How to Trigger the Same Function From Multiple Events in jQuery
- jQuery Multiple Events: Track User Interaction in HTML Form
- jQuery Multiple Events: Change Background Color
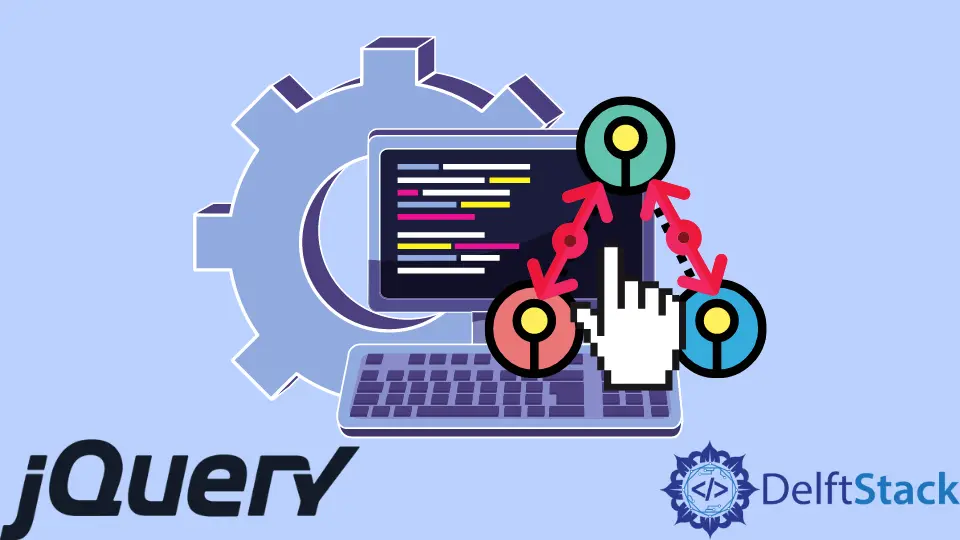
This article teaches you how to trigger the same function from multiple events in jQuery. We’ll show two examples of how to do this using jQuery .on()
API.
The first example tracks user movements in HTML form inputs. While the second will change an HTML element’s background color.
jQuery Multiple Events: Track User Interaction in HTML Form
In this example, we set up an anonymous function that tracks two user interactions. The first is onblur
and the second is onmouseleave
.
We’ll have to write this in two places on a normal day, but we can do it simultaneously with the .on()
API. First, we choose an element for the multiple events.
Then we use .on()
to monitor onblur
and onmouseleave
events.
Doing this can track when a user switches between form inputs. If you want to, you can implement form validation in the process.
In the following, we have an HTML form with two HTML inputs. Then we have the jQuery .on()
API listens for onblur
and onmouseleave
events.
During this process, we log messages to the web browser’s console.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Multiple Events: Basic Form Validation</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
body { display: grid; justify-content: center; align-items: center; height: 100vh;}
form { border: 2px solid #1560bd; font-size: 2em; padding: 1.2em; }
</style>
</head>
<body>
<main>
<form id="html_form">
<label for="first_name">First name</label>
<input id="first_name" type="text" name="first_name">
<label for="last_name">Last name</label>
<input id="last_name" type="text" name="last_name">
<input type="submit" name="submit" value="Submit form">
</form>
</main>
<script>
$("document").ready(function() {
// Use the "on" API to attach anonymous functions
// to the focus, blur, and mouseleave events. Although
// we only show console.log messages; you can implement
// form validation routines.
$("#first_name, #last_name").on({
focus: function() {
$(this).css("outline", "3px solid #1a1a1a");
},
blur: function() {
$(this).removeAttr("style");
console.log("Blur left");
},
mouseleave: function() {
$(this).removeAttr("style");
console.log("Mouse left");
},
})
});
// Prevent form submission
$("#html_form").submit(function(e) {
return false;
})
</script>
</body>
</html>
Output:
jQuery Multiple Events: Change Background Color
You can change an element’s background color based on mouse movements. You can use onmouseenter
and onmouseleave
in Vanilla JavaScript.
But with jQuery .on()
API, you can do it all in one line, but you can break it up for better readability. In the following, when you move your mouse over the main
element, its background color changes.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Multiple Events: Change page background color</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
body { display: grid; justify-content: center; align-items: center; height: 100vh;}
main { font-size: 2em; padding: 1em; border: 5px solid #1a1a1a; display: block;}
</style>
</head>
<body>
<main id="main">
<p>This is a big text. Move your mouse and see what happens.</p>
</main>
<script type="text/javascript">
$("document").ready(function() {
// Change the background color of the main
// element when the user moves their mouse over
// it.
$("#main").on({
mouseenter: function() {
$(this).css("background", "#aaa");
},
mouseleave: function() {
$(this).css("background", "#fafafa");
},
})
});
</script>
</body>
</html>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn