jQuery でイベント リスナーを削除する 5つの方法
-
jQuery
off()
API を使用してイベント リスナーを削除する -
jQuery
unbind()
API を使用してイベント リスナーを削除する -
jQuery
prop()
およびattr()
API を使用してイベント リスナーを削除する - 要素の複製を使用してイベント リスナーを削除する
-
jQuery
undelegate()
API を使用してイベント リスナーを削除する
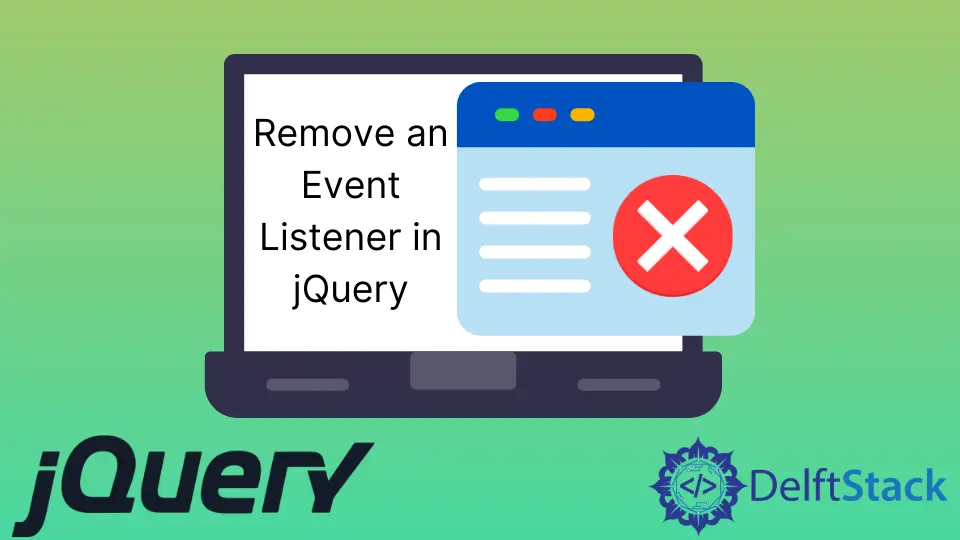
この記事では、jQuery でイベント リスナーを削除する方法を示す 5つの方法を説明します。 イベント リスナーを追加した方法に応じて、任意のメソッドを使用できます。
すべての例には、Web ブラウザーで実行できるコードと詳細なコメントが含まれています。
jQuery off()
API を使用してイベント リスナーを削除する
jQuery off()
API は、名前が示すように、選択した要素からイベント ハンドラーを削除します。 次のコードには、0 から 10 までの数値を受け入れるフォーム入力があります。
ユーザーがフォームを送信すると、これが true かどうかを確認します。 そうでない場合は、off()
API を使用してボタン送信イベントを削除します。 同時に、ボタンが機能しなくなったことを示す兆候として、送信ボタンを無効にします。
コード:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
input[disabled="disabled"]{
cursor: not-allowed;
}
</style>
</head>
<body>
<main>
<form id="form">
<label for="number">Enter a number between 1 and 10:</label>
<input type="number" name="number" id="input-number" required>
<input type="submit" name="submit-form" id="submit-form">
</form>
</main>
<script>
$(document).ready(function(){
$("#form").submit(function(event) {
// Prevent the form from submitting
event.preventDefault();
// Coerce the form input to a number using the
// Number object. Afterward, we perform a basic
// form validation.
let input_number_value = Number($("#input-number").val());
if (typeof(input_number_value) == 'number' &&
input_number_value >= 0 &&
input_number_value <= 10
) {
console.log($("#input-number").val());
} else {
// If the user enters below or above 10,
// we disable the submit button and remove
// the "submit" event listener.
$("#submit-form").attr("disabled", true);
$("#form").off('submit');
console.log("You entered an invalid number. So we've disabled the submit button.")
}
})
})
</script>
</body>
</html>
出力:
それでも、off()
API では、これを使用して動的要素からクリック イベントを削除できます。 このような要素は、クリック イベントなどのユーザー インタラクションに基づいて作成します。
同時に、$(document).on()
を使用して、この要素にクリック イベントを追加できます。 このため、このクリック イベントは $(document).off()
を使用してのみ削除できます。
コード例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01_02-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
main {
width: 50%;
margin: 2em auto;
font-size: 1.2em;
}
main button {
font-size: 1em;
}
.box {
background-color: #1560bd;
width: 100px;
height: 100px;
margin-bottom: 12px;
color: #ffffff;
text-align: center;
padding: 0.2em;
cursor: pointer;
}
[disabled="disabled"]{
cursor: not-allowed;
}
</style>
</head>
<body>
<main>
<div id="div_board">
<p>Click the button to add a div!</p>
</div>
<button id="add_div">Add a Div</button>
</main>
<script>
$(document).ready(function() {
$("#add_div").click(function() {
// Append a new div, then disable the button
// and remove the click event using the ".off()"
// API.
$("#div_board").append("<div class='box'>I am a new DIV. Click me!</div>");
$("#add_div").off('click');
$("#add_div").attr("disabled", true);
});
});
// We attach a dynamic click event to the new div.
// After the user clicks it, we use $(document).off()
// to remove the "click" event. Other techniques like
// "unbind" will not work
$(document).on('click', 'div.box', function() {
alert('You clicked me');
// This will remove the click event because
// we added it using $(document).on
$(document).off('click', 'div.box');
// Tell them why the box can no longer receive a
// click event.
console.log("After clicking the box, we removed the click event. As a result, you can no longer click the box.")
/*This will not work, and the click event will
not get removed
$('div.box').unbind('click');*/
})
</script>
</body>
</html>
出力:
jQuery unbind()
API を使用してイベント リスナーを削除する
unbind()
API は、要素に関連付けられたすべてのイベント ハンドラーを削除します。 必要に応じて、削除するイベントの名前を渡すことができます。
次のコードでそれを行いました。 unbind()
API を使用してクリック イベントを削除します。 これは、ユーザーがコードに実装された検証に失敗した場合に発生します。
この検証では、代替画像テキストの文字数をチェックします。 この数が 20 ~ 50 文字に収まらない場合、コードは unbind()
API を使用してクリック イベントを削除します。
コード例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#test-image {
cursor: pointer;
}
</style>
</head>
<body>
<div>
<!-- You can Download the test image from Unsplash:
https://unsplash.com/photos/lsdA8QpWN_A -->
<img id="test-image" src="test-image.jpg" alt="">
</div>
<script>
$(document).ready(function(){
$("#test-image").click(function() {
let image_alt_text = prompt("Enter between 20 and 50 characters for the alt text", "");
if (image_alt_text !== null) {
if (image_alt_text.length >= 20 && image_alt_text.length <= 50) {
if ($("#test-image").attr("alt") === '') {
$("#test-image").attr("alt", image_alt_text);
} else {
console.log("Image already has an alt text.");
}
return
} else {
// User entered an invalid alt text
// Remove the click event using the unbind
// method.
$("#test-image").unbind("click");
// Tell them why they no longer have access to the
// prompt dialog box.
console.log("The dialog box that allows you to enter an alt text is no longer available because you entered an invalid alt text. Thank you.")
}
}
})
});
</script>
</body>
</html>
出力:
jQuery prop()
および attr()
API を使用してイベント リスナーを削除する
要素のクリック イベントが onclick()
属性からのものである場合、prop()
および attr()
API を使用して削除できます。 次の例では、段落に関数をアクティブにする onclick()
イベントがあります。
関数がメッセージを表示した後、クリック イベントを null
に設定して削除します。
コード例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>03-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<p id="big-text" style="font-size: 3em;" onclick="say_thank_you()">I am a very big text! Click me!</p>
<script>
function say_thank_you() {
alert("You clicked me! Good bye!");
// Remove the onclick event handler
$("#big-text").prop("onclick", null).attr("onclick", null);
}
</script>
</body>
</html>
出力:
要素の複製を使用してイベント リスナーを削除する
jQuery clone()
API を使用した要素の複製は、イベント リスナーを削除する別の方法です。 開始するには、clone()
API を使用して要素を複製します。 複製された要素にはイベント ハンドラがありません。
それでも、それはメモリ内にのみ存在し、イベント ハンドラーを持つ元の要素はまだページ上にあります。 これを修正するには、replaceWith()
API を使用して元の要素をクローンに置き換えます。
コード例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>04-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#click-once {
cursor: pointer;
}
</style>
</head>
<body>
<p id="click-once" style="font-size: 3em;">Click for your one-time welcome message.</p>
<script>
$(document).ready(function() {
$("#click-once").click(function() {
alert("Thanks for your visit!");
// Replace the original paragraph with a
// clone. This clone will not have any event
// handler.
let original_paragraph;
let cloned_paragraph;
original_paragraph = $("#click-once");
cloned_paragraph = original_paragraph.clone();
original_paragraph.replaceWith(cloned_paragraph);
// If the user wants the one-time message, tell
// them how to get it back.
console.log("Your one-time message is no longer available. If you want to see it again, reload the page and click the text on the web page.")
})
});
</script>
</body>
</html>
出力:
jQuery undelegate()
API を使用してイベント リスナーを削除する
HTML <body>
をプロキシとして使用して要素にクリック イベントを割り当てる場合、undelegate()
API を使用してそれを削除できます。 以下では、$('body').on('click')
を使用して、.main
クラスを持つ要素にクリック イベントを割り当てます。
その結果、クリック イベントを削除するには undelegate()
API が必要になります。
コード例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>05-jQuery-remove-event-listener</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
main {
background-color: #1a1a1a;
color: #ffffff;
padding: 2em;
height: 50vh;
}
p {
cursor: pointer;
}
</style>
</head>
<body>
<main class="main">
<p>Hello World, click me!</p>
</main>
<script>
$(document).ready(function() {
$('body').on("click", '.main', function() {
alert("Hello and Good Bye!");
// Remove the click event using the
// undelegate() method.
$('body').undelegate('.main', 'click');
})
});
</script>
</body>
</html>
出力:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn