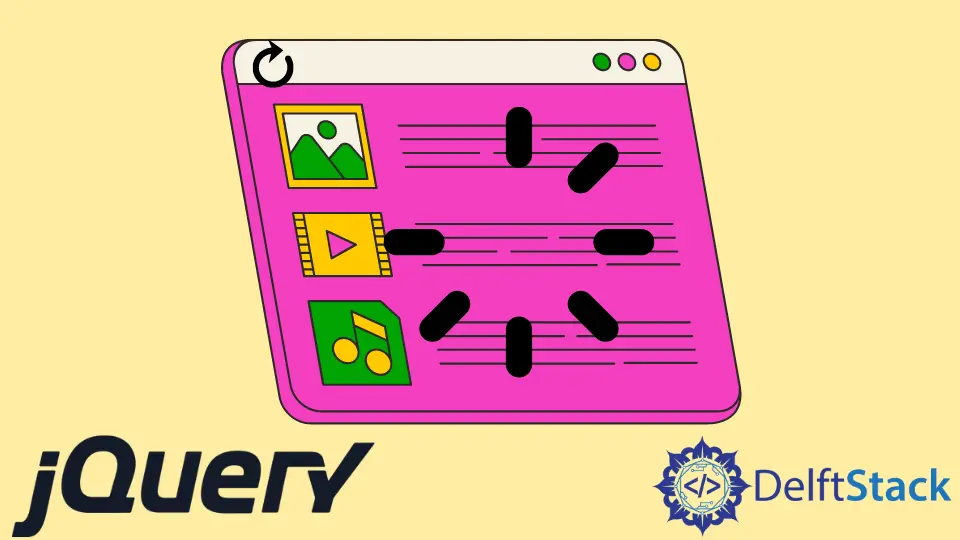
Creating an on-page load event in jQuery is a fundamental skill for web developers looking to enhance user experience. By ensuring that scripts execute only after the entire page is loaded, you can prevent errors and improve performance.
This tutorial will guide you through the process of creating an on-page load event using jQuery, providing clear examples and detailed explanations. Whether you’re a novice or an experienced developer, understanding how to manage load events can significantly impact your web projects. Let’s dive right in!
Understanding the On-Page Load Event
Before we delve into the practical examples, it’s essential to understand what an on-page load event is. In simple terms, this event triggers when the entire content of a webpage, including images, scripts, and stylesheets, has finished loading. This is crucial because it ensures that your jQuery scripts run only after all elements are available in the DOM (Document Object Model).
Using jQuery, you can easily create an on-page load event using the $(document).ready()
function. This function allows you to execute any jQuery code once the DOM is fully loaded, which is a best practice for ensuring that your scripts work correctly without encountering any issues related to elements not being available.
Method 1: Using jQuery’s $(document).ready()
The most common way to create an on-page load event in jQuery is to utilize the $(document).ready()
method. This method ensures that your code runs only after the DOM is completely loaded. Here’s how you can implement it:
$(document).ready(function() {
alert("Page is fully loaded!");
});
Output:
Page is fully loaded!
In this example, as soon as the DOM is ready, an alert box will pop up, indicating that the page has fully loaded. This is a straightforward approach, ideal for beginners and those looking to execute simple scripts or functions right after the page is loaded.
The $(document).ready()
function is vital for preventing errors that may arise if your script tries to access elements that haven’t been loaded yet. It creates a safe environment for running your jQuery code, ensuring that all elements are available for manipulation or interaction.
Method 2: Using the Shorter Version of $(document).ready()
If you prefer a more concise syntax, jQuery allows you to omit the function keyword and parentheses in the $(document).ready()
method. This shorter version achieves the same result and can make your code cleaner and easier to read. Here’s how it looks:
$(function() {
console.log("Page is fully loaded!");
});
Output:
Page is fully loaded!
In this example, instead of an alert, we use console.log()
to output a message to the console. This is particularly useful for debugging or when you want to execute more complex scripts without interrupting the user experience with alerts.
This shorthand is widely used among developers for its simplicity and efficiency. It’s a great option when you want to keep your code minimal while still ensuring that it runs only after the DOM is ready.
Method 3: Using $(window).on('load', ...)
While $(document).ready()
is sufficient for most scenarios, there are instances where you may want to wait until all resources, including images and iframes, are fully loaded. In such cases, you can use the $(window).on('load', ...)
method. Here’s how:
$(window).on('load', function() {
console.log("All resources are fully loaded!");
});
Output:
All resources are fully loaded!
This method is particularly useful for scenarios where your scripts depend on images or other resources being fully loaded. Unlike $(document).ready()
, which only waits for the DOM, $(window).on('load', ...)
ensures that everything is completely loaded before executing the code.
This approach can help you avoid issues that arise from manipulating elements that are not yet fully rendered, particularly in cases where images or other external resources are critical to your layout or functionality.
Conclusion
Creating an on-page load event in jQuery is a simple yet essential skill for web developers. By using methods like $(document).ready()
and $(window).on('load', ...)
, you can ensure that your scripts run at the right time, improving the overall performance and user experience of your website. Whether you’re creating interactive elements or simply logging messages, mastering these techniques will enhance your ability to build dynamic web applications.
Incorporating these practices into your development workflow can save you time and prevent frustrating errors, ultimately leading to smoother and more efficient web projects.
FAQ
-
What is the difference between
$(document).ready()
and$(window).on('load', ...)
?
$(document).ready()
triggers once the DOM is fully loaded, while$(window).on('load', ...)
waits for all resources, including images, to load. -
Can I use jQuery without including it in my project?
No, jQuery must be included in your project via a CDN or downloaded file for the methods to work. -
Is it necessary to use jQuery for handling on-page load events?
No, you can use vanilla JavaScript with theDOMContentLoaded
event, but jQuery simplifies the process.
-
How can I check if jQuery is loaded on my webpage?
You can check by typingjQuery
or$
in the browser console. If it returns a function, jQuery is loaded. -
Are there performance implications of using on-page load events?
Yes, using the right method can impact performance. Use$(document).ready()
for quicker scripts and$(window).on('load', ...)
for resource-heavy scripts.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook