jQuery: 여러 이벤트에서 동일한 기능 트리거
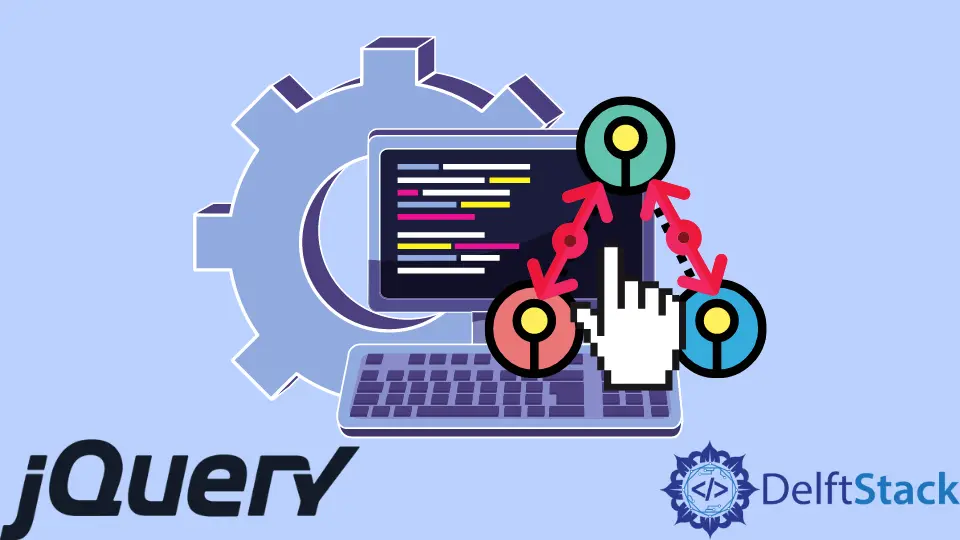
이 기사에서는 jQuery의 여러 이벤트에서 동일한 기능을 트리거하는 방법을 설명합니다. jQuery .on()
API를 사용하여 이를 수행하는 방법에 대한 두 가지 예를 보여줍니다.
첫 번째 예는 HTML 양식 입력에서 사용자 움직임을 추적합니다. 두 번째는 HTML 요소의 배경색을 변경합니다.
jQuery 다중 이벤트: HTML 양식에서 사용자 상호 작용 추적
이 예에서는 두 가지 사용자 상호 작용을 추적하는 익명 함수를 설정합니다. 첫 번째는 onblur
이고 두 번째는 onmouseleave
입니다.
평상시에는 두 곳에 작성해야 하지만 .on()
API를 사용하면 동시에 할 수 있습니다. 먼저 여러 이벤트에 대한 요소를 선택합니다.
그런 다음 .on()
을 사용하여 onblur
및 onmouseleave
이벤트를 모니터링합니다.
이렇게 하면 사용자가 양식 입력 사이를 전환할 때 추적할 수 있습니다. 원하는 경우 프로세스에서 양식 유효성 검사를 구현할 수 있습니다.
다음에는 두 개의 HTML 입력이 있는 HTML 양식이 있습니다. 그런 다음 jQuery .on()
API가 onblur
및 onmouseleave
이벤트를 수신하도록 합니다.
이 과정에서 웹 브라우저의 콘솔에 메시지를 기록합니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Multiple Events: Basic Form Validation</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
body { display: grid; justify-content: center; align-items: center; height: 100vh;}
form { border: 2px solid #1560bd; font-size: 2em; padding: 1.2em; }
</style>
</head>
<body>
<main>
<form id="html_form">
<label for="first_name">First name</label>
<input id="first_name" type="text" name="first_name">
<label for="last_name">Last name</label>
<input id="last_name" type="text" name="last_name">
<input type="submit" name="submit" value="Submit form">
</form>
</main>
<script>
$("document").ready(function() {
// Use the "on" API to attach anonymous functions
// to the focus, blur, and mouseleave events. Although
// we only show console.log messages; you can implement
// form validation routines.
$("#first_name, #last_name").on({
focus: function() {
$(this).css("outline", "3px solid #1a1a1a");
},
blur: function() {
$(this).removeAttr("style");
console.log("Blur left");
},
mouseleave: function() {
$(this).removeAttr("style");
console.log("Mouse left");
},
})
});
// Prevent form submission
$("#html_form").submit(function(e) {
return false;
})
</script>
</body>
</html>
출력:
jQuery 다중 이벤트: 배경색 변경
마우스 움직임에 따라 요소의 배경색을 변경할 수 있습니다. Vanilla JavaScript에서 onmouseenter
및 onmouseleave
를 사용할 수 있습니다.
그러나 jQuery .on()
API를 사용하면 한 줄에 모든 작업을 수행할 수 있지만 더 나은 가독성을 위해 분할할 수 있습니다. 다음에서 main
요소 위로 마우스를 이동하면 배경색이 변경됩니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Multiple Events: Change page background color</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
body { display: grid; justify-content: center; align-items: center; height: 100vh;}
main { font-size: 2em; padding: 1em; border: 5px solid #1a1a1a; display: block;}
</style>
</head>
<body>
<main id="main">
<p>This is a big text. Move your mouse and see what happens.</p>
</main>
<script type="text/javascript">
$("document").ready(function() {
// Change the background color of the main
// element when the user moves their mouse over
// it.
$("#main").on({
mouseenter: function() {
$(this).css("background", "#aaa");
},
mouseleave: function() {
$(this).css("background", "#fafafa");
},
})
});
</script>
</body>
</html>
출력:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn