jQuery에서 이벤트 리스너를 제거하는 5가지 방법
-
jQuery
off()
API를 사용하여 이벤트 리스너 제거 -
jQuery
unbind()
API를 사용하여 이벤트 리스너 제거 -
jQuery
prop()
및attr()
API를 사용하여 이벤트 리스너 제거 - 요소 복제를 사용하여 이벤트 리스너 제거
-
jQuery
undelegate()
API를 사용하여 이벤트 리스너 제거
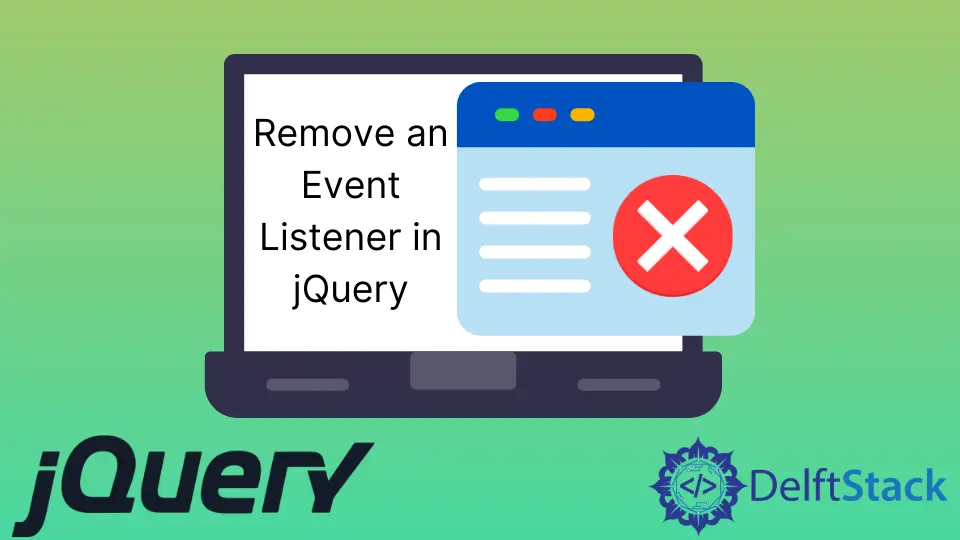
이 기사에서는 jQuery에서 이벤트 리스너를 제거하는 방법을 보여주는 다섯 가지 방법을 설명합니다. 이벤트 리스너를 추가한 방법에 따라 모든 방법을 사용할 수 있습니다.
모든 예제에는 웹 브라우저에서 실행할 수 있는 코드와 자세한 설명이 있습니다.
jQuery off()
API를 사용하여 이벤트 리스너 제거
이름에서 알 수 있듯이 jQuery off()
API는 선택한 요소에서 이벤트 핸들러를 제거합니다. 다음 코드에는 0에서 10 사이의 숫자를 허용하는 양식 입력이 있습니다.
사용자가 양식을 제출하면 이것이 사실인지 확인합니다. 그렇지 않은 경우 off()
API를 사용하여 버튼 제출 이벤트를 제거합니다. 동시에 버튼이 더 이상 작동하지 않는다는 표시로 제출 버튼을 비활성화합니다.
암호:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
input[disabled="disabled"]{
cursor: not-allowed;
}
</style>
</head>
<body>
<main>
<form id="form">
<label for="number">Enter a number between 1 and 10:</label>
<input type="number" name="number" id="input-number" required>
<input type="submit" name="submit-form" id="submit-form">
</form>
</main>
<script>
$(document).ready(function(){
$("#form").submit(function(event) {
// Prevent the form from submitting
event.preventDefault();
// Coerce the form input to a number using the
// Number object. Afterward, we perform a basic
// form validation.
let input_number_value = Number($("#input-number").val());
if (typeof(input_number_value) == 'number' &&
input_number_value >= 0 &&
input_number_value <= 10
) {
console.log($("#input-number").val());
} else {
// If the user enters below or above 10,
// we disable the submit button and remove
// the "submit" event listener.
$("#submit-form").attr("disabled", true);
$("#form").off('submit');
console.log("You entered an invalid number. So we've disabled the submit button.")
}
})
})
</script>
</body>
</html>
출력:
여전히 off()
API에서 이를 사용하여 동적 요소에서 클릭 이벤트를 제거할 수 있습니다. 예를 들어 클릭 이벤트와 같은 사용자 상호 작용을 기반으로 이러한 요소를 만듭니다.
동시에 $(document).on()
을 사용하여 이 요소에 클릭 이벤트를 추가할 수 있습니다. 이로 인해 이 클릭 이벤트는 $(document).off()
를 통해서만 제거할 수 있습니다.
코드 예:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01_02-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
main {
width: 50%;
margin: 2em auto;
font-size: 1.2em;
}
main button {
font-size: 1em;
}
.box {
background-color: #1560bd;
width: 100px;
height: 100px;
margin-bottom: 12px;
color: #ffffff;
text-align: center;
padding: 0.2em;
cursor: pointer;
}
[disabled="disabled"]{
cursor: not-allowed;
}
</style>
</head>
<body>
<main>
<div id="div_board">
<p>Click the button to add a div!</p>
</div>
<button id="add_div">Add a Div</button>
</main>
<script>
$(document).ready(function() {
$("#add_div").click(function() {
// Append a new div, then disable the button
// and remove the click event using the ".off()"
// API.
$("#div_board").append("<div class='box'>I am a new DIV. Click me!</div>");
$("#add_div").off('click');
$("#add_div").attr("disabled", true);
});
});
// We attach a dynamic click event to the new div.
// After the user clicks it, we use $(document).off()
// to remove the "click" event. Other techniques like
// "unbind" will not work
$(document).on('click', 'div.box', function() {
alert('You clicked me');
// This will remove the click event because
// we added it using $(document).on
$(document).off('click', 'div.box');
// Tell them why the box can no longer receive a
// click event.
console.log("After clicking the box, we removed the click event. As a result, you can no longer click the box.")
/*This will not work, and the click event will
not get removed
$('div.box').unbind('click');*/
})
</script>
</body>
</html>
출력:
jQuery unbind()
API를 사용하여 이벤트 리스너 제거
unbind()
API는 요소에 연결된 모든 이벤트 핸들러를 제거합니다. 원하는 경우 제거하려는 이벤트의 이름을 전달할 수 있습니다.
다음 코드에서 이를 수행했습니다. unbind()
API를 사용하여 클릭 이벤트를 제거합니다. 사용자가 코드에 구현된 확인에 실패하면 이런 일이 발생합니다.
이 확인은 대체 이미지 텍스트의 문자 수를 확인합니다. 이 숫자가 20~50자 사이에 있지 않으면 코드는 unbind()
API를 사용하여 클릭 이벤트를 제거합니다.
코드 예:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#test-image {
cursor: pointer;
}
</style>
</head>
<body>
<div>
<!-- You can Download the test image from Unsplash:
https://unsplash.com/photos/lsdA8QpWN_A -->
<img id="test-image" src="test-image.jpg" alt="">
</div>
<script>
$(document).ready(function(){
$("#test-image").click(function() {
let image_alt_text = prompt("Enter between 20 and 50 characters for the alt text", "");
if (image_alt_text !== null) {
if (image_alt_text.length >= 20 && image_alt_text.length <= 50) {
if ($("#test-image").attr("alt") === '') {
$("#test-image").attr("alt", image_alt_text);
} else {
console.log("Image already has an alt text.");
}
return
} else {
// User entered an invalid alt text
// Remove the click event using the unbind
// method.
$("#test-image").unbind("click");
// Tell them why they no longer have access to the
// prompt dialog box.
console.log("The dialog box that allows you to enter an alt text is no longer available because you entered an invalid alt text. Thank you.")
}
}
})
});
</script>
</body>
</html>
출력:
jQuery prop()
및 attr()
API를 사용하여 이벤트 리스너 제거
요소의 클릭 이벤트가 onclick()
속성에서 발생한 경우 prop()
및 attr()
API를 사용하여 제거할 수 있습니다. 다음 예제에서 단락에는 함수를 활성화하는 onclick()
이벤트가 있습니다.
함수가 메시지를 표시한 후 null
로 설정하여 클릭 이벤트를 제거합니다.
코드 예:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>03-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<p id="big-text" style="font-size: 3em;" onclick="say_thank_you()">I am a very big text! Click me!</p>
<script>
function say_thank_you() {
alert("You clicked me! Good bye!");
// Remove the onclick event handler
$("#big-text").prop("onclick", null).attr("onclick", null);
}
</script>
</body>
</html>
출력:
요소 복제를 사용하여 이벤트 리스너 제거
jQuery clone()
API를 사용한 요소 복제는 이벤트 리스너를 제거하는 또 다른 방법입니다. 시작하려면 clone()
API를 사용하여 요소를 복제하십시오. 복제된 요소에는 이벤트 핸들러가 없습니다.
여전히 메모리에만 존재하며 이벤트 핸들러가 있는 원래 요소는 여전히 페이지에 있습니다. 이 문제를 해결하려면 replaceWith()
API를 사용하여 원본 요소를 복제본으로 교체합니다.
코드 예:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>04-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#click-once {
cursor: pointer;
}
</style>
</head>
<body>
<p id="click-once" style="font-size: 3em;">Click for your one-time welcome message.</p>
<script>
$(document).ready(function() {
$("#click-once").click(function() {
alert("Thanks for your visit!");
// Replace the original paragraph with a
// clone. This clone will not have any event
// handler.
let original_paragraph;
let cloned_paragraph;
original_paragraph = $("#click-once");
cloned_paragraph = original_paragraph.clone();
original_paragraph.replaceWith(cloned_paragraph);
// If the user wants the one-time message, tell
// them how to get it back.
console.log("Your one-time message is no longer available. If you want to see it again, reload the page and click the text on the web page.")
})
});
</script>
</body>
</html>
출력:
jQuery undelegate()
API를 사용하여 이벤트 리스너 제거
HTML <body>
를 프록시로 사용하여 요소에 클릭 이벤트를 할당할 때 undelegate()
API를 사용하여 제거할 수 있습니다. 다음에서는 $('body').on('click')
을 사용하여 .main
클래스가 있는 요소에 클릭 이벤트를 할당합니다.
따라서 클릭 이벤트를 제거하려면 undelegate()
API가 필요합니다.
코드 예:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>05-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
main {
background-color: #1a1a1a;
color: #ffffff;
padding: 2em;
height: 50vh;
}
p {
cursor: pointer;
}
</style>
</head>
<body>
<main class="main">
<p>Hello World, click me!</p>
</main>
<script>
$(document).ready(function() {
$('body').on("click", '.main', function() {
alert("Hello and Good Bye!");
// Remove the click event using the
// undelegate() method.
$('body').undelegate('.main', 'click');
})
});
</script>
</body>
</html>
출력:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn